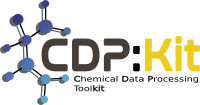 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_PATH2D_HPP
30 #define CDPL_VIS_PATH2D_HPP
44 class Path2DConverter;
184 void arc(
double cx,
double cy,
double rx,
double ry,
double start_ang,
double sweep);
221 void arcTo(
double cx,
double cy,
double rx,
double ry,
double start_ang,
double sweep);
279 void addEllipse(
double x,
double y,
double width,
double height);
352 typedef std::vector<Element> ElementList;
355 ElementList elements;
360 #endif // CDPL_VIS_PATH2D_HPP
void setFillRule(FillRule rule)
Sets the fill rule of the path to the specified value.
void addEllipse(const Math::Vector2D &pos, double width, double height)
Creates an ellipse positioned at pos with the specified width and height, and adds it to this path as...
FillRule
Specifies which method to use for filling closed shapes described by the Path2D object.
Definition: Path2D.hpp:85
Path2D()
Constructs an empty Path2D instance.
void arcTo(double cx, double cy, double rx, double ry, double start_ang, double sweep)
Creates an elliptical arc centered at (cx, cy) with ellipse radii rx and ry beginning at the angle st...
void moveTo(const Math::Vector2D &pos)
Sets the current position to pos and starts a new subpath, implicitly closing the previous path.
void arc(double cx, double cy, double rx, double ry, double start_ang, double sweep)
Creates an elliptical arc centered at (cx, cy) with ellipse radii rx and ry beginning at the angle st...
Path2D(const Path2D &path)
Constructs a copy of the Path2D instance path.
Provides a container for painting operations, enabling arbitrary graphical shapes to be constructed a...
Definition: Path2D.hpp:78
void clear()
Deletes all path elements added so far and sets the fill rule to its default.
void lineTo(double x, double y)
Adds a straight line from the current position to the point (x, y).
void arcTo(const Math::Vector2D &ctr, double rx, double ry, double start_ang, double sweep)
Creates an elliptical arc centered at ctr with ellipse radii rx and ry beginning at the angle start_a...
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void addRectangle(const Math::Vector2D &pos, double width, double height)
Creates a rectangle positioned at pos with the specified width and height, and adds it to this path a...
FillRule getFillRule() const
Returns the currently set fill rule.
void addEllipse(double x, double y, double width, double height)
Creates an ellipse positioned at (x, y) with the specified width and height, and adds it to this path...
bool operator!=(const Path2D &path) const
Non-equality comparison operator.
void getBounds(Rectangle2D &bounds) const
Calculates the axis-aligned bounding box of the path.
void moveTo(double x, double y)
Sets the current position to (x, y) and starts a new subpath, implicitly closing the previous path.
void lineTo(const Math::Vector2D &pos)
Adds a straight line from the current position to the point (x, y).
Provides an interface for classes that implement the conversion of Vis::Path2D objects into rendering...
Definition: Path2DConverter.hpp:48
The namespace of the Chemical Data Processing Library.
void closePath()
Closes the current subpath by drawing a line to the beginning of the subpath, automatically starting ...
Specifies an axis aligned rectangular area in 2D space.
Definition: Rectangle2D.hpp:51
Path2D & operator=(const Path2D &path)
Assignment operator.
void convert(Path2DConverter &conv) const
Iterates over the stored path elements and calls the corresponding method of conv for each visited el...
CVector< double, 2 > Vector2D
A bounded 2 element vector holding floating point values of type double.
Definition: Vector.hpp:1632
bool hasDrawingElements() const
Tells whether the Path2D instance contains any elements representing drawing operations (lines and ar...
void arc(const Math::Vector2D &ctr, double rx, double ry, double start_ang, double sweep)
Creates an elliptical arc centered at ctr with ellipse radii rx and ry beginning at the angle start_a...
virtual ~Path2D()
Virtual destructor.
Path2D & operator+=(const Path2D &path)
Appends the elements stored in the Path2D instance path to this path.
void addRectangle(double x, double y, double width, double height)
Creates a rectangle positioned at (x, y) with the specified width and height, and adds it to this pat...
Definition of the preprocessor macro CDPL_VIS_API.
bool operator==(const Path2D &path) const
Equality comparison operator.
bool isEmpty() const
Tells whether the Path2D instance does not contain any elements.
Definition of vector data types.
@ EVEN_ODD
Specifies that the path has to be filled using the odd even fill rule.
Definition: Path2D.hpp:94