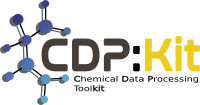 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_MATRIXEXPRESSION_HPP
28 #define CDPL_MATH_MATRIXEXPRESSION_HPP
30 #include <type_traits>
47 class VectorContainer;
49 class MatrixContainer;
51 template <
typename E,
typename F>
56 typedef F FunctorType;
57 typedef E ExpressionType;
58 typedef typename E::ConstClosureType ExpressionClosureType;
74 return expr.getSize1();
79 return expr.getSize2();
84 return FunctorType::apply(expr(i, j));
88 ExpressionClosureType expr;
91 template <
typename E,
typename F>
99 template <
typename E,
typename F>
104 typedef F FunctorType;
105 typedef E ExpressionType;
106 typedef typename E::ConstClosureType ExpressionClosureType;
122 return expr.getSize();
127 return expr.getSize();
132 return FunctorType::apply(expr, i, j);
136 ExpressionClosureType expr;
139 template <
typename E,
typename F>
147 template <
typename E1,
typename E2,
typename F>
152 typedef F FunctorType;
153 typedef E1 Expression1Type;
154 typedef E2 Expression2Type;
155 typedef typename E1::ConstClosureType Expression1ClosureType;
156 typedef typename E2::ConstClosureType Expression2ClosureType;
168 expr1(e1), expr2(e2) {}
182 return FunctorType::apply(expr1(i, j), expr2(i, j));
186 Expression1ClosureType expr1;
187 Expression2ClosureType expr2;
190 template <
typename E1,
typename E2,
typename F>
198 template <
typename E1,
typename E2,
typename F>
203 typedef F FunctorType;
204 typedef E1 Expression1Type;
205 typedef E2 Expression2Type;
206 typedef typename E1::ConstClosureType Expression1ClosureType;
207 typedef typename E2::ConstClosureType Expression2ClosureType;
219 expr1(e1), expr2(e2) {}
223 return expr1.getSize1();
228 return expr2.getSize2();
233 return FunctorType::apply(expr1, expr2, i, j);
237 Expression1ClosureType expr1;
238 Expression2ClosureType expr2;
241 template <
typename E1,
typename E2,
typename F>
249 template <
typename E1,
typename E2,
typename F>
254 typedef F FunctorType;
255 typedef E1 Expression1Type;
256 typedef E2 Expression2Type;
257 typedef typename E1::ConstClosureType Expression1ClosureType;
258 typedef typename E2::ConstClosureType Expression2ClosureType;
270 expr1(e1), expr2(e2) {}
274 return expr1.getSize();
279 return expr2.getSize();
284 return FunctorType::apply(expr1(i), expr2(j));
288 Expression1ClosureType expr1;
289 Expression2ClosureType expr2;
292 template <
typename E1,
typename E2,
typename F>
300 template <
typename E1,
typename E2,
typename F>
305 typedef F FunctorType;
306 typedef E1 Expression1Type;
307 typedef E2 Expression2Type;
308 typedef typename E1::ConstClosureType Expression1ClosureType;
309 typedef typename E2::ConstClosureType Expression2ClosureType;
321 expr1(e1), expr2(e2) {}
325 return expr1.getSize1();
330 return FunctorType::apply(expr1, expr2, i);
335 return FunctorType::apply(expr1, expr2, i);
339 Expression1ClosureType expr1;
340 Expression2ClosureType expr2;
343 template <
typename E1,
typename E2,
typename F>
351 template <
typename E1,
typename E2,
typename F>
356 typedef F FunctorType;
357 typedef E1 Expression1Type;
358 typedef E2 Expression2Type;
359 typedef typename E1::ConstClosureType Expression1ClosureType;
360 typedef typename E2::ConstClosureType Expression2ClosureType;
372 expr1(e1), expr2(e2) {}
376 return expr2.getSize2();
381 return FunctorType::apply(expr1, expr2, i);
386 return FunctorType::apply(expr1, expr2, i);
390 Expression1ClosureType expr1;
391 Expression2ClosureType expr2;
394 template <
typename E1,
typename E2,
typename F>
