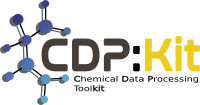 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_QTRENDERER2D_HPP
30 #define CDPL_VIS_QTRENDERER2D_HPP
83 void drawLine(
double x1,
double y1,
double x2,
double y2);
87 void drawText(
double x,
double y,
const std::string& txt);
88 void drawEllipse(
double x,
double y,
double width,
double height);
102 std::unique_ptr<QPolygonF> qPolygon;
103 std::unique_ptr<QPainterPath> qPainterPath;
108 #endif // CDPL_VIS_QTRENDERER2D_HPP
An interface that provides methods for low level 2D drawing operations.
Definition: Renderer2D.hpp:86
void drawPath(const Path2D &path)
Draws the given path.
void restoreState()
Restores the last renderer state saved by a call to saveState().
void drawLineSegments(const Math::Vector2DArray &points)
Draws the sequence of disjoint line segments defined by points.
Specifies how to draw lines and outlines of shapes.
Definition: Pen.hpp:53
void setFont(const Font &font)
Sets the font to be used in subsequent text drawing operations.
void drawText(double x, double y, const std::string &txt)
Draws the specified text at the position (x, y).
Specifies a font for drawing text.
Definition: Font.hpp:54
Provides a container for painting operations, enabling arbitrary graphical shapes to be constructed a...
Definition: Path2D.hpp:78
void drawEllipse(double x, double y, double width, double height)
Draws an ellipse with the given width and height around the center position (x, y).
void setTransform(const Math::Matrix3D &xform)
Sets the applied affine transformation matrix to xform.
void drawRectangle(double x1, double y1, double x2, double y2)
Draws an axis aligned rectangle of the specified width and height whose upper-left corner is located ...
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
Definition of the class CDPL::Vis::Renderer2D.
void clearClipPath()
Disables clipping.
void setPen(const Pen &pen)
Sets the pen to be used in subsequent drawing operations.
The namespace of the Chemical Data Processing Library.
void setBrush(const Brush &brush)
Sets the brush to be used in subsequent drawing operations.
~QtRenderer2D()
Destructor.
void transform(const Math::Matrix3D &xform)
Multiplies the current affine transformation matrix by xform.
void drawLine(double x1, double y1, double x2, double y2)
Draws a line segment from (x1, y1) to (x2, y2).
Specifies the fill pattern and fill color of shapes.
Definition: Brush.hpp:50
void drawPoint(double x, double y)
Draws a point at the position (x, y).
void drawPolygon(const Math::Vector2DArray &points)
Draws the polygon defined by points.
VectorArray< Vector2D > Vector2DArray
An array of Math::Vector2D objects.
Definition: VectorArray.hpp:79
#define CDPL_VIS_QT_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition of the preprocessor macro CDPL_VIS_API.
void saveState()
Saves the current renderer state.
void drawPolyline(const Math::Vector2DArray &points)
Draws the polyline defined by points.
QtRenderer2D(QPainter &painter)
Constructs a renderer object which uses the QPainter instance painter for its drawing operations.
Implements the Renderer2D interface on top of the Qt Toolkit.
Definition: QtRenderer2D.hpp:57
void setClipPath(const Path2D &path)