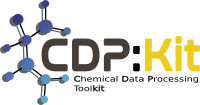 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_MAXCOMMONBONDSUBSTRUCTURESEARCH_HPP
30 #define CDPL_CHEM_MAXCOMMONBONDSUBSTRUCTURESEARCH_HPP
37 #include <boost/iterator/indirect_iterator.hpp>
63 typedef std::vector<AtomBondMapping*> ABMappingList;
71 typedef boost::indirect_iterator<ABMappingList::iterator, AtomBondMapping>
MappingIterator;
76 typedef boost::indirect_iterator<ABMappingList::const_iterator, const AtomBondMapping>
ConstMappingIterator;
271 void initMatchExpressions();
273 bool findEquivAtoms();
275 bool atomsCompatible(
const Bond&,
const Bond&)
const;
277 const Atom* getCommonAtom(
const Bond&,
const Bond&)
const;
279 bool buildAssocGraph();
281 bool findAssocGraphCliques(std::size_t);
282 bool isLegal(
const AGNode*);
284 void undoAtomMapping(std::size_t);
288 bool hasPostMappingMatchExprs()
const;
291 bool foundMappingUnique(
bool);
293 void clearMappings();
296 void freeAtomBondMapping();
298 void freeAssocGraph();
299 void freeAtomBondMappings();
303 AGNode* allocAGNode(
const Bond*,
const Bond*);
304 AGEdge* allocAGEdge(
const Atom*,
const Atom*);
306 typedef std::vector<const AGEdge*> AGraphEdgeList;
312 void setQueryBond(
const Bond*);
313 const Bond* getQueryBond()
const;
315 void setAssocBond(
const Bond*);
316 const Bond* getAssocBond()
const;
318 void addEdge(
const AGEdge*);
320 bool isConnected(
const AGNode*)
const;
321 const AGEdge* findEdge(
const AGNode*)
const;
325 void setIndex(std::size_t idx);
329 const Bond* queryBond;
330 const Bond* assocBond;
332 AGraphEdgeList atomEdges;
339 void setQueryAtom(
const Atom*);
340 const Atom* getQueryAtom()
const;
342 void setAssocAtom(
const Atom*);
343 const Atom* getAssocAtom()
const;
345 void setNode1(
const AGNode*);
346 void setNode2(
const AGNode*);
348 const AGNode* getNode1()
const;
349 const AGNode* getNode2()
const;
351 const AGNode* getOther(
const AGNode*)
const;
354 const Atom* queryAtom;
355 const Atom* assocAtom;
364 void initQueryAtomMask(std::size_t);
365 void initTargetAtomMask(std::size_t);
367 void initQueryBondMask(std::size_t);
368 void initTargetBondMask(std::size_t);
370 void setQueryAtomBit(std::size_t);
371 void setTargetAtomBit(std::size_t);
373 void resetQueryAtomBit(std::size_t);
374 void resetTargetAtomBit(std::size_t);
376 bool testQueryAtomBit(std::size_t)
const;
377 bool testTargetAtomBit(std::size_t)
const;
379 void setQueryBondBit(std::size_t);
380 void setTargetBondBit(std::size_t);
382 void resetQueryAtomBits();
383 void resetTargetAtomBits();
385 void resetBondBits();
387 bool operator<(
const ABMappingMask&)
const;
388 bool operator>(
const ABMappingMask&)
const;
399 typedef std::vector<Util::BitSet> BitMatrix;
400 typedef std::vector<AGNode*> AGraphNodeList;
401 typedef std::vector<AGraphNodeList> AGraphNodeMatrix;
402 typedef std::set<ABMappingMask> UniqueMappingList;
403 typedef std::vector<const Atom*> AtomList;
404 typedef std::vector<const Bond*> BondList;
405 typedef std::vector<MatchExpression<Atom, MolecularGraph>::SharedPointer> AtomMatchExprTable;
406 typedef std::vector<MatchExpression<Bond, MolecularGraph>::SharedPointer> BondMatchExprTable;
407 typedef Util::ObjectStack<AGNode> NodeCache;
408 typedef Util::ObjectStack<AGEdge> EdgeCache;
409 typedef Util::ObjectStack<AtomBondMapping> MappingCache;
411 const MolecularGraph* query;
412 const MolecularGraph* target;
413 BitMatrix atomEquivMatrix;
414 AGraphNodeMatrix nodeMatrix;
415 ABMappingList foundMappings;
416 UniqueMappingList uniqueMappings;
417 AGraphEdgeList cliqueEdges;
418 AGraphNodeList cliqueNodes;
419 ABMappingMask mappingMask;
420 AtomMatchExprTable atomMatchExprTable;
421 BondMatchExprTable bondMatchExprTable;