402 template <
typename E1,
typename E2,
typename F>
407 typedef F FunctorType;
408 typedef E1 Expression1Type;
409 typedef E2 Expression2Type;
410 typedef const E1 Expression1ClosureType;
411 typedef typename E2::ConstClosureType Expression2ClosureType;
423 expr1(e1), expr2(e2) {}
427 return expr2.getSize1();
432 return expr2.getSize2();
437 return FunctorType::apply(expr1, expr2(i, j));
441 Expression1ClosureType expr1;
442 Expression2ClosureType expr2;
445 template <
typename E1,
typename E2,
typename F>
453 template <
typename E1,
typename E2,
typename F>
458 typedef F FunctorType;
459 typedef E1 Expression1Type;
460 typedef E2 Expression2Type;
461 typedef typename E1::ConstClosureType Expression1ClosureType;
462 typedef const E2 Expression2ClosureType;
474 expr1(e1), expr2(e2) {}
478 return expr1.getSize1();
483 return expr1.getSize2();
488 return FunctorType::apply(expr1(i, j), expr2);
492 Expression1ClosureType expr1;
493 Expression2ClosureType expr2;
496 template <
typename E1,
typename E2,
typename F>
504 template <
typename M>
516 typedef typename std::conditional<std::is_const<M>::value,
517 typename M::ConstReference,
519 typedef typename std::conditional<std::is_const<M>::value,
520 typename M::ConstClosureType,
540 return data.getSize2();
545 return data.getSize1();
550 return data.getMaxSize();
555 return (data.getSize1() == 0 || data.getSize2() == 0);
570 data.operator=(mt.data);
574 template <
typename M1>
581 template <
typename E>
588 template <
typename E>
595 template <
typename E>
602 template <
typename T>
610 template <
typename T>
618 template <
typename E>
625 template <
typename E>
632 template <
typename E>
653 template <
typename M>
657 template <
typename M>
661 template <
typename M>
665 template <
typename M>
669 template <
typename E>
675 return ExpressionType(e());
678 template <
typename E>
685 template <
typename E1,
typename E2>
686 typename MatrixBinary1Traits<E1, E2, ScalarAddition<typename E1::ValueType, typename E2::ValueType> >::ResultType
692 return ExpressionType(e1(), e2());
695 template <
typename E1,
typename E2>
696 typename MatrixBinary1Traits<E1, E2, ScalarSubtraction<typename E1::ValueType, typename E2::ValueType> >::ResultType
702 return ExpressionType(e1(), e2());
705 template <
typename E,
typename T>
706 typename std::enable_if<IsScalar<T>::value,
typename Scalar2MatrixBinaryTraits<E, T, ScalarMultiplication<typename E::ValueType, T> >::ResultType>::type
712 return ExpressionType(e(), t);
715 template <
typename T,
typename E>
716 typename std::enable_if<IsScalar<T>::value,
typename Scalar1MatrixBinaryTraits<T, E, ScalarMultiplication<T, typename E::ValueType> >::ResultType>::type
722 return ExpressionType(t, e());
725 template <
typename E,
typename T>
726 typename std::enable_if<IsScalar<T>::value,
typename Scalar2MatrixBinaryTraits<E, T, ScalarDivision<typename E::ValueType, T> >::ResultType>::type
732 return ExpressionType(e(), t);
735 template <
typename E1,
typename E2>
742 template <
typename E1,
typename E2>
749 template <
typename E1,
typename E2,
typename T>
756 template <
typename E>
757 typename MatrixUnaryTraits<E, ScalarConjugation<typename E::ValueType> >::ResultType
762 return ExpressionType(e());
765 template <
typename E>
766 typename MatrixUnaryTraits<E, ScalarConjugation<typename E::ValueType> >::ResultType
771 return ExpressionType(e());
774 template <
typename E>
775 typename MatrixUnaryTraits<E, ScalarReal<typename E::ValueType> >::ResultType
780 return ExpressionType(e());
783 template <
