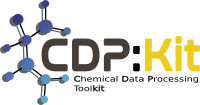 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_MULTIFORMATDATAWRITER_HPP
30 #define CDPL_UTIL_MULTIFORMATDATAWRITER_HPP
49 template <
typename DataType>
57 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::out |
58 std::ios_base::trunc | std::ios_base::binary);
61 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::out |
62 std::ios_base::trunc | std::ios_base::binary);
65 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::out |
66 std::ios_base::trunc | std::ios_base::binary);
82 operator const void*()
const;
100 template <
typename DataType>
103 for (std::string::size_type i = file_name.find_first_of(
'.', 0); i != std::string::npos; i = file_name.find_first_of(
'.', i)) {
109 writerPtr = handler->createWriter(file_name, mode);
110 dataFormat = handler->getDataFormat();
115 throw Base::IOError(
"MultiFormatDataWriter: could not deduce data format of '" + file_name +
"'");
120 template <
typename DataType>
122 std::ios_base::openmode mode)
127 throw Base::IOError(
"MultiFormatDataWriter: could not find handler for format '" + fmt +
"'");
129 writerPtr = handler->createWriter(file_name, mode);
130 dataFormat = handler->getDataFormat();
135 template <
typename DataType>
137 std::ios_base::openmode mode):
143 throw Base::IOError(
"MultiFormatDataWriter: could not find handler for format '" + fmt.
getName() +
"'");
145 writerPtr = handler->createWriter(file_name, mode);
150 template <
typename DataType>
156 throw Base::IOError(
"MultiFormatDataWriter: could not find handler for format '" + fmt +
"'");
158 writerPtr = handler->createWriter(ios);
159 dataFormat = handler->getDataFormat();
164 template <
typename DataType>
171 throw Base::IOError(
"MultiFormatDataWriter: could not find handler for format '" + fmt.
getName() +
"'");
173 writerPtr = handler->createWriter(ios);
178 template <
typename DataType>
184 template <
typename DataType>
188 writerPtr->
write(obj);
192 template <
typename DataType>
198 template <
typename DataType>
201 return writerPtr->operator
const void*();
204 template <
typename DataType>
207 return writerPtr->operator!();
210 template <
typename DataType>
217 #endif // CDPL_UTIL_MULTIFORMATDATAWRITER_HPP
Thrown to indicate that an I/O operation has failed because of physical (e.g. broken pipe) or logical...
Definition: Base/Exceptions.hpp:250
void invokeIOCallbacks(double progress) const
Invokes all registered I/O callback functions with the argument *this.
DataType DataType
The type of the written data objects.
Definition: DataWriter.hpp:74
A singleton class that serves as a global registry for Base::DataInputHandler and Base::DataOutputHan...
Definition: DataIOManager.hpp:104
static OutputHandlerPointer getOutputHandlerByFileExtension(const std::string &file_ext)
Returns a pointer to a Base::DataOutputHandler implementation instance registered for the data format...
Definition: DataIOManager.hpp:632
Definition of exception classes.
std::shared_ptr< DataWriter > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated DataWriter instances.
Definition: DataWriter.hpp:69
void setParent(const ControlParameterContainer *cntnr)
Sets or removes the parent control-parameter container used to resolve requests for missing entries.
The namespace of the Chemical Data Processing Library.
Definition of the class CDPL::Base::DataIOManager.
An interface for writing data objects of a given type to an arbitrary data sink.
Definition: DataWriter.hpp:63
static OutputHandlerPointer getOutputHandlerByFormat(const DataFormat &fmt)
Returns a pointer to a Base::DataOutputHandler implementation instance registered for the specified d...
Definition: DataIOManager.hpp:609