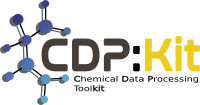 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_PEN_HPP
30 #define CDPL_VIS_PEN_HPP
254 #endif // CDPL_VIS_PEN_HPP
const Color & getColor() const
Returns the color of the pen.
void setCapStyle(CapStyle cap_style)
Sets the line cap style to cap_style.
@ NO_LINE
Tells the renderer not to draw lines or shape outlines.
Definition: Pen.hpp:65
@ DASH_DOT_LINE
Specifies a line with alternating dots and dashes.
Definition: Pen.hpp:85
@ SQUARE_CAP
Specifies a squared line end that covers the end point and extends beyond it with half the line width...
Definition: Pen.hpp:107
@ FLAT_CAP
Specifies a squared line end that does not cover the end point of the line.
Definition: Pen.hpp:102
CapStyle
Defines constants for supported line cap styles.
Definition: Pen.hpp:97
@ MITER_JOIN
Specifies a join style where the outer edges of the lines are extended to meet at an angle,...
Definition: Pen.hpp:124
@ SOLID_LINE
Specifies a solid line without gaps.
Definition: Pen.hpp:70
Specifies how to draw lines and outlines of shapes.
Definition: Pen.hpp:53
void setWidth(double width)
Sets the line width to the specified value.
void setLineStyle(LineStyle line_style)
Sets the line style to line_style.
@ DOT_LINE
Specifies a dotted line.
Definition: Pen.hpp:80
bool operator==(const Pen &pen) const
Equality comparison operator.
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Pen(LineStyle line_style)
Constructs a pen with the specified line style, line width 1.0, cap style Pen::ROUND_CAP,...
Pen(const Color &color, double width=1.0, LineStyle line_style=SOLID_LINE, CapStyle cap_style=ROUND_CAP, JoinStyle join_style=ROUND_JOIN)
Constructs a pen with the specified color, line width, line style, cap style and join style.
double getWidth() const
Returns the line width.
void setColor(const Color &color)
Sets the pen's color to the specified value.
CapStyle getCapStyle() const
Returns the line cap style.
void setJoinStyle(JoinStyle join_style)
Sets the line join style to join_style.
The namespace of the Chemical Data Processing Library.
JoinStyle getJoinStyle() const
Returns the line join style.
LineStyle
Defines constants for supported line styles.
Definition: Pen.hpp:60
JoinStyle
Defines constants for supported line join styles.
Definition: Pen.hpp:119
LineStyle getLineStyle() const
Returns the line style.
Definition of the class CDPL::Vis::Color.
Definition of the preprocessor macro CDPL_VIS_API.
Specifies a color in terms of its red, green and blue components and an alpha-channel for transparenc...
Definition: Color.hpp:52
bool operator!=(const Pen &pen) const
Inequality comparison operator.
@ BEVEL_JOIN
Specifies a join style where the triangular notch between the two lines is filled.
Definition: Pen.hpp:129
@ DASH_LINE
Specifies a dashed line.
Definition: Pen.hpp:75
Pen()
Constructs a pen with line style Pen::SOLID_LINE, line width 1.0, cap style Pen::ROUND_CAP,...