typename E>
784 typename MatrixUnaryTraits<E, ScalarImaginary<typename E::ValueType> >::ResultType
789 return ExpressionType(e());
792 template <
typename E1,
typename E2>
793 typename VectorMatrixBinaryTraits<E1, E2, ScalarMultiplication<typename E1::ValueType, typename E2::ValueType> >::ResultType
799 return ExpressionType(e1(), e2());
802 template <
typename E1,
typename E2>
803 typename MatrixBinary1Traits<E1, E2, ScalarDivision<typename E1::ValueType, typename E2::ValueType> >::ResultType
809 return ExpressionType(e1(), e2());
812 template <
typename E1,
typename E2>
813 typename MatrixBinary1Traits<E1, E2, ScalarMultiplication<typename E1::ValueType, typename E2::ValueType> >::ResultType
819 return ExpressionType(e1(), e2());
822 template <
typename E1,
typename E2>
823 typename Matrix1VectorBinaryTraits<E1, E2, MatrixVectorProduct<E1, E2> >::ResultType
828 return ExpressionType(e1(), e2());
831 template <
typename E1,
typename E2>
832 typename Matrix1VectorBinaryTraits<E1, E2, MatrixVectorProduct<E1, E2> >::ResultType
837 return ExpressionType(e1(), e2());
840 template <
typename C,
typename E1,
typename E2>
843 return c().assign(
prod(e1, e2));
846 template <
typename E1,
typename E2>
847 typename Matrix2VectorBinaryTraits<E1, E2, VectorMatrixProduct<E1, E2> >::ResultType
852 return ExpressionType(e1(), e2());
855 template <
typename E1,
typename E2>
856 typename Matrix2VectorBinaryTraits<E1, E2, VectorMatrixProduct<E1, E2> >::ResultType
861 return ExpressionType(e1(), e2());
864 template <
typename C,
typename E1,
typename E2>
867 return c().assign(
prod(e1, e2));
870 template <
typename E1,
typename E2>
871 typename MatrixBinary2Traits<E1, E2, MatrixProduct<E1, E2> >::ResultType
876 return ExpressionType(e1(), e2());
879 template <
typename E1,
typename E2>
880 typename MatrixBinary2Traits<E1, E2, MatrixProduct<E1, E2> >::ResultType
885 return ExpressionType(e1(), e2());
888 template <
typename C,
typename E1,
typename E2>
891 return c().assign(
prod(e1, e2));
894 template <
typename E>
901 template <
typename E>
908 template <
typename E>
915 template <
typename E>
922 template <
typename E>
923 typename VectorMatrixUnaryTraits<E, DiagonalMatrixFromVector<E> >::ResultType
928 return ExpressionType(e());
931 template <
typename E>
932 typename VectorMatrixUnaryTraits<E, CrossProductMatrixFromVector<E> >::ResultType
937 return ExpressionType(e());
940 template <
typename E>
946 template <
typename E>
952 template <
typename E>
961 #endif // CDPL_MATH_MATRIXEXPRESSION_HPP
M::DifferenceType DifferenceType
Definition: MatrixExpression.hpp:513
SizeType getSize1() const
Definition: MatrixExpression.hpp:476
const ValueType ConstReference
Definition: MatrixExpression.hpp:211
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:752
SizeType getSize() const
Definition: MatrixExpression.hpp:374
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:213
std::conditional< std::is_const< M >::value, typename M::ConstReference, typename M::Reference >::type Reference
Definition: MatrixExpression.hpp:518
SizeType getSize2() const
Definition: MatrixExpression.hpp:277
Definition: TypeTraits.hpp:179
VectorMatrixBinary< E1, E2, F > ExpressionType
Definition: MatrixExpression.hpp:296
GridEquality< E1, E2 >::ResultType operator!=(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:346
#define CDPL_MATH_CHECK_SIZE_EQUALITY(size1, size2, e)
Definition: Check.hpp:62
Definition: MatrixExpression.hpp:149
const unsigned int E
Specifies that the stereocenter has E configuration.
