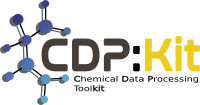 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_PRINCIPALAXESALIGNMENTSTARTGENERATOR_HPP
30 #define CDPL_SHAPE_PRINCIPALAXESALIGNMENTSTARTGENERATOR_HPP
34 #include <boost/random/mersenne_twister.hpp>
52 static constexpr
double DEF_SYMMETRY_THRESHOLD = 0.15;
53 static constexpr std::size_t DEF_NUM_RANDOM_STARTS = 4;
54 static constexpr
double DEF_MAX_RANDOM_TRANSLATION = 2.0;
115 void generateForElementCenters(
const GaussianShapeFunction& func,
unsigned int axes_swap_flags,
bool ref_shape);
116 void generate(
const Math::Vector3D& ctr_trans,
unsigned int axes_swap_flags);
118 template <
typename QE>
121 typedef std::vector<QuaternionTransformation> StartTransformList;
122 typedef boost::random::mt11213b RandomEngine;
126 bool nonColCtrStarts;
128 bool genForAlgdShape;
130 bool genForLargerShape;
131 StartTransformList startTransforms;
134 double maxRandomTrans;
135 std::size_t numRandomStarts;
136 unsigned int refAxesSwapFlags;
137 std::size_t numSubTransforms;
138 RandomEngine randomEngine;
143 #endif // CDPL_SHAPE_PRINCIPALAXESALIGNMENTSTARTGENERATOR_HPP
Definition: GaussianShapeAlignmentStartGenerator.hpp:49
void setReference(const GaussianShapeFunction &func, unsigned int sym_class)
unsigned int setupAligned(GaussianShapeFunction &func, Math::Matrix4D &xform) const
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
Definition: PrincipalAxesAlignmentStartGenerator.hpp:49
Definition of the class CDPL::Shape::GaussianShapeAlignmentStartGenerator.
const QuaternionTransformation & getStartTransform(std::size_t idx) const
bool genForLargerShapeCenters() const
bool genForReferenceShapeCenters() const
double getSymmetryThreshold()
void setSymmetryThreshold(double thresh)
void genShapeCenterStarts(bool generate)
Definition of various quaternion expression types and operations.
Definition: Expression.hpp:98
bool genForAlignedShapeCenters() const
void genRandomStarts(bool generate)
void setRandomSeed(unsigned int seed)
double getMaxRandomTranslation() const
void setMaxRandomTranslation(double max_trans)
void setNumRandomStarts(std::size_t num_starts)
void genNonColorCenterStarts(bool generate)
std::size_t getNumStartTransforms() const
unsigned int setupReference(GaussianShapeFunction &func, Math::Matrix4D &xform) const
void genForLargerShapeCenters(bool generate)
bool genRandomStarts() const
Definition: Vector.hpp:1053
bool genNonColorCenterStarts() const
std::size_t getNumStartSubTransforms() const
Definition: GaussianShapeFunction.hpp:53
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::size_t getNumRandomStarts() const
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
bool genColorCenterStarts() const
void genColorCenterStarts(bool generate)
bool genShapeCenterStarts() const
void genForAlignedShapeCenters(bool generate)
bool generate(const GaussianShapeFunction &func, unsigned int sym_class)
PrincipalAxesAlignmentStartGenerator()
void genForReferenceShapeCenters(bool generate)
Definition of vector data types.