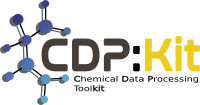 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_MATRIXPROXY_HPP
28 #define CDPL_MATH_MATRIXPROXY_HPP
30 #include <type_traits>
59 typedef typename std::conditional<std::is_const<M>::value,
60 typename M::ConstReference,
62 typedef typename std::conditional<std::is_const<M>::value,
63 typename M::ConstClosureType,
73 return data(index, i);
78 return data(index, i);
83 return data(index, i);
88 return data(index, i);
98 return data.getSize2();
103 return (data.getSize2() == 0);
122 template <
typename E>
129 template <
typename E>
136 template <
typename E>
143 template <
typename T>
146 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
150 template <
typename T>
153 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
157 template <
typename E>
160 vectorAssignVector<ScalarAssignment>(*
this, e);
164 template <
typename E>
167 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
171 template <
typename E>
174 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
194 template <
typename M>
206 typedef typename std::conditional<std::is_const<M>::value,
207 typename M::ConstReference,
209 typedef typename std::conditional<std::is_const<M>::value,
210 typename M::ConstClosureType,
220 return data(i, index);
225 return data(i, index);
230 return data(i, index);
235 return data(i, index);
245 return data.getSize1();
250 return (data.getSize1() == 0);
269 template <
typename E>
276 template <
typename E>
283 template <
typename E>
290 template <
typename T>
293 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
297 template <
typename T>
300 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
304 template <
typename E>
307 vectorAssignVector<ScalarAssignment>(*
this, e);
311 template <
typename E>
314 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
318 template <
typename E>
321 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
341 template <
typename M>
353 typedef typename std::conditional<std::is_const<M>::value,
354 typename M::ConstReference,
356 typedef typename std::conditional<std::is_const<M>::value,
357 typename M::ConstClosureType,
364 data(
m), range1(r1), range2(r2) {}
368 return data(range1(i), range2(j));
373 return data(range1(i), range2(j));
417 template <
typename E>
424 template <
typename E>
431 template <
typename E>
438 template <
typename T>
441 matrixAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
445 template <
typename T>
448 matrixAssignScalar<ScalarDivisionAssignment>(*
this, t);
452 template <
typename E>
455 matrixAssignMatrix<ScalarAssignment>(*
this, e);
459 template <
typename E>
462 matrixAssignMatrix<ScalarAdditionAssignment>(*
this, e);
466 template <
typename E>
469 matrixAssignMatrix<ScalarSubtractionAssignment>(*
this, e);
490 template <
typename M>
502 typedef typename std::conditional<std::is_const<M>::value,
503 typename M::ConstReference,
505 typedef typename std::conditional<std::is_const<M>::value,
506 typename M::ConstClosureType,
513 data(
m), slice1(s1), slice2(s2) {}
517 return data(slice1(i), slice2(j));
522 return data(slice1(i), slice2(j));
576 template <
typename E>
583 template <
typename E>
590 template <
typename E>
597 template <
typename T>
600 matrixAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
604 template <
typename T>
607 matrixAssignScalar<ScalarDivisionAssignment>(*
this, t);
611 template <
typename E>
614 matrixAssignMatrix<ScalarAssignment>(*
this, e);
618 template <
typename E>
621 matrixAssignMatrix<ScalarAdditionAssignment>(*
this, e);
625 template <
typename E>
628 matrixAssignMatrix<ScalarSubtractionAssignment>(*
this, e);
649 template <
typename M>
653 template <
typename M>
657 template <
typename M>
661 template <
typename M>
665 template <
typename M>
669 template <
typename M>
673 template <
typename M>
677 template <
typename M>
681 template <
typename M>
685 template <
typename M>
689 template <
typename M>
693 template <
typename M>
697 template <
typename M>
701 template <
typename M>
705 template <
typename M>
709 template <
typename M>
713 template <
typename M>
721 template <
typename M>
728 template <
typename M>
735 template <
typename M>
736 MatrixColumn<const M>
742 template <
typename E>
751 template <
typename E>
760 template <
typename E>
770 return MatrixRange<E>(e(), RangeType(start1, stop1), RangeType(start2, stop2));
773 template <
typename E>
786 template <
typename E>
795 template <
typename E>
804 template <
typename E>
816 return MatrixSlice<E>(e(), SliceType(start1, stride1, size1), SliceType(start2, stride2, size2));
819 template <
typename E>
831 return MatrixSlice<const E>(e(), SliceType(start1, stride1, size1), SliceType(start2, stride2, size2));
836 #endif // CDPL_MATH_MATRIXPROXY_HPP
ConstReference operator()(SizeType i) const
Definition: MatrixProxy.hpp:223
MatrixSlice & operator=(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:577
MatrixRow & plusAssign(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:165
MatrixSlice & operator-=(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:591
Definition: TypeTraits.hpp:179
friend void swap(MatrixColumn &c1, MatrixColumn &c2)
Definition: MatrixProxy.hpp:331
MatrixClosureType & getData()
Definition: MatrixProxy.hpp:253
void vectorSwap(V &v, VectorExpression< E > &e)
Definition: VectorAssignment.hpp:72
Implementation of vector assignment routines.
