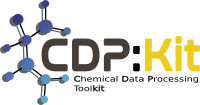 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_DESCR_RDFCODECALCULATOR_HPP
30 #define CDPL_DESCR_RDFCODECALCULATOR_HPP
193 template <
typename Iter,
typename Vec>
197 template <
typename Iter>
198 void init(Iter beg, Iter end);
200 double smoothingFactor;
201 double scalingFactor;
203 double radiusIncrement;
204 std::size_t numSteps;
205 std::size_t numEntities;
208 bool distToIntervalCenterRounding;
218 template <
typename T>
220 smoothingFactor(1.0), scalingFactor(1.0), startRadius(0.0), radiusIncrement(0.1), numSteps(99),
221 distToIntervalCenterRounding(false)
224 template <
typename T>
227 smoothingFactor = factor;
230 template <
typename T>
233 scalingFactor = factor;
236 template <
typename T>
239 startRadius = start_radius;
242 template <
typename T>
245 radiusIncrement = radius_inc;
248 template <
typename T>
251 numSteps = num_steps;
254 template <
typename T>
257 return smoothingFactor;
260 template <
typename T>
263 return scalingFactor;
266 template <
typename T>
272 template <
typename T>
275 return radiusIncrement;
278 template <
typename T>
284 template <
typename T>
290 template <
typename T>
296 template <
typename T>
299 distToIntervalCenterRounding = enable;
302 template <
typename T>
305 return distToIntervalCenterRounding;
308 template <
typename T>
309 template <
typename Iter,
typename Vec>
314 double r = startRadius;
316 for (std::size_t i = 0; i <= numSteps; i++,
r += radiusIncrement) {
319 for (std::size_t j = 0; j < numEntities; j++) {
320 for (std::size_t k = j + 1; k < numEntities; k++) {
321 double t =
r - distMatrix(j, k);
323 sum += weightMatrix(j, k) * std::exp(-smoothingFactor * t * t);
327 rdf_code[i] = scalingFactor *
sum;
331 template <
typename T>
332 template <
typename Iter>
335 numEntities = std::distance(beg, end);
337 weightMatrix.resize(numEntities, numEntities,
false);
338 distMatrix.resize(numEntities, numEntities,
false);
342 for (std::size_t i = 0; beg != end; i++) {
343 const EntityType& entity1 = *beg;
346 entity1_pos = coordsFunc(entity1);
348 std::size_t j = i + 1;
350 for (Iter it = ++beg; it != end; ++it, j++) {
351 const EntityType& entity2 = *it;
354 weightMatrix(i, j) = 1.0;
356 weightMatrix(i, j) = weightFunc(entity1, entity2);
359 double dist =
length(entity1_pos - coordsFunc(entity2));
361 if (distToIntervalCenterRounding)
362 dist = std::round((dist - startRadius) / radiusIncrement) * radiusIncrement + startRadius;
364 distMatrix(i, j) = dist;
367 distMatrix(i, j) = 0.0;
372 #endif // CDPL_DESCR_RDFCODECALCULATOR_HPP
std::function< const Math::Vector3D &(const EntityType &)> Entity3DCoordinatesFunction
Type of the generic functor class used to store a user-defined entity 3D coordinates function.
Definition: RDFCodeCalculator.hpp:74
void setScalingFactor(double factor)
Allows to specify the scaling factor for the RDF code elements.
Definition: RDFCodeCalculator.hpp:231
double getScalingFactor() const
Returns the scaling factor applied to the RDF code elements.
Definition: RDFCodeCalculator.hpp:261
std::size_t getNumSteps() const
Returns the number of performed radius incrementation steps.
Definition: RDFCodeCalculator.hpp:279
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
void setRadiusIncrement(double radius_inc)
Sets the radius step size between successive RDF code elements.
Definition: RDFCodeCalculator.hpp:243
std::function< double(const EntityType &, const EntityType &)> EntityPairWeightFunction
Type of the generic functor class used to store a user-defined entity pair weight function.
Definition: RDFCodeCalculator.hpp:65
double getStartRadius() const
Returns the starting value of the radius.
Definition: RDFCodeCalculator.hpp:267
Atom.
Definition: Atom.hpp:52
double getRadiusIncrement() const
Returns the radius step size between successive RDF code elements.
Definition: RDFCodeCalculator.hpp:273
void setStartRadius(double start_radius)
Sets the starting value of the radius.
Definition: RDFCodeCalculator.hpp:237
RDFCodeCalculator()
Constructs the RDFCodeCalculator instance.
Definition: RDFCodeCalculator.hpp:219
void setSmoothingFactor(double factor)
Allows to specify the smoothing factor used in the calculation of entity pair RDF contributions.
Definition: RDFCodeCalculator.hpp:225
void calculate(Iter beg, Iter end, Vec &rdf_code)
Calculates the RDF code of an entity sequence.
Definition: RDFCodeCalculator.hpp:310
GridElementSum< E >::ResultType sum(const GridExpression< E > &e)
Definition: GridExpression.hpp:416
RDFCodeCalculator.
Definition: RDFCodeCalculator.hpp:53
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
void enableDistanceToIntervalCenterRounding(bool enable)
Allows to specify whether entity pair distances should be rounded to the nearest radius interval cent...
Definition: RDFCodeCalculator.hpp:297
The namespace of the Chemical Data Processing Library.
T EntityType
Definition: RDFCodeCalculator.hpp:56
void setEntityPairWeightFunction(const EntityPairWeightFunction &func)
Allows to specify a custom entity pair weight function.
Definition: RDFCodeCalculator.hpp:285
Definition of matrix data types.
void setEntity3DCoordinatesFunction(const Entity3DCoordinatesFunction &func)
Allows to specify the entity 3D coordinates function.
Definition: RDFCodeCalculator.hpp:291
double getSmoothingFactor() const
Returns the smoothing factor used in the calculation of entity pair RDF contributions.
Definition: RDFCodeCalculator.hpp:255
void setNumSteps(std::size_t num_steps)
Sets the number of desired radius incrementation steps.
Definition: RDFCodeCalculator.hpp:249
bool distanceToIntervalsCenterRoundingEnabled() const
Tells whether entity pair distances get rounded to the nearest radius interval centers.
Definition: RDFCodeCalculator.hpp:303
Definition of vector data types.
VectorNorm2< E >::ResultType length(const VectorExpression< E > &e)
Definition: VectorExpression.hpp:553