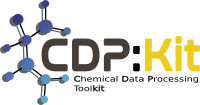 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_REACTIONSUBSTRUCTURESEARCH_HPP
30 #define CDPL_CHEM_REACTIONSUBSTRUCTURESEARCH_HPP
38 #include <boost/iterator/indirect_iterator.hpp>
64 typedef std::vector<AtomBondMapping*> ABMappingList;
70 typedef boost::indirect_iterator<ABMappingList::iterator, AtomBondMapping>
MappingIterator;
75 typedef boost::indirect_iterator<ABMappingList::const_iterator, const AtomBondMapping>
ConstMappingIterator;
262 typedef std::pair<std::size_t, std::size_t> IndexOffsetPair;
270 void initQueryData();
271 void initTargetData();
273 bool findEquivAtoms();
274 bool findEquivBonds();
278 std::size_t nextQueryAtom()
const;
279 bool nextTargetAtom(std::size_t,
unsigned int, std::size_t&, std::size_t&)
const;
281 bool atomMappingAllowed(std::size_t, std::size_t)
const;
283 bool mapBonds(
const Atom*,
const Atom*,
const IndexOffsetPair&);
284 bool mapAtoms(std::size_t);
285 bool mapAtoms(std::size_t, std::size_t);
289 bool hasPostMappingMatchExprs()
const;
292 bool foundMappingUnique();
294 void freeAtomBondMappings();
295 void freeAtomBondMapping();
303 void initAtomMask(std::size_t);
304 void initBondMask(std::size_t);
306 void setAtomBit(std::size_t);
307 void resetAtomBit(std::size_t);
309 bool testAtomBit(std::size_t)
const;
311 void setBondBit(std::size_t);
312 void resetBondMask();
314 bool operator<(
const ABMappingMask&)
const;
315 bool operator>(
const ABMappingMask&)
const;
324 typedef std::vector<Util::BitSet> BitMatrix;
325 typedef std::vector<const Atom*> AtomMappingTable;
326 typedef std::vector<std::size_t> BondMappingTable;
327 typedef std::deque<std::size_t> AtomQueue;
328 typedef std::set<ABMappingMask> UniqueMappingList;
329 typedef std::vector<const Atom*> AtomList;
330 typedef std::vector<const Bond*> BondList;
331 typedef std::vector<std::size_t> IndexList;
332 typedef std::vector<MatchExpression<Atom, MolecularGraph>::SharedPointer> AtomMatchExprTable;
333 typedef std::vector<MatchExpression<Bond, MolecularGraph>::SharedPointer> BondMatchExprTable;
334 typedef std::vector<IndexOffsetPair> IndexOffsetTable;
341 AtomList targetAtoms;
342 BondList targetBonds;
343 IndexOffsetTable queryABIndexOffsets;
344 IndexOffsetTable targetABIndexOffsets;
345 BitMatrix atomEquivMatrix;
346 BitMatrix bondEquivMatrix;
347 AtomQueue termQueryAtoms;
348 AtomMappingTable queryAtomMapping;
349 BondMappingTable queryBondMapping;
351 ABMappingMask targetMappingMask;
352 ABMappingList foundMappings;
353 UniqueMappingList uniqueMappings;
354 AtomMatchExprTable atomMatchExprTable;
355 BondMatchExprTable bondMatchExprTable;
356 ReactionMatchExprPtr rxnMatchExpression;
357 IndexList postMappingMatchAtoms;
358 IndexList postMappingMatchBonds;
359 MappingCache mappingCache;
361 bool initQueryMappingData;
364 unsigned int enabledRxnRoles;
365 std::size_t numQueryAtoms;
366 std::size_t numQueryBonds;
367 std::size_t numTargetAtoms;
368 std::size_t numTargetBonds;
369 std::size_t maxNumMappings;
374 #endif // CDPL_CHEM_REACTIONSUBSTRUCTURESEARCH_HPP
MappingIterator begin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
Definition of the class CDPL::Util::ObjectStack.
void setQuery(const Reaction &query)
Allows to specify a new query reaction pattern.
~ReactionSubstructureSearch()
Destructor.
Definition of the preprocessor macro CDPL_CHEM_API.
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: ReactionSubstructureSearch.hpp:70
std::size_t getNumMappings() const
Returns the number of atom/bond mappings that were recorded in the last call to findMappings().
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
bool uniqueMappingsOnly() const
Tells whether duplicate atom/bond mappings are discarded.
ConstMappingIterator begin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
ConstMappingIterator end() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
ReactionSubstructureSearch()
Constructs and initializes a ReactionSubstructureSearch instance.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
std::size_t getMaxNumMappings() const
Returns the specified limit on the number of stored atom/bond mappings.
Atom.
Definition: Atom.hpp:52
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: ReactionSubstructureSearch.hpp:75
ReactionSubstructureSearch(const Reaction &query)
Constructs and initializes a ReactionSubstructureSearch instance for the specified query reaction pat...
ReactionSubstructureSearch.
Definition: ReactionSubstructureSearch.hpp:62
Definition of the type CDPL::Util::BitSet.
unsigned int getEnabledReactionRoles() const
Tells which reaction component roles are considered in the search for matching reaction substructures...
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
MappingIterator end()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
void uniqueMappingsOnly(bool unique)
Allows to specify whether or not to store only unique atom/bond mappings.
ConstMappingIterator getMappingsBegin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
MappingIterator getMappingsEnd()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
Definition of the class CDPL::Chem::AtomBondMapping.
bool findMappings(const Reaction &target)
Searches for all possible atom/bond between the query reaction pattern and the specified target react...
MappingIterator getMappingsBegin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
void setEnabledReactionRoles(unsigned int roles)
Allows the reaction role specific exclusion of query and target components from the search for matchi...
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
Reaction.
Definition: Reaction.hpp:52
bool mappingExists(const Reaction &target)
Tells whether the query reaction pattern matches the specified target reaction.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
AtomBondMapping & getMapping(std::size_t idx)
Returns a non-const reference to the stored atom/bond mapping object at index idx.
const AtomBondMapping & getMapping(std::size_t idx) const
Returns a const reference to the stored atom/bond mapping object at index idx.
void setMaxNumMappings(std::size_t max_num_mappings)
Allows to specify a limit on the number of stored atom/bond mappings.
ConstMappingIterator getMappingsEnd() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.