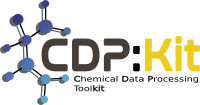 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_NOTMATCHEXPRESSION_HPP
30 #define CDPL_CHEM_NOTMATCHEXPRESSION_HPP
49 template <
typename ObjType1,
typename ObjType2 =
void>
64 expression(expr_ptr) {}
76 bool operator()(
const ObjType1& query_obj1,
const ObjType2& query_obj2,
const ObjType1& target_obj1,
const ObjType2& target_obj2,
91 bool operator()(
const ObjType1& query_obj1,
const ObjType2& query_obj2,
const ObjType1& target_obj1,
const ObjType2& target_obj2,
110 template <
typename ObjType>
125 expression(expr_ptr) {}
135 bool operator()(
const ObjType& query_obj,
const ObjType& target_obj,
const Base::Any& aux_data)
const;
166 template <
typename ObjType1,
typename ObjType2>
168 const ObjType1& target_obj1,
const ObjType2& target_obj2,
171 return !this->expression->operator()(query_obj1, query_obj2, target_obj1, target_obj2, data);
174 template <
typename ObjType1,
typename ObjType2>
176 const ObjType1& target_obj1,
const ObjType2& target_obj2,
179 return !this->expression->operator()(query_obj1, query_obj2, target_obj1, target_obj2, mapping, data);
182 template <
typename ObjType1,
typename ObjType2>
185 return this->expression->requiresAtomBondMapping();
189 template <
typename ObjType>
193 return !this->expression->operator()(query_obj, target_obj, data);
196 template <
typename ObjType>
200 return !this->expression->operator()(query_obj, target_obj, mapping, data);
203 template <
typename ObjType1>
206 return this->expression->requiresAtomBondMapping();
209 #endif // CDPL_CHEM_NOTMATCHEXPRESSION_HPP
NOTMatchExpression(const typename MatchExpression< ObjType1, ObjType2 >::SharedPointer &expr_ptr)
Constructs a NOTMatchExpression object that wraps the match expression instance specified by expr_ptr...
Definition: NOTMatchExpression.hpp:63
bool requiresAtomBondMapping() const
Tells whether the wrapped match expression requires a reevaluation after a query to target atom/bond ...
std::shared_ptr< NOTMatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated NOTMatchExpression instances.
Definition: NOTMatchExpression.hpp:118
NOTMatchExpression(const typename MatchExpression< ObjType, void >::SharedPointer &expr_ptr)
Constructs a NOTMatchExpressionBase object that wraps the match expression instance specified by expr...
Definition: NOTMatchExpression.hpp:124
bool requiresAtomBondMapping() const
Tells whether the wrapped match expression requires a reevaluation after a query to target atom/bond ...
Definition: NOTMatchExpression.hpp:183
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
NOTMatchExpression.
Definition: NOTMatchExpression.hpp:51
std::shared_ptr< MatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchExpression instances.
Definition: MatchExpression.hpp:81
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
bool operator()(const ObjType1 &query_obj1, const ObjType2 &query_obj2, const ObjType1 &target_obj1, const ObjType2 &target_obj2, const Base::Any &aux_data) const
Performs an evaluation of the wrapped match expression for the given query and target objects and ret...
Definition: NOTMatchExpression.hpp:167
std::shared_ptr< NOTMatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated NOTMatchExpression instances.
Definition: NOTMatchExpression.hpp:57