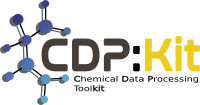 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CONFGEN_RMSDCONFORMERSELECTOR_HPP
30 #define CDPL_CONFGEN_RMSDCONFORMERSELECTOR_HPP
34 #include <unordered_map>
58 static constexpr std::size_t DEF_MAX_NUM_SYMMETRY_MAPPINGS = 32768;
84 typedef std::vector<std::size_t> IndexArray;
86 typedef VectorArrayCache::SharedObjectPointer VectorArrayPtr;
92 void buildSymMappingSearchMolGraph(
const Util::BitSet& atom_mask);
93 void setupSymMappingValidationData(
const Util::BitSet& stable_config_atom_mask,
100 VectorArrayPtr buildCoordsArrayForMapping(
const IndexArray& mapping,
103 typedef std::unordered_map<const Chem::Atom*, Chem::StereoDescriptor> AtomStereoDescriptorMap;
104 typedef std::vector<const Chem::Atom*> AtomList;
106 typedef std::vector<IndexArray*> IndexArrayList;
107 typedef std::vector<VectorArrayPtr> VectorArrayList;
115 IndexArrayList symMappings;
116 AtomStereoDescriptorMap atomStereoDescrs;
118 VectorArrayList confAlignCoords;
119 VectorArrayList selectedConfAlignCoords;
120 AtomList atomNeighbors;
122 std::size_t maxNumSymMappings;
128 #endif // CDPL_CONFGEN_RMSDCONFORMERSELECTOR_HPP
Definition of the class CDPL::Util::ObjectStack.
Definition of the class CDPL::Util::ObjectPool.
Implementation of the Kabsch algorithm.
Definition of the class CDPL::Math::VectorArray.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Fragment.
Definition: Fragment.hpp:52
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Definition of the preprocessor macro CDPL_CONFGEN_API.
Definition of the class CDPL::Chem::AutomorphismGroupSearch.
Definition of the class CDPL::Chem::Fragment.
#define CDPL_CONFGEN_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
The namespace of the Chemical Data Processing Library.
Definition of the type CDPL::Chem::StereoDescriptor.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
AutomorphismGroupSearch.
Definition: AutomorphismGroupSearch.hpp:52
std::function< bool()> CallbackFunction
A generic wrapper class used to store a user-defined callback functions (see [FUNWRP]).
Definition: CallbackFunction.hpp:44
Type definition of a generic wrapper class for storing user-defined callback functions.