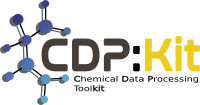 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_QUATERNION_HPP
28 #define CDPL_MATH_QUATERNION_HPP
32 #include <type_traits>
55 typedef typename std::conditional<std::is_const<Q>::value,
56 typename Q::ConstReference,
117 data.operator=(
r.data);
121 template <
typename E>
128 template <
typename T>
136 template <
typename E>
143 template <
typename T>
151 template <
typename E>
158 template <
typename T>
166 template <
typename E>
173 template <
typename T>
181 template <
typename E>
188 template <
typename T>
196 template <
typename E>
203 template <
typename E>
210 template <
typename E>
230 data.set(c1, c2, c3, c4);
237 template <
typename T>
267 std::copy(q.data, q.data + 4, data);
270 template <
typename E>
273 quaternionAssignQuaternion<ScalarAssignment>(*
this, e);
338 std::copy(q.data, q.data + 4, data);
343 template <
typename C>
349 template <
typename E>
357 template <
typename T1>
358 typename std::enable_if<IsScalar<T1>::value,
Quaternion>::type&
369 template <
typename T1>
370 typename std::enable_if<IsScalar<T1>::value,
Quaternion>::type&
377 template <
typename C>
383 template <
typename E>
391 template <
typename T1>
392 typename std::enable_if<IsScalar<T1>::value,
Quaternion>::type&
399 template <
typename C>
405 template <
typename E>
413 template <
typename T1>
414 typename std::enable_if<IsScalar<T1>::value,
Quaternion>::type&
417 quaternionAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
421 template <
typename E>
429 template <
typename T1>
430 typename std::enable_if<IsScalar<T1>::value,
Quaternion>::type&
433 quaternionAssignScalar<ScalarDivisionAssignment>(*
this, t);
437 template <
typename E>
445 template <
typename E>
448 quaternionAssignQuaternion<ScalarAssignment>(*
this, e);
452 template <
typename E>
455 quaternionAssignQuaternion<ScalarAdditionAssignment>(*
this, e);
459 template <
typename E>
462 quaternionAssignQuaternion<ScalarSubtractionAssignment>(*
this, e);
469 std::swap_ranges(data, data + 4, q.data);
481 template <
typename T>
501 template <
typename T1>
537 template <
typename T1>
544 template <
typename T1>
545 typename std::enable_if<IsScalar<T1>::value,
RealQuaternion>::type&
552 template <
typename T1>
559 template <
typename T1>
560 typename std::enable_if<IsScalar<T1>::value,
RealQuaternion>::type&
567 template <
typename T1>
574 template <
typename T1>
575 typename std::enable_if<IsScalar<T1>::value,
RealQuaternion>::type&
582 template <
typename T1>
589 template <
typename T1>
590 typename std::enable_if<IsScalar<T1>::value,
RealQuaternion>::type&
597 template <
typename T1>
604 template <
typename T1>
605 typename std::enable_if<IsScalar<T1>::value,
RealQuaternion>::type&
612 template <
typename T1>
619 template <
typename T1>
626 template <
typename T1>
636 std::swap(value, q.value);
649 template <
typename T>
652 template <
typename Q>
656 template <
typename Q>
660 template <
typename T>
667 template <
typename T1,
typename T2>
668 Quaternion<typename CommonType<T1, T2>::Type>
669 quat(
const T1& t1,
const T2& t2)
673 return QuaternionType(t1, t2);
676 template <
typename T1,
typename T2,
typename T3>
677 Quaternion<typename CommonType<typename CommonType<T1, T2>::Type, T3>::Type>
678 quat(
const T1& t1,
const T2& t2,
const T3& t3)
682 return QuaternionType(t1, t2, t3);
685 template <
typename T1,
typename T2,
typename T3,
typename T4>
686 Quaternion<typename CommonType<typename CommonType<typename CommonType<T1, T2>::Type, T3>::Type, T4>::Type>
687 quat(
const T1& t1,
const T2& t2,
const T3& t3,
const T4& t4)
691 return QuaternionType(t1, t2, t3, t4);
706 #endif // CDPL_MATH_QUATERNION_HPP
const T & ConstReference
Definition: Quaternion.hpp:246
ConstReference getC1() const
Definition: Quaternion.hpp:306
const unsigned int Q
A generic type that covers any element except hydrogen and carbon.
