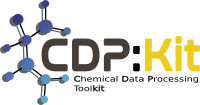 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_MULTIFORMATDATAREADER_HPP
30 #define CDPL_UTIL_MULTIFORMATDATAREADER_HPP
49 template <
typename DataType>
57 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::binary);
60 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::binary);
63 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::binary);
89 operator const void*()
const;
109 template <
typename DataType>
112 for (std::string::size_type i = file_name.find_first_of(
'.', 0); i != std::string::npos; i = file_name.find_first_of(
'.', i)) {
118 readerPtr = handler->createReader(file_name, mode);
119 dataFormat = handler->getDataFormat();
124 throw Base::IOError(
"MultiFormatDataReader: could not deduce data format of '" + file_name +
"'");
129 template <
typename DataType>
131 std::ios_base::openmode mode)
136 throw Base::IOError(
"MultiFormatDataReader: could not find handler for format '" + fmt +
"'");
138 readerPtr = handler->createReader(file_name, mode);
139 dataFormat = handler->getDataFormat();
144 template <
typename DataType>
146 std::ios_base::openmode mode):
152 throw Base::IOError(
"MultiFormatDataReader: could not find handler for format '" + fmt.
getName() +
"'");
154 readerPtr = handler->createReader(file_name, mode);
159 template <
typename DataType>
165 throw Base::IOError(
"MultiFormatDataReader: could not find handler for format '" + fmt +
"'");
167 readerPtr = handler->createReader(is);
168 dataFormat = handler->getDataFormat();
173 template <
typename DataType>
180 throw Base::IOError(
"MultiFormatDataReader: could not find handler for format '" + fmt.
getName() +
"'");
182 readerPtr = handler->createReader(is);
187 template <
typename DataType>
193 template <
typename DataType>
197 readerPtr->
read(obj, overwrite);
201 template <
typename DataType>
205 readerPtr->
read(idx, obj, overwrite);
209 template <
typename DataType>
217 template <
typename DataType>
220 return readerPtr->hasMoreData();
223 template <
typename DataType>
226 return readerPtr->getRecordIndex();
229 template <
typename DataType>
232 readerPtr->setRecordIndex(idx);
235 template <
typename DataType>
238 return readerPtr->getNumRecords();
241 template <
typename DataType>
244 return readerPtr->operator
const void*();
247 template <
typename DataType>
250 return readerPtr->operator!();
253 template <
typename DataType>
259 template <
typename DataType>
266 #endif // CDPL_UTIL_MULTIFORMATDATAREADER_HPP
static InputHandlerPointer getInputHandlerByFileExtension(const std::string &file_ext)
Returns a pointer to a Base::DataInputHandler implementation instance registered for the data format ...
Definition: DataIOManager.hpp:573
static InputHandlerPointer getInputHandlerByFormat(const DataFormat &fmt)
Returns a pointer to a Base::DataInputHandler implementation instance registered for the specified da...
Definition: DataIOManager.hpp:562
DataType DataType
The type of the read data objects.
Definition: DataReader.hpp:79
Thrown to indicate that an I/O operation has failed because of physical (e.g. broken pipe) or logical...
Definition: Base/Exceptions.hpp:250
void invokeIOCallbacks(double progress) const
Invokes all registered I/O callback functions with the argument *this.
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
A singleton class that serves as a global registry for Base::DataInputHandler and Base::DataOutputHan...
Definition: DataIOManager.hpp:104
Definition of exception classes.
std::shared_ptr< DataReader > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated DataReader instances.
Definition: DataReader.hpp:84
void setParent(const ControlParameterContainer *cntnr)
Sets or removes the parent control-parameter container used to resolve requests for missing entries.
The namespace of the Chemical Data Processing Library.
Definition of the class CDPL::Base::DataIOManager.