422 MolGraphMatchExprPtr molGraphMatchExpr;
423 AtomList postMappingMatchAtoms;
424 BondList postMappingMatchBonds;
427 MappingCache mappingCache;
432 std::size_t numQueryAtoms;
433 std::size_t numQueryBonds;
434 std::size_t numTargetAtoms;
435 std::size_t numTargetBonds;
436 std::size_t maxBondSubstructureSize;
437 std::size_t currNumNullNodes;
438 std::size_t minNumNullNodes;
439 std::size_t maxNumMappings;
440 std::size_t minSubstructureSize;
441 std::size_t currNodeIdx;
446 #endif // CDPL_CHEM_MAXCOMMONBONDSUBSTRUCTURESEARCH_HPP
std::size_t getMinSubstructureSize() const
Returns the minimum accepted common substructure size.
void uniqueMappingsOnly(bool unique)
Allows to specify whether or not to store only unique atom/bond mappings.
Definition of the class CDPL::Util::ObjectStack.
MaxCommonBondSubstructureSearch.
Definition: MaxCommonBondSubstructureSearch.hpp:61
MaxCommonBondSubstructureSearch(const MolecularGraph &query)
Constructs and initializes a MaxCommonBondSubstructureSearch instance for the specified query structu...
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
MaxCommonBondSubstructureSearch()
Constructs and initializes a MaxCommonBondSubstructureSearch instance.
MappingIterator end()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
bool mappingExists(const MolecularGraph &target)
Searches for a common substructure between the query and the specified target molecular graph.
Atom.
Definition: Atom.hpp:52
std::size_t getNumMappings() const
Returns the number of atom/bond mappings that were recorded in the last search for common substructur...
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: MaxCommonBondSubstructureSearch.hpp:71
const AtomBondMapping & getMapping(std::size_t idx) const
Returns a const reference to the stored atom/bond mapping object at index idx.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: MaxCommonBondSubstructureSearch.hpp:76
MappingIterator getMappingsBegin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
ConstMappingIterator end() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
bool findMappings(const MolecularGraph &target)
Searches for all atom/bond mappings of query subgraphs to substructures of the specified target molec...
std::shared_ptr< MatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchExpression instances.
Definition: MatchExpression.hpp:81
Definition of the class CDPL::Chem::AtomBondMapping.
void setMaxNumMappings(std::size_t max_num_mappings)
Allows to specify a limit on the number of stored atom/bond mappings.
MappingIterator begin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
void setMinSubstructureSize(std::size_t min_size)
Allows to specify the minimum accepted common substructure size.
ConstMappingIterator begin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
MappingIterator getMappingsEnd()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
bool uniqueMappingsOnly() const
Tells whether duplicate atom/bond mappings are discarded.
~MaxCommonBondSubstructureSearch()
Destructor.
ConstMappingIterator getMappingsEnd() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
std::shared_ptr< MaxCommonBondSubstructureSearch > SharedPointer
Definition: MaxCommonBondSubstructureSearch.hpp:66
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
std::size_t getMaxNumMappings() const
Returns the specified limit on the number of stored atom/bond mappings.
void setQuery(const MolecularGraph &query)
Allows to specify a new query structure.
AtomBondMapping & getMapping(std::size_t idx)
Returns a non-const reference to the stored atom/bond mapping object at index idx.
ConstMappingIterator getMappingsBegin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.