const MatrixClosureType & getData() const
Definition: MatrixProxy.hpp:565
void swap(MatrixSlice &s)
Definition: MatrixProxy.hpp:632
MatrixColumn & assign(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:305
MatrixClosureType & getData()
Definition: MatrixProxy.hpp:560
bool isEmpty() const
Definition: MatrixProxy.hpp:101
SizeType getSize() const
Definition: MatrixProxy.hpp:243
DifferenceType getStride2() const
Definition: MatrixProxy.hpp:540
ConstReference operator[](SizeType i) const
Definition: MatrixProxy.hpp:86
MatrixRange & operator=(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:418
std::conditional< std::is_const< M >::value, typename M::ConstReference, typename M::Reference >::type Reference
Definition: MatrixProxy.hpp:504
const MatrixClosureType & getData() const
Definition: MatrixProxy.hpp:258
std::conditional< std::is_const< M >::value, typename M::ConstClosureType, typename M::ClosureType >::type MatrixClosureType
Definition: MatrixProxy.hpp:507
MatrixRange & operator-=(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:432
M::SizeType SizeType
Definition: MatrixProxy.hpp:349
MatrixColumn< M > column(MatrixExpression< M > &e, typename MatrixColumn< M >::SizeType j)
Definition: MatrixProxy.hpp:730
std::conditional< std::is_const< M >::value, typename M::ConstReference, typename M::Reference >::type Reference
Definition: MatrixProxy.hpp:355
Definition: Expression.hpp:54
const MatrixClosureType & getData() const
Definition: MatrixProxy.hpp:406
SelfType ClosureType
Definition: MatrixProxy.hpp:66
MatrixRange & operator=(const MatrixRange &r)
Definition: MatrixProxy.hpp:411
SizeType getStart2() const
Definition: MatrixProxy.hpp:381
MatrixColumn & minusAssign(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:319
std::enable_if< IsScalar< T >::value, MatrixSlice >::type & operator*=(const T &t)
Definition: MatrixProxy.hpp:598
Reference operator()(SizeType i)
Definition: MatrixProxy.hpp:218
MatrixColumn & operator+=(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:277
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixProxy.hpp:520
MatrixSlice & operator+=(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:584
MatrixSlice(MatrixType &m, const SliceType &s1, const SliceType &s2)
Definition: MatrixProxy.hpp:512
const SelfType ConstClosureType
Definition: MatrixProxy.hpp:508
Slice< SizeType, DifferenceType > SliceType
Definition: MatrixProxy.hpp:510
MatrixSlice & plusAssign(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:619
M::SizeType SizeType
Definition: MatrixProxy.hpp:55
Range< SizeType > RangeType
Definition: MatrixProxy.hpp:361
SizeType getStart1() const
Definition: MatrixProxy.hpp:525
M::ConstReference ConstReference
Definition: MatrixProxy.hpp:352
M MatrixType
Definition: MatrixProxy.hpp:201
Definition: Expression.hpp:76
MatrixSlice< E > slice(MatrixExpression< E > &e, const typename MatrixSlice< E >::SliceType &s1, const typename MatrixSlice< E >::SliceType &s2)
Definition: MatrixProxy.hpp:788
std::enable_if< IsScalar< T >::value, MatrixRange >::type & operator*=(const T &t)
Definition: MatrixProxy.hpp:439
MatrixRow & assign(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:158
MatrixColumn & operator=(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:270
DifferenceType getStride1() const
Definition: MatrixProxy.hpp:535
MatrixRow & minusAssign(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:172
M::SizeType SizeType
Definition: MatrixProxy.hpp:202
std::conditional< std::is_const< M >::value, typename M::ConstClosureType, typename M::ClosureType >::type MatrixClosureType
Definition: MatrixProxy.hpp:211
MatrixSlice & assign(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:612
