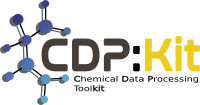 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
28 #ifndef CDPL_MATH_MINIMIZERVARIABLEARRAYTRAITS_HPP
29 #define CDPL_MATH_MINIMIZERVARIABLEARRAYTRAITS_HPP
71 for (
SizeType i = 0; i < size; i++) {
78 ssq = 1 + ssq * (scale / ax) * (scale / ax);
82 ssq += (ax / scale) * (ax / scale);
93 y.plusAssign(alpha * x);
106 template <
typename T>
118 template <
typename V>
127 template <
typename T>
138 template <
typename T>
145 const typename VectorType::ConstPointer vx = it->getData();
146 typename VectorType::SizeType dim = it->getSize();
148 for (
typename VectorType::SizeType i = 0; i < dim; i++) {
155 ssq = 1 + ssq * (scale / ax) * (scale / ax);
159 ssq += (ax / scale) * (ax / scale);
168 template <
typename T>
178 it2->plusAssign(tmp);
193 template <
typename T>
206 it1->minusAssign(*it2);
210 template <
typename V>
219 template <
typename T>
224 for (
typename ArrayType::const_iterator it1 = a1.begin(), it2 = a2.begin(), end1 = a1.end(); it1 != end1; ++it1, ++it2)
230 template <
typename T>
236 for (
typename ArrayType::const_iterator it = a.begin(), end = a.end(); it != end; ++it) {
237 const typename VectorType::ConstPointer vx = it->getData();
238 typename VectorType::SizeType dim = it->getSize();
240 for (
typename VectorType::SizeType i = 0; i < dim; i++) {
247 ssq = 1 + ssq * (scale / ax) * (scale / ax);
251 ssq += (ax / scale) * (ax / scale);
260 template <
typename T>
263 typename ArrayType::iterator it2 = y.begin();
266 for (
typename ArrayType::const_iterator it1 = x.begin(), end1 = x.end(); it1 != end1; ++it1, ++it2) {
270 it2->plusAssign(tmp);
276 for (
typename ArrayType::iterator it = a.begin(), end = a.end(); it != end; ++it)
285 template <
typename T>
288 for (
typename ArrayType::iterator it = a.begin(), end = a.end(); it != end; ++it)
295 typename ArrayType::const_iterator it2 = a2.begin();
297 for (
typename ArrayType::iterator it1 = a1.begin(), end1 = a1.end(); it1 != end1; ++it1, ++it2)
298 it1->minusAssign(*it2);
304 #endif // CDPL_MATH_MINIMIZERVARIABLEARRAYTRAITS_HPP
static void sub(ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:112
Definition: TypeTraits.hpp:171
Definition of the class CDPL::Math::VectorArray.
A::SizeType SizeType
Definition: MinimizerVariableArrayTraits.hpp:49
VectorInnerProduct< E1, E2 >::ResultType innerProd(const VectorExpression< E1 > &e1, const VectorExpression< E2 > &e2)
Definition: VectorExpression.hpp:504
std::size_t SizeType
The type of objects stored by the array.
Definition: Array.hpp:110
ConstElementIterator getElementsEnd() const
Returns a constant iterator pointing to the end of the array.
Definition: Array.hpp:896
static void assign(ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:101
static void axpy(const T &alpha, const ArrayType &x, ArrayType &y)
Definition: MinimizerVariableArrayTraits.hpp:91
static void multiply(ArrayType &a, const T &v)
Definition: MinimizerVariableArrayTraits.hpp:194
An array for storing generic vector objects.
Definition: VectorArray.hpp:49
V::ValueType ValueType
Definition: MinimizerVariableArrayTraits.hpp:216
static T norm2(const ArrayType &a)
Definition: MinimizerVariableArrayTraits.hpp:139
ConstElementIterator getElementsBegin() const
Returns a constant iterator pointing to the beginning of the array.
Definition: Array.hpp:884
static T norm2(const ArrayType &a)
Definition: MinimizerVariableArrayTraits.hpp:59
static T dot(const ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:220
Definition of type traits.
static void assign(ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:280
V VectorType
Definition: MinimizerVariableArrayTraits.hpp:215
ArrayType::SizeType SizeType
Definition: MinimizerVariableArrayTraits.hpp:125
StorageType::const_iterator ConstElementIterator
A constant random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:125
static void axpy(const T &alpha, const ArrayType &x, ArrayType &y)
Definition: MinimizerVariableArrayTraits.hpp:169
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
ArrayType::size_type SizeType
Definition: MinimizerVariableArrayTraits.hpp:217
Definition: MinimizerVariableArrayTraits.hpp:45
std::vector< V > ArrayType
Definition: MinimizerVariableArrayTraits.hpp:214
static T dot(const ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:128
The namespace of the Chemical Data Processing Library.
static void multiply(ArrayType &a, const T &v)
Definition: MinimizerVariableArrayTraits.hpp:286
static void clear(ArrayType &a)
Definition: MinimizerVariableArrayTraits.hpp:274
static void axpy(const T &alpha, const ArrayType &x, ArrayType &y)
Definition: MinimizerVariableArrayTraits.hpp:261
static void clear(ArrayType &a)
Definition: MinimizerVariableArrayTraits.hpp:96
static T dot(const ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:53
VectorArray< V > ArrayType
Definition: MinimizerVariableArrayTraits.hpp:122
static void multiply(ArrayType &a, const T &v)
Definition: MinimizerVariableArrayTraits.hpp:107
static T norm2(const ArrayType &a)
Definition: MinimizerVariableArrayTraits.hpp:231
static void assign(ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:188
A ArrayType
Definition: MinimizerVariableArrayTraits.hpp:47
StorageType::iterator ElementIterator
A mutable random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:137
V::ValueType ValueType
Definition: MinimizerVariableArrayTraits.hpp:124
static void clear(ArrayType &a)
Definition: MinimizerVariableArrayTraits.hpp:182
A::ValueType ValueType
Definition: MinimizerVariableArrayTraits.hpp:48
static void sub(ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:293
const unsigned int A
A generic type that covers any element except hydrogen.
Definition: AtomType.hpp:617
V VectorType
Definition: MinimizerVariableArrayTraits.hpp:123
static void sub(ArrayType &a1, const ArrayType &a2)
Definition: MinimizerVariableArrayTraits.hpp:201