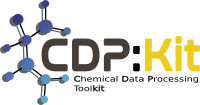 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
32 #ifndef CDPL_CHEM_MORGANNUMBERINGCALCULATOR_HPP
33 #define CDPL_CHEM_MORGANNUMBERINGCALCULATOR_HPP
90 typedef std::pair<std::size_t, std::size_t>
STPair;
93 typedef std::vector<std::size_t>
STArray;
94 typedef std::vector<long>
LArray;
95 typedef std::vector<std::string>
SArray;
101 NumberingState(STPairArray* sym_class_ids, SArray* symbols,
103 symClassIDs(sym_class_ids),
104 atomSymbols(symbols),
105 atomCharges(charges), atomIsotopes(isotopes), bondMatrix(bond_mtx) {}
112 void init(
const MolecularGraph&);
114 void perceiveSymClasses();
117 void distributeNumbers(std::size_t);
119 void getNextAtomIndices(
STArray&);
121 void addListEntriesForAtom(std::size_t, std::size_t);
123 void copy(NumberingState&);
125 typedef STPairArray::const_iterator STPairArrayIterator;
132 const MolecularGraph* molGraph;
137 std::string nodeValues;
140 STPairArrayIterator lastSymClass;
141 std::size_t centerAtomNumber;
142 std::size_t nextAtomNumber;
151 NumberingState numbering;
156 #endif // CDPL_CHEM_MORGANNUMBERINGCALCULATOR_HPP
Array< long > LArray
An array of unsigned integers of type long.
Definition: Array.hpp:572
Array< std::string > SArray
An array of std::string objects.
Definition: Array.hpp:592
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
MorganNumberingCalculator.
Definition: MorganNumberingCalculator.hpp:58
MolecularGraph.
Definition: MolecularGraph.hpp:52
MorganNumberingCalculator()
Constructs the MorganNumberingCalculator instance.
Definition of the class CDPL::Util::Array.
void calculate(const MolecularGraph &molgraph, Util::STArray &numbering)
Performs a canonical numbering of the atoms in the molecular graph molgraph.
Array< STPair > STPairArray
An array of pairs of unsigned integers of type std::size_t.
Definition: Array.hpp:582
The namespace of the Chemical Data Processing Library.
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
Definition of matrix data types.
MorganNumberingCalculator(const MolecularGraph &molgraph, Util::STArray &numbering)
Constructs the MorganNumberingCalculator instance and performs a canonical numbering of the atoms in ...
std::pair< std::size_t, std::size_t > STPair
A pair of unsigned integers of type std::size_t.
Definition: Array.hpp:577
SparseMatrix< unsigned long > SparseULMatrix
An unbounded sparse matrix holding unsigned integers of type unsigned long.
Definition: Matrix.hpp:1904