MatrixRange & minusAssign(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:467
SizeType getStart2() const
Definition: MatrixProxy.hpp:530
Reference operator[](SizeType i)
Definition: MatrixProxy.hpp:81
const SelfType ConstClosureType
Definition: MatrixProxy.hpp:65
Reference operator()(SizeType i, SizeType j)
Definition: MatrixProxy.hpp:366
SelfType ClosureType
Definition: MatrixProxy.hpp:213
M::ValueType ValueType
Definition: MatrixProxy.hpp:500
MatrixRange & assign(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:453
MatrixColumn(MatrixType &m, SizeType i)
Definition: MatrixProxy.hpp:215
MatrixRange(MatrixType &m, const RangeType &r1, const RangeType &r2)
Definition: MatrixProxy.hpp:363
Reference operator()(SizeType i, SizeType j)
Definition: MatrixProxy.hpp:515
MatrixColumn & operator=(const MatrixColumn &c)
Definition: MatrixProxy.hpp:263
std::enable_if< IsScalar< T >::value, MatrixRow >::type & operator*=(const T &t)
Definition: MatrixProxy.hpp:144
bool isEmpty() const
Definition: MatrixProxy.hpp:555
M::ConstReference ConstReference
Definition: MatrixProxy.hpp:205
const MatrixClosureType & getData() const
Definition: MatrixProxy.hpp:111
Definition: MatrixProxy.hpp:49
Definition: MatrixProxy.hpp:196
SizeType SizeType
Definition: Range.hpp:50
const unsigned int M
A generic type that covers any element that is a metal.
Definition: AtomType.hpp:637
M MatrixType
Definition: MatrixProxy.hpp:497
SizeType getSize2() const
Definition: MatrixProxy.hpp:391
Definition of type traits.
MatrixRow(MatrixType &m, SizeType i)
Definition: MatrixProxy.hpp:68
ConstReference operator()(SizeType i) const
Definition: MatrixProxy.hpp:76
MatrixRange & operator+=(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:425
M::ValueType ValueType
Definition: MatrixProxy.hpp:57
M::DifferenceType DifferenceType
Definition: MatrixProxy.hpp:56
std::enable_if< IsScalar< T >::value, MatrixColumn >::type & operator/=(const T &t)
Definition: MatrixProxy.hpp:298
M::ConstReference ConstReference
Definition: MatrixProxy.hpp:501
Definition of various functors.
M::MatrixTemporaryType Type
Definition: TypeTraits.hpp:188
M::DifferenceType DifferenceType
Definition: MatrixProxy.hpp:203
std::conditional< std::is_const< M >::value, typename M::ConstReference, typename M::Reference >::type Reference
Definition: MatrixProxy.hpp:61
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
Definition: TypeTraits.hpp:186
Implementation of matrix assignment routines.
std::enable_if< IsScalar< T >::value, MatrixRow >::type & operator/=(const T &t)
Definition: MatrixProxy.hpp:151
ConstReference operator[](SizeType i) const
Definition: MatrixProxy.hpp:233
MatrixSlice & minusAssign(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:626
std::conditional< std::is_const< M >::value, typename M::ConstReference, typename M::Reference >::type Reference
Definition: MatrixProxy.hpp:208
Reference operator()(SizeType i)
Definition: MatrixProxy.hpp:71
SelfType ClosureType
Definition: MatrixProxy.hpp:509
M MatrixType
Definition: MatrixProxy.hpp:348
SizeType SizeType
Definition: Slice.hpp:50
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
M::DifferenceType DifferenceType
Definition: MatrixProxy.hpp:350
const unsigned int s
Specifies that the stereocenter has s configuration.
Definition: CIPDescriptor.hpp:81
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
DifferenceType getStride() const
Definition: Slice.hpp:73
SizeType getStart() const
Definition: Slice.hpp:68
SizeType getIndex() const
Definition: MatrixProxy.hpp:238
void swap(MatrixColumn &c)
Definition: MatrixProxy.hpp:325
The namespace of the Chemical Data Processing Library.
