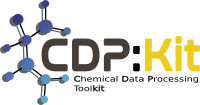 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_PROPERTYMATCHEXPRESSION_HPP
30 #define CDPL_CHEM_PROPERTYMATCHEXPRESSION_HPP
55 template <
typename ValueType,
typename MatchFunc,
typename ObjType1,
typename ObjType2 =
void>
79 value(), matchFunc(), propertyFunc(property_func), fixed(false) {}
88 value(value), matchFunc(), propertyFunc(property_func), fixed(true) {}
104 bool operator()(
const ObjType1& query_obj1,
const ObjType2& query_obj2,
const ObjType1& target_obj1,
105 const ObjType2& target_obj2,
const Base::Any& aux_data)
const;
124 template <
typename ValueType,
typename MatchFunc,
typename ObjType>
148 value(), matchFunc(), propertyFunc(property_func), fixed(false) {}
157 value(value), matchFunc(), propertyFunc(property_func), fixed(true) {}
171 bool operator()(
const ObjType& query_obj,
const ObjType& target_obj,
const Base::Any& aux_data)
const;
185 template <
typename ValueType,
typename MatchFunc,
typename ObjType1,
typename ObjType2>
187 const ObjType1& target_obj1,
const ObjType2& target_obj2,
191 return matchFunc(propertyFunc(target_obj1, target_obj2), value);
193 return matchFunc(propertyFunc(target_obj1, target_obj2), propertyFunc(query_obj1, query_obj2));
197 template <
typename ValueType,
typename MatchFunc,
typename ObjType>
202 return matchFunc(propertyFunc(target_obj), value);
204 return matchFunc(propertyFunc(target_obj), propertyFunc(query_obj));
207 #endif // CDPL_CHEM_PROPERTYMATCHEXPRESSION_HPP
PropertyMatchExpression(const ValueType &value, const PropertyFunction &property_func)
Constructs a PropertyMatchExpression instance that performs query/target object equivalence tests bas...
Definition: PropertyMatchExpression.hpp:156
PropertyMatchExpression(const ValueType &value, const PropertyFunction &property_func)
Constructs a PropertyMatchExpression instance that performs query/target object equivalence tests bas...
Definition: PropertyMatchExpression.hpp:87
PropertyMatchExpression(const PropertyFunction &property_func)
Constructs a PropertyMatchExpression instance that performs query/target object equivalence tests bas...
Definition: PropertyMatchExpression.hpp:78
std::shared_ptr< PropertyMatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated PropertyMatchExpression instances...
Definition: PropertyMatchExpression.hpp:63
bool operator()(const ObjType1 &query_obj1, const ObjType2 &query_obj2, const ObjType1 &target_obj1, const ObjType2 &target_obj2, const Base::Any &aux_data) const
Checks whether the value of the target object propery matches the query property value.
Definition: PropertyMatchExpression.hpp:186
std::function< ValueType(const ObjType1 &, const ObjType2 &)> PropertyFunction
Type of the generic functor class used to store user-defined property accessor functions.
Definition: PropertyMatchExpression.hpp:71
std::shared_ptr< PropertyMatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated PropertyMatchExpression instances...
Definition: PropertyMatchExpression.hpp:132
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
PropertyMatchExpression(const PropertyFunction &property_func)
Constructs a PropertyMatchExpression instance that performs query/target object equivalence tests bas...
Definition: PropertyMatchExpression.hpp:147
PropertyMatchExpression.
Definition: PropertyMatchExpression.hpp:57
std::function< ValueType(const ObjType &)> PropertyFunction
Type of the generic functor class used to store user-defined property accessor functions.
Definition: PropertyMatchExpression.hpp:140