Definition: AtomType.hpp:627
T & Reference
Definition: Quaternion.hpp:245
Quaternion & operator-=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:406
ConstPointer getData() const
Definition: Quaternion.hpp:281
QuaternionReference & operator=(const QuaternionReference &r)
Definition: Quaternion.hpp:115
RealQuaternion & operator=(const RealQuaternion &q)
Definition: Quaternion.hpp:531
const QuaternionReference< const SelfType > ConstClosureType
Definition: Quaternion.hpp:492
std::enable_if< IsScalar< T1 >::value, RealQuaternion >::type & operator/=(const T1 &t)
Definition: Quaternion.hpp:606
friend void swap(RealQuaternion &q1, RealQuaternion &q2)
Definition: Quaternion.hpp:639
RealQuaternion & plusAssign(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:620
ConstReference getC4() const
Definition: Quaternion.hpp:521
QuaternionReference & minusAssign(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:211
ConstReference getC1() const
Definition: Quaternion.hpp:85
friend void swap(QuaternionReference &r1, QuaternionReference &r2)
Definition: Quaternion.hpp:222
QuaternionReference & operator*=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:167
std::enable_if< IsScalar< T >::value, QuaternionReference >::type & operator*=(const T &t)
Definition: Quaternion.hpp:175
Definition: Quaternion.hpp:483
Quaternion & operator=(const Quaternion &q)
Definition: Quaternion.hpp:335
SelfType ClosureType
Definition: Quaternion.hpp:59
Quaternion & operator+=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:384
friend void swap(Quaternion &q1, Quaternion &q2)
Definition: Quaternion.hpp:472
std::enable_if< IsScalar< T1 >::value, Quaternion >::type & operator+=(const T1 &t)
Definition: Quaternion.hpp:371
QuaternionReference & assign(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:197
std::enable_if< IsScalar< T >::value, RealQuaternion< T > >::type quat(const T &t)
Definition: Quaternion.hpp:662
const T & ConstReference
Definition: Quaternion.hpp:490
RealQuaternion()
Definition: Quaternion.hpp:495
ValueType ArrayType[4]
Definition: Quaternion.hpp:247
QuaternionReference(QuaternionType &q)
Definition: Quaternion.hpp:62
void swap(Quaternion &q)
Definition: Quaternion.hpp:466
Definition: Quaternion.hpp:239
T * Pointer
Definition: Quaternion.hpp:248
void swap(RealQuaternion &q)
Definition: Quaternion.hpp:633
RealQuaternion< unsigned long > ULRealQuaternion
Definition: Quaternion.hpp:702
std::enable_if< IsScalar< T1 >::value, RealQuaternion >::type & operator-=(const T1 &t)
Definition: Quaternion.hpp:576
const SelfType ConstClosureType
Definition: Quaternion.hpp:60
ConstReference getC3() const
Definition: Quaternion.hpp:95
Definition of various quaternion expression types and operations.
Definition: Expression.hpp:186
ConstReference getC4() const
Definition: Quaternion.hpp:321
RealQuaternion & operator-=(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:568
std::enable_if< IsScalar< T1 >::value, Quaternion >::type & operator*=(const T1 &t)
Definition: Quaternion.hpp:415
std::enable_if< IsScalar< T >::value, QuaternionReference >::type & operator+=(const T &t)
Definition: Quaternion.hpp:145
ConstReference getC2() const
Definition: Quaternion.hpp:511
ConstReference getC1() const
Definition: Quaternion.hpp:506
Definition: Expression.hpp:98
const T & Reference
Definition: Quaternion.hpp:489
Q::ConstReference ConstReference
Definition: Quaternion.hpp:58
Quaternion & operator=(const QuaternionContainer< C > &c)
Definition: Quaternion.hpp:344
std::enable_if< IsScalar< T1 >::value, Quaternion >::type & operator/=(const T1 &t)
Definition: Quaternion.hpp:431
Q QuaternionType
Definition: Quaternion.hpp:53
std::enable_if< IsScalar< T >::value, QuaternionReference >::type & operator=(const T &t)
Definition: Quaternion.hpp:130
T ValueType
Definition: Quaternion.hpp:488
QuaternionReference & operator=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:122
RealQuaternion< float > FRealQuaternion
Definition: Quaternion.hpp:699
Quaternion(const Quaternion &q)
Definition: Quaternion.hpp:265
ConstReference getC3() const
Definition: Quaternion.hpp:316
std::enable_if< IsScalar< T >::value, QuaternionReference >::type & operator-=(const T &t)
Definition: Quaternion.hpp:160
ConstReference getC3() const
Definition: Quaternion.hpp:516
RealQuaternion & operator*=(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:583
QuaternionReference & operator+=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:137
Reference getC3()
Definition: Quaternion.hpp:296
ConstReference getC2() const
Definition: Quaternion.hpp:311
std::conditional< std::is_const< Q >::value, typename Q::ConstReference, typename Q::Reference >::type Reference
Definition: Quaternion.hpp:57
Quaternion(const ValueType &c1, const ValueType &c2=ValueType(), const ValueType &c3=ValueType(), const ValueType &c4=ValueType())
Definition: Quaternion.hpp:256
Definition of type traits.
