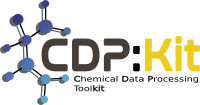 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_QTFONTMETRICS_HPP
30 #define CDPL_VIS_QTFONTMETRICS_HPP
88 QPaintDevice* qPaintDevice;
89 std::unique_ptr<QFontMetricsF> qFontMetrics;
94 #endif // CDPL_VIS_QTFONTMETRICS_HPP
void setFont(const Font &font)
Specifies the font for which to obtain the metrics.
Implements the FontMetrics interface for the Qt rendering backend.
Definition: QtFontMetrics.hpp:56
double getWidth(char ch) const
Returns the advance width for the character ch.
double getWidth(const std::string &str) const
Returns the total advance width for the characters in the string str.
void getBounds(char ch, Rectangle2D &bounds) const
Returns the bounding rectangle of the character ch relative to the left-most point on the baseline.
Specifies a font for drawing text.
Definition: Font.hpp:54
Definition of the class CDPL::Vis::FontMetrics.
void getBounds(const std::string &str, Rectangle2D &bounds) const
Returns the total bounding rectangle for the characters in the string str.
QtFontMetrics(QPaintDevice *paint_dev=0)
Constructs a font metrics object for Qt's default font and the given paint device.
double getLeading() const
Returns the leading of the font.
double getAscent() const
Returns the ascent of the font.
double getHeight() const
Returns the height of the font.
~QtFontMetrics()
Destructor.
The namespace of the Chemical Data Processing Library.
Specifies an axis aligned rectangular area in 2D space.
Definition: Rectangle2D.hpp:51
double getDescent() const
Returns the descent of the font.
An interface class with methods that provide information about the metrics of a font.
Definition: FontMetrics.hpp:71
#define CDPL_VIS_QT_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition of the preprocessor macro CDPL_VIS_API.