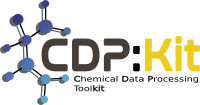 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BIOMOL_PDBDATA_HPP
30 #define CDPL_BIOMOL_PDBDATA_HPP
105 typedef std::map<RecordType, std::string> TypeToDataMap;
108 typedef typename TypeToDataMap::value_type
Record;
166 #endif // CDPL_BIOMOL_PDBDATA_HPP
RecordIterator getRecordsBegin()
@ SCALE2
Definition: PDBData.hpp:97
std::string & getData(const RecordType &type)
@ LINK
Definition: PDBData.hpp:86
@ EXPDTA
Definition: PDBData.hpp:66
A datastructure for the storage of imported PDB data records (see [CTFILE]).
Definition: PDBData.hpp:50
@ CISPEP
Definition: PDBData.hpp:87
TypeToDataMap::value_type Record
Definition: PDBData.hpp:108
@ MTRIX3
Definition: PDBData.hpp:92
@ COMPND
Definition: PDBData.hpp:63
@ STRUCTURE_ID
Definition: PDBData.hpp:99
@ MTRIX1
Definition: PDBData.hpp:90
@ SCALE1
Definition: PDBData.hpp:96
ConstRecordIterator end() const
RecordIterator setRecord(const RecordType &type, const std::string &data)
@ SCALE3
Definition: PDBData.hpp:98
ConstRecordIterator getRecordsBegin() const
@ FORMUL
Definition: PDBData.hpp:79
@ AUTHOR
Definition: PDBData.hpp:67
@ REMARK
Definition: PDBData.hpp:71
@ REVDAT
Definition: PDBData.hpp:68
RecordIterator getRecordsEnd()
@ DEPOSITION_DATE
Definition: PDBData.hpp:100
@ HETNAM
Definition: PDBData.hpp:80
RecordType
Definition: PDBData.hpp:56
ConstRecordIterator getRecord(const RecordType &type) const
@ SPLIT
Definition: PDBData.hpp:61
@ SEQADV
Definition: PDBData.hpp:75
bool removeRecord(const RecordType &type)
@ HELIX
Definition: PDBData.hpp:82
PDBData(const PDBData &other)
Definition: PDBData.hpp:117
@ TURN
Definition: PDBData.hpp:84
@ ORIGX2
Definition: PDBData.hpp:94
@ SSBOND
Definition: PDBData.hpp:85
TypeToDataMap::iterator RecordIterator
Definition: PDBData.hpp:112
@ SPRSDE
Definition: PDBData.hpp:69
std::size_t getNumRecords() const
@ HET
Definition: PDBData.hpp:78
@ SOURCE
Definition: PDBData.hpp:64
RecordIterator setRecord(const Record &rec)
@ ORIGX3
Definition: PDBData.hpp:95
Definition of the preprocessor macro CDPL_BIOMOL_API.
TypeToDataMap::const_iterator ConstRecordIterator
Definition: PDBData.hpp:110
The namespace of the Chemical Data Processing Library.
@ ORIGX1
Definition: PDBData.hpp:93
ConstRecordIterator getRecordsEnd() const
@ SEQRES
Definition: PDBData.hpp:76
@ JRNL
Definition: PDBData.hpp:70
@ SITE
Definition: PDBData.hpp:88
const std::string & getData(const RecordType &type) const
@ CRYST1
Definition: PDBData.hpp:89
@ DBREF
Definition: PDBData.hpp:72
@ OBSLTE
Definition: PDBData.hpp:59
RecordIterator getRecord(const RecordType &type)
@ MODRES
Definition: PDBData.hpp:77
@ DBREF2
Definition: PDBData.hpp:74
@ DBREF1
Definition: PDBData.hpp:73
void removeRecord(const RecordIterator &it)
PDBData()
Definition: PDBData.hpp:114
@ CAVEAT
Definition: PDBData.hpp:62
#define CDPL_BIOMOL_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::shared_ptr< PDBData > SharedPointer
Definition: PDBData.hpp:53
ConstRecordIterator begin() const
@ MTRIX2
Definition: PDBData.hpp:91
@ SHEET
Definition: PDBData.hpp:83
@ HETSYN
Definition: PDBData.hpp:81
@ TITLE
Definition: PDBData.hpp:60
bool containsRecord(const RecordType &type) const
@ KEYWDS
Definition: PDBData.hpp:65
@ HEADER
Definition: PDBData.hpp:58