Reference getC3()
Definition: Quaternion.hpp:75
SelfType QuaternionTemporaryType
Definition: Quaternion.hpp:252
Quaternion & plusAssign(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:453
const QuaternionType & getData() const
Definition: Quaternion.hpp:105
RealQuaternion< long > LRealQuaternion
Definition: Quaternion.hpp:701
void set(const ValueType &c1=ValueType(), const ValueType &c2=ValueType(), const ValueType &c3=ValueType(), const ValueType &c4=ValueType())
Definition: Quaternion.hpp:227
RealQuaternion(const ValueType &r)
Definition: Quaternion.hpp:498
Reference getC1()
Definition: Quaternion.hpp:286
Definition of various functors.
Quaternion & minusAssign(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:460
Definition: TypeTraits.hpp:193
RealQuaternion & assign(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:613
ConstReference getC4() const
Definition: Quaternion.hpp:100
Implementation of quaternion assignment routines.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
const T * ConstPointer
Definition: Quaternion.hpp:249
std::enable_if< IsScalar< T1 >::value, Quaternion >::type & operator-=(const T1 &t)
Definition: Quaternion.hpp:393
Quaternion & operator=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:350
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
Quaternion< long > LQuaternion
Definition: Quaternion.hpp:696
RealQuaternion & operator=(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:538
Quaternion & operator+=(const QuaternionContainer< C > &c)
Definition: Quaternion.hpp:378
T ValueType
Definition: Quaternion.hpp:244
Pointer getData()
Definition: Quaternion.hpp:276
ConstReference getC2() const
Definition: Quaternion.hpp:90
std::enable_if< IsScalar< T1 >::value, RealQuaternion >::type & operator=(const T1 &t)
Definition: Quaternion.hpp:546
RealQuaternion & minusAssign(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:627
QuaternionReference & operator-=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:152
The namespace of the Chemical Data Processing Library.
QuaternionType & getData()
Definition: Quaternion.hpp:110
RealQuaternion & operator+=(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:553
Reference getC4()
Definition: Quaternion.hpp:80
std::enable_if< IsScalar< T1 >::value, RealQuaternion >::type & operator+=(const T1 &t)
Definition: Quaternion.hpp:561
const QuaternionReference< const SelfType > ConstClosureType
Definition: Quaternion.hpp:251
Definition: Quaternion.hpp:48
Quaternion & operator-=(const QuaternionContainer< C > &c)
Definition: Quaternion.hpp:400
RealQuaternion(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:502
void set(const ValueType &c1=ValueType(), const ValueType &c2=ValueType(), const ValueType &c3=ValueType(), const ValueType &c4=ValueType())
Definition: Quaternion.hpp:326
Q::ValueType ValueType
Definition: Quaternion.hpp:54
QuaternionReference & plusAssign(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:204
QuaternionReference< SelfType > ClosureType
Definition: Quaternion.hpp:250
Quaternion & operator*=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:422
Quaternion & assign(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:446
QuaternionReference< SelfType > ClosureType
Definition: Quaternion.hpp:491
Quaternion()
Definition: Quaternion.hpp:254
Quaternion(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:271
std::enable_if< IsScalar< T1 >::value, RealQuaternion >::type & operator*=(const T1 &t)
Definition: Quaternion.hpp:591
RealQuaternion & operator/=(const RealQuaternion< T1 > &q)
Definition: Quaternion.hpp:598
Quaternion< float > FQuaternion
Definition: Quaternion.hpp:694
Reference getC2()
Definition: Quaternion.hpp:70
std::enable_if< IsScalar< T >::value, QuaternionReference >::type & operator/=(const T &t)
Definition: Quaternion.hpp:190
RealQuaternion< double > DRealQuaternion
Definition: Quaternion.hpp:700
Quaternion< unsigned long > ULQuaternion
Definition: Quaternion.hpp:697
QuaternionReference & operator/=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:182
void swap(QuaternionReference &r)
Definition: Quaternion.hpp:217
std::enable_if< IsScalar< T1 >::value, Quaternion >::type & operator=(const T1 &t)
Definition: Quaternion.hpp:359
Quaternion< double > DQuaternion
Definition: Quaternion.hpp:695
Reference getC4()
Definition: Quaternion.hpp:301
Quaternion< T > QuaternionTemporaryType
Definition: Quaternion.hpp:493
Reference getC1()
Definition: Quaternion.hpp:65
Reference getC2()
Definition: Quaternion.hpp:291
Quaternion & operator/=(const QuaternionExpression< E > &e)
Definition: Quaternion.hpp:438