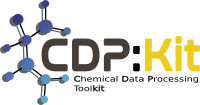 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_DESCR_MOLECULERDFDESCRIPTORCALCULATOR_HPP
30 #define CDPL_DESCR_MOLECULERDFDESCRIPTORCALCULATOR_HPP
179 #endif // CDPL_DESCR_MOLECULERDFDESCRIPTORCALCULATOR_HPP
std::function< const Math::Vector3D &(const EntityType &)> Entity3DCoordinatesFunction
Type of the generic functor class used to store a user-defined entity 3D coordinates function.
Definition: RDFCodeCalculator.hpp:74
MoleculeRDFDescriptorCalculator.
Definition: MoleculeRDFDescriptorCalculator.hpp:57
void setNumSteps(std::size_t num_steps)
Sets the number of desired radius incrementation steps.
void setRadiusIncrement(double radius_inc)
Sets the radius step size between successive RDF code elements.
MoleculeRDFDescriptorCalculator(const Chem::AtomContainer &cntnr, Math::DVector &descr)
double getStartRadius() const
Returns the starting value of the radius.
void setAtom3DCoordinatesFunction(const Atom3DCoordinatesFunction &func)
Allows to specify the atom coordinates function.
Atom.
Definition: Atom.hpp:52
Definition of the class CDPL::Descr::RDFCodeCalculator.
double getScalingFactor() const
Returns the scaling factor applied to the RDF code elements.
A common interface for data-structures that support a random access to stored Chem::Atom instances.
Definition: AtomContainer.hpp:55
double getRadiusIncrement() const
Returns the radius step size between successive RDF code elements.
RDFCodeCalculator::Entity3DCoordinatesFunction Atom3DCoordinatesFunction
Definition: MoleculeRDFDescriptorCalculator.hpp:62
std::function< double(const Chem::Atom &, const Chem::Atom &, unsigned int)> AtomPairWeightFunction
Definition: MoleculeRDFDescriptorCalculator.hpp:63
MoleculeRDFDescriptorCalculator()
Constructs the MoleculeRDFDescriptorCalculator instance.
The namespace of the Chemical Data Processing Library.
std::size_t getNumSteps() const
Returns the number of performed radius incrementation steps.
double getSmoothingFactor() const
Returns the smoothing factor used in the calculation of atom pair RDF contributions.
void enableDistanceToIntervalCenterRounding(bool enable)
Allows to specify whether atom pair distances should be rounded to the nearest radius interval center...
void setStartRadius(double start_radius)
Sets the starting value of the radius.
void setAtomPairWeightFunction(const AtomPairWeightFunction &func)
Allows to specify a custom atom pair weight function.
Definition of the preprocessor macro CDPL_DESCR_API.
bool distanceToIntervalsCenterRoundingEnabled() const
Tells whether atom pair distances get rounded to the nearest radius interval centers.
#define CDPL_DESCR_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setSmoothingFactor(double factor)
Allows to specify the smoothing factor used in the calculation of atom pair RDF contributions.
void calculate(const Chem::AtomContainer &cntnr, Math::DVector &descr)
Definition of vector data types.
void setScalingFactor(double factor)
Allows to specify the scaling factor for the RDF code elements.