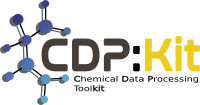 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BASE_PROPERTYCONTAINER_HPP
30 #define CDPL_BASE_PROPERTYCONTAINER_HPP
33 #include <unordered_map>
77 typedef std::unordered_map<LookupKey, Any, LookupKey::HashFunc> PropertyMap;
105 template <
typename T>
106 void setProperty(
const LookupKey& key,
T&& val);
119 template <
typename T>
120 const T& getProperty(
const LookupKey& key)
const;
135 template <
typename T>
136 const T& getPropertyOrDefault(
const LookupKey& key,
const T& def_val)
const;
150 inline const Any& getProperty(
const LookupKey& key,
bool throw_ =
false)
const;
157 inline bool isPropertySet(
const LookupKey& key)
const;
251 inline bool isEmptyAny(
const Any& val)
const;
253 template <
typename T>
254 bool isEmptyAny(
const T& val)
const;
256 PropertyMap properties;
264 template <
typename T>
267 return getProperty(key,
true).template getData<T>();
270 template <
typename T>
273 const Any& val = getProperty(key,
false);
275 return (val.
isEmpty() ? def_val : val.template getData<T>());
280 static const Any NOT_FOUND;
284 if (it != properties.end())
293 template <
typename T>
296 if (isEmptyAny(val)) {
297 properties.erase(key);
301 properties[key] = std::forward<T>(val);
306 return (properties.find(key) != properties.end());
309 bool CDPL::Base::PropertyContainer::isEmptyAny(
const Any& val)
const
314 template <
typename T>
315 bool CDPL::Base::PropertyContainer::isEmptyAny(
const T&)
const
325 #endif // CDPL_BASE_PROPERTYCONTAINER_HPP
void swap(PropertyContainer &cntnr)
Exchanges the properties of this container with the properties of the container cntnr.
bool isEmpty() const noexcept
Tells whether the Any instance stores any data.
Definition: Any.hpp:182
ConstPropertyIterator getPropertiesBegin() const
Returns a constant iterator pointing to the beginning of the property entries.
PropertyMap::const_iterator ConstPropertyIterator
A constant iterator used to iterate over the property entries.
Definition: PropertyContainer.hpp:88
void copyProperties(const PropertyContainer &cntnr)
Replaces the current set of properties by a copy of the entries in cntnr.
#define CDPL_BASE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
virtual ~PropertyContainer()
Virtual destructor.
std::size_t getNumProperties() const
Returns the number of property entries.
Thrown to indicate that some requested data item could not be found.
Definition: Base/Exceptions.hpp:171
void clearProperties()
Clears all property values.
const std::string & getName() const
Returns the name of the LookupKey instance.
An unique lookup key for control-parameter and property values.
Definition: LookupKey.hpp:54
Definition of the preprocessor macro CDPL_BASE_API.
void addProperties(const PropertyContainer &cntnr)
Adds the property value entries in the PropertyContainer instance cntnr.
Definition of the class CDPL::Base::LookupKey.
PropertyMap::value_type PropertyEntry
A Base::LookupKey / Base::Any pair that stores the property value for a given property key.
Definition: PropertyContainer.hpp:83
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
ConstPropertyIterator begin() const
Returns a constant iterator pointing to the beginning of the property entries.
bool isPropertySet(const LookupKey &key) const
Tells whether or not a value has been assigned to the property specified by key.
Definition: PropertyContainer.hpp:304
const PropertyContainer & getProperties() const
Returns a const reference to itself.
Definition: PropertyContainer.hpp:320
void setProperty(const LookupKey &key, T &&val)
Sets the value of the property specified by key to val.
Definition: PropertyContainer.hpp:294
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Definition of exception classes.
A class providing methods for the storage and lookup of object properties.
Definition: PropertyContainer.hpp:75
PropertyContainer()
Constructs an empty PropertyContainer instance.
Definition: PropertyContainer.hpp:227
ConstPropertyIterator end() const
Returns a constant iterator pointing to the end of the property entries.
The namespace of the Chemical Data Processing Library.
bool removeProperty(const LookupKey &key)
Clears the value of the property specified by key.
PropertyContainer & operator=(const PropertyContainer &cntnr)
Assignment operator.
const T & getPropertyOrDefault(const LookupKey &key, const T &def_val) const
Returns the value of the property specified by key as a const reference to an object of type T,...
Definition: PropertyContainer.hpp:271
Definition of the class CDPL::Base::Any.
PropertyContainer(const PropertyContainer &cntnr)
Constructs a copy of the PropertyContainer instance cntnr.
const T & getProperty(const LookupKey &key) const
Returns the value of the property specified by key as a const reference to an object of type T.
Definition: PropertyContainer.hpp:265
ConstPropertyIterator getPropertiesEnd() const
Returns a constant iterator pointing to the end of the property entries.