MatrixRow & operator=(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:123
DifferenceType DifferenceType
Definition: Slice.hpp:51
M::ValueType ValueType
Definition: MatrixProxy.hpp:204
std::enable_if< IsScalar< T >::value, MatrixColumn >::type & operator*=(const T &t)
Definition: MatrixProxy.hpp:291
const SelfType ConstClosureType
Definition: MatrixProxy.hpp:212
SizeType getSize2() const
Definition: MatrixProxy.hpp:550
M::ConstReference ConstReference
Definition: MatrixProxy.hpp:58
SizeType getSize1() const
Definition: MatrixProxy.hpp:545
M::SizeType SizeType
Definition: MatrixProxy.hpp:498
const SelfType ConstClosureType
Definition: MatrixProxy.hpp:359
SelfType ClosureType
Definition: MatrixProxy.hpp:360
friend void swap(MatrixRange &r1, MatrixRange &r2)
Definition: MatrixProxy.hpp:479
MatrixColumn & operator-=(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:284
void swap(MatrixRange &r)
Definition: MatrixProxy.hpp:473
std::enable_if< IsScalar< T >::value, MatrixSlice >::type & operator/=(const T &t)
Definition: MatrixProxy.hpp:605
MatrixRow< M > row(MatrixExpression< M > &e, typename MatrixRow< M >::SizeType i)
Definition: MatrixProxy.hpp:716
bool isEmpty() const
Definition: MatrixProxy.hpp:396
std::conditional< std::is_const< M >::value, typename M::ConstClosureType, typename M::ClosureType >::type MatrixClosureType
Definition: MatrixProxy.hpp:64
M::ValueType ValueType
Definition: MatrixProxy.hpp:351
SizeType getIndex() const
Definition: MatrixProxy.hpp:91
SizeType getSize() const
Definition: Range.hpp:77
Definition of a data type for describing index slices.
MatrixSlice & operator=(const MatrixSlice &s)
Definition: MatrixProxy.hpp:570
friend void swap(MatrixSlice &s1, MatrixSlice &s2)
Definition: MatrixProxy.hpp:638
void swap(MatrixRow &r)
Definition: MatrixProxy.hpp:178
MatrixClosureType & getData()
Definition: MatrixProxy.hpp:106
void matrixSwap(M &m, MatrixExpression< E > &e)
Definition: MatrixAssignment.hpp:76
Reference operator[](SizeType i)
Definition: MatrixProxy.hpp:228
MatrixRow & operator=(const MatrixRow &r)
Definition: MatrixProxy.hpp:116
friend void swap(MatrixRow &r1, MatrixRow &r2)
Definition: MatrixProxy.hpp:184
Definition of basic expression types.
MatrixRange< E > range(MatrixExpression< E > &e, const typename MatrixRange< E >::RangeType &r1, const typename MatrixRange< E >::RangeType &r2)
Definition: MatrixProxy.hpp:744
M::DifferenceType DifferenceType
Definition: MatrixProxy.hpp:499
SizeType getSize1() const
Definition: MatrixProxy.hpp:386
MatrixRow & operator+=(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:130
SizeType getSize() const
Definition: MatrixProxy.hpp:96
Definition of a data type for describing index ranges.
Definition: MatrixProxy.hpp:492
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixProxy.hpp:371
SizeType getSize() const
Definition: Slice.hpp:78
MatrixRow & operator-=(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:137
MatrixColumn & plusAssign(const VectorExpression< E > &e)
Definition: MatrixProxy.hpp:312
std::enable_if< IsScalar< T >::value, MatrixRange >::type & operator/=(const T &t)
Definition: MatrixProxy.hpp:446
MatrixRange & plusAssign(const MatrixExpression< E > &e)
Definition: MatrixProxy.hpp:460
M MatrixType
Definition: MatrixProxy.hpp:54
std::conditional< std::is_const< M >::value, typename M::ConstClosureType, typename M::ClosureType >::type MatrixClosureType
Definition: MatrixProxy.hpp:358
SizeType getStart1() const
Definition: MatrixProxy.hpp:376
Definition: MatrixProxy.hpp:343
bool isEmpty() const
Definition: MatrixProxy.hpp:248
MatrixClosureType & getData()
Definition: MatrixProxy.hpp:401
SizeType getStart() const
Definition: Range.hpp:67