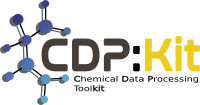 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_OBJECTPOOL_HPP
30 #define CDPL_UTIL_OBJECTPOOL_HPP
90 maxSize(pool.maxSize), ctorFunc(pool.ctorFunc), dtorFunc(pool.dtorFunc),
91 initFunc(pool.initFunc), cleanFunc(pool.cleanFunc) {}
94 maxSize(max_size), ctorFunc(DefaultConstructor()), dtorFunc(DefaultDestructor()) {}
96 template <
typename C,
typename D>
97 ObjectPool(
const C& ctor_func,
const D& dtor_func, std::size_t max_size = 0):
98 maxSize(max_size), ctorFunc(ctor_func), dtorFunc(dtor_func)
103 std::for_each(pool.begin(), pool.end(), dtorFunc);
118 throw std::bad_alloc();
148 std::for_each(pool.begin(), pool.end(), dtorFunc);
168 maxSize = pool.maxSize;
169 ctorFunc = pool.ctorFunc;
170 dtorFunc = pool.dtorFunc;
171 initFunc = pool.initFunc;
179 void shrinkToMaxSize()
184 while (pool.size() > maxSize) {
185 dtorFunc(pool.back());
206 if (maxSize > 0 && pool.size() >= maxSize) {
222 typedef std::vector<ObjectType*> PooledObjectList;
225 PooledObjectList pool;
234 #endif // CDPL_UTIL_OBJECTPOOL_HPP
ObjectPool(const C &ctor_func, const D &dtor_func, std::size_t max_size=0)
Definition: ObjectPool.hpp:97
std::size_t getSize() const
Definition: ObjectPool.hpp:129
ObjectPool & operator=(const ObjectPool &pool)
Definition: ObjectPool.hpp:163
~ObjectPool()
Definition: ObjectPool.hpp:101
void operator()(T *obj) const
Definition: ObjectPool.hpp:83
SharedObjectPointer get()
Definition: ObjectPool.hpp:106
void setMaxSize(std::size_t max_size)
Definition: ObjectPool.hpp:139
T * operator()() const
Definition: ObjectPool.hpp:74
std::shared_ptr< ObjectType > SharedObjectPointer
Definition: ObjectPool.hpp:65
ObjectPool(std::size_t max_size=0)
Definition: ObjectPool.hpp:93
std::size_t getMaxSize() const
Definition: ObjectPool.hpp:134
Definition: ObjectPool.hpp:72
std::function< void(ObjectType *)> DestructorFunction
Definition: ObjectPool.hpp:68
std::function< void(ObjectType &)> ObjectFunction
Definition: ObjectPool.hpp:69
A data structure that caches instances of type T up to a user specified amount.
Definition: ObjectPool.hpp:60
ObjectPool(const ObjectPool &pool)
Definition: ObjectPool.hpp:89
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Definition: ObjectPool.hpp:81
T ObjectType
Definition: ObjectPool.hpp:63
The namespace of the Chemical Data Processing Library.
void setInitFunction(const ObjectFunction &func)
Definition: ObjectPool.hpp:153
void freeMemory()
Definition: ObjectPool.hpp:146
const unsigned int D
Specifies Hydrogen (Deuterium).
Definition: AtomType.hpp:62
void setCleanupFunction(const ObjectFunction &func)
Definition: ObjectPool.hpp:158
std::function< ObjectType *()> ConstructorFunction
Definition: ObjectPool.hpp:67
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92