Definition: CIPDescriptor.hpp:96
SizeType getSize1() const
Definition: MatrixExpression.hpp:120
SelfType ClosureType
Definition: MatrixExpression.hpp:265
std::common_type< T1, T2 >::type Type
Definition: CommonType.hpp:43
Matrix1VectorBinary(const Expression1Type &e1, const Expression2Type &e2)
Definition: MatrixExpression.hpp:320
MatrixTranspose< E > trans(MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:941
VectorMatrixUnaryTraits< E, CrossProductMatrixFromVector< E > >::ResultType cross(const VectorExpression< E > &e)
Definition: MatrixExpression.hpp:933
MatrixTranspose & plusAssign(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:626
F::ResultType ValueType
Definition: MatrixExpression.hpp:312
MatrixTranspose & operator=(const MatrixTranspose< M1 > &mt)
Definition: MatrixExpression.hpp:575
VectorMatrixBinaryTraits< E1, E2, ScalarMultiplication< typename E1::ValueType, typename E2::ValueType > >::ResultType outerProd(const VectorExpression< E1 > &e1, const VectorExpression< E2 > &e2)
Definition: MatrixExpression.hpp:794
static ResultType apply(const MatrixExpression< M1 > &e1, const MatrixExpression< M2 > &e2)
Definition: Functional.hpp:571
CommonType< typename E1::SizeType, typename E2::SizeType >::Type SizeType
Definition: MatrixExpression.hpp:317
SizeType getSize1() const
Definition: MatrixExpression.hpp:72
MatrixScalarUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:661
std::enable_if< IsScalar< T >::value, typename Scalar2GridBinaryTraits< E, T, ScalarMultiplication< typename E::ValueType, T > >::ResultType >::type operator*(const GridExpression< E > &e, const T &t)
Definition: GridExpression.hpp:309
Matrix2VectorBinary< E1, E2, F > ExpressionType
Definition: MatrixExpression.hpp:398
MatrixUnary(const ExpressionType &e)
Definition: MatrixExpression.hpp:69
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:663
Definition: MatrixExpression.hpp:243
M MatrixType
Definition: MatrixExpression.hpp:511
E::DifferenceType DifferenceType
Definition: MatrixExpression.hpp:115
GridEquality< E1, E2 >::ResultType operator==(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:339
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:231
const ValueType ConstReference
Definition: MatrixExpression.hpp:415
M::SizeType SizeType
Definition: MatrixExpression.hpp:512
Definition: MatrixExpression.hpp:141
SelfType ClosureType
Definition: MatrixExpression.hpp:367
Definition: Expression.hpp:54
Definition: MatrixExpression.hpp:506
const ValueType Reference
Definition: MatrixExpression.hpp:161
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:522
F::ResultType ValueType
Definition: MatrixExpression.hpp:159
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:112
SizeType getSize2() const
Definition: MatrixExpression.hpp:77
SelfType ClosureType
Definition: MatrixExpression.hpp:113
GridUnaryTraits< E, ScalarConjugation< typename E::ValueType > >::ResultType herm(const GridExpression< E > &e)
Definition: GridExpression.hpp:369
SizeType getSize() const
Definition: MatrixExpression.hpp:323
F::ResultType ValueType
Definition: MatrixExpression.hpp:109
F::ResultType ValueType
Definition: MatrixExpression.hpp:465
SizeType getSize2() const
Definition: MatrixExpression.hpp:481
ExpressionType ResultType
Definition: MatrixExpression.hpp:144
MatrixTranspose & minusAssign(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:633
GridUnaryTraits< E, ScalarReal< typename E::ValueType > >::ResultType real(const GridExpression< E > &e)
Definition: GridExpression.hpp:378
Reference operator()(SizeType i, SizeType j)
Definition: MatrixExpression.hpp:528
SizeType getSize2() const
Definition: MatrixExpression.hpp:175
E1::DifferenceType DifferenceType
Definition: MatrixExpression.hpp:471
Definition: MatrixExpression.hpp:200
GridUnaryTraits< E, ScalarNegation< typename E::ValueType > >::ResultType operator-(const GridExpression< E > &e)
