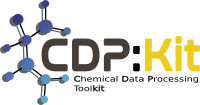 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
32 #ifndef CDPL_MOLPROP_PEOESIGMACHARGECALCULATOR_HPP
33 #define CDPL_MOLPROP_PEOESIGMACHARGECALCULATOR_HPP
55 class MHMOPiChargeCalculator;
67 static constexpr std::size_t DEF_NUM_ITERATIONS = 20;
68 static constexpr
double DEF_DAMPING_FACTOR = 0.48;
140 double getNbrElectronegativityAvg(std::size_t idx)
const;
151 void linkTo(AtomState* nbr_state);
153 double getCharge()
const;
154 double getElectronegativity()
const;
156 void shiftCharges(
double att_fact);
157 void updateElectronegativity();
159 double getNbrElectronegativityAvg()
const;
162 typedef std::vector<AtomState*> AtomStateList;
164 AtomStateList nbrAtomStates;
169 double enegativityP1;
173 typedef std::vector<AtomState::SharedPointer> AtomStateList;
175 std::size_t numIterations;
176 double dampingFactor;
177 AtomStateList atomStates;
178 AtomStateList implHStates;
183 #endif // CDPL_MOLPROP_PEOESIGMACHARGECALCULATOR_HPP
void calculate(const Chem::MolecularGraph &molgraph)
Calculates the sigma charges and electronegativities of the atoms in the molecular graph molgraph by ...
std::shared_ptr< PEOESigmaChargeCalculator > SharedPointer
Definition: PEOESigmaChargeCalculator.hpp:65
double getElectronegativity(std::size_t idx) const
Returns the calculated sigma electronegativity of the atom with index idx.
Atom.
Definition: Atom.hpp:52
PEOESigmaChargeCalculator()
Constructs the PEOESigmaChargeCalculator instance.
PEOESigmaChargeCalculator(const Chem::MolecularGraph &molgraph)
Constructs the PEOESigmaChargeCalculator instance and calculates the sigma charges and electronegativ...
MolecularGraph.
Definition: MolecularGraph.hpp:52
MHMOPiChargeCalculator.
Definition: MHMOPiChargeCalculator.hpp:67
std::size_t getNumIterations() const
Returns the number of performed charge shifting iterations.
void setNumIterations(std::size_t num_iter)
Allows to specify the number of charge shifting iterations that have to be performed.
PEOESigmaChargeCalculator.
Definition: PEOESigmaChargeCalculator.hpp:62
double getDampingFactor() const
Returns the applied damping factor.
The namespace of the Chemical Data Processing Library.
double getCharge(std::size_t idx) const
Returns the calculated sigma charge of the atom with index idx.
Definition of the preprocessor macro CDPL_MOLPROP_API.
#define CDPL_MOLPROP_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setDampingFactor(double factor)
Allows to specify the applied damping factor.