Definition: GridExpression.hpp:272
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:315
Definition: Functional.hpp:239
F::ResultType ValueType
Definition: MatrixExpression.hpp:261
const ValueType Reference
Definition: MatrixExpression.hpp:263
ExpressionType ResultType
Definition: MatrixExpression.hpp:246
Scalar1MatrixBinary< E1, E2, F > ExpressionType
Definition: MatrixExpression.hpp:449
Definition: Functional.hpp:211
Definition: Expression.hpp:76
const ValueType Reference
Definition: MatrixExpression.hpp:111
MatrixTranspose & operator-=(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:596
MatrixTranspose & assign(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:619
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:366
MatrixBinary2(const Expression1Type &e1, const Expression2Type &e2)
Definition: MatrixExpression.hpp:218
MatrixScalarUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:639
Definition: Functional.hpp:253
E::DifferenceType DifferenceType
Definition: MatrixExpression.hpp:67
std::conditional< std::is_const< M >::value, typename M::ConstClosureType, typename M::ClosureType >::type MatrixClosureType
Definition: MatrixExpression.hpp:521
static ResultType apply(const MatrixExpression< M1 > &e1, const MatrixExpression< M2 > &e2, Argument3Type epsilon)
Definition: Functional.hpp:607
Definition: MatrixExpression.hpp:302
MatrixScalarRealUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:692
MatrixBinary2< E1, E2, F > ExpressionType
Definition: MatrixExpression.hpp:245
ExpressionType ResultType
Definition: MatrixExpression.hpp:501
M::ValueType ValueType
Definition: MatrixExpression.hpp:514
const ValueType Reference
Definition: MatrixExpression.hpp:467
SizeType getSize1() const
Definition: MatrixExpression.hpp:221
const ValueType ConstReference
Definition: MatrixExpression.hpp:466
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:180
SelfType ClosureType
Definition: MatrixExpression.hpp:65
GridBinary1Traits< E1, E2, ScalarDivision< typename E1::ValueType, typename E2::ValueType > >::ResultType elemDiv(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:396
Definition: MatrixExpression.hpp:101
MatrixTranspose & operator+=(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:589
SelfType ClosureType
Definition: MatrixExpression.hpp:418
GridElementSum< E >::ResultType sum(const GridExpression< E > &e)
Definition: GridExpression.hpp:416
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
ConstReference operator[](SizeType i) const
Definition: MatrixExpression.hpp:384
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:694
const ValueType ConstReference
Definition: MatrixExpression.hpp:62
SelfType ClosureType
Definition: MatrixExpression.hpp:469
const unsigned int M
A generic type that covers any element that is a metal.
Definition: AtomType.hpp:637
Definition of type traits.
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:468
Matrix1VectorBinaryTraits< E1, E2, MatrixVectorProduct< E1, E2 > >::ResultType prod(const MatrixExpression< E1 > &e1, const VectorExpression< E2 > &e2)
Definition: MatrixExpression.hpp:833
Definition: Functional.hpp:225
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:162
const E & operator+(const GridExpression< E > &e)
Definition: GridExpression.hpp:282
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:130
Matrix2VectorBinary(const Expression1Type &e1, const Expression2Type &e2)
Definition: MatrixExpression.hpp:371
Definition: Expression.hpp:142
E2::DifferenceType DifferenceType
Definition: MatrixExpression.hpp:420
SizeType getSize1() const
Definition: MatrixExpression.hpp:425
MatrixScalarRealUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:722
Matrix1VectorBinary< E1, E2, F > ExpressionType
Definition: MatrixExpression.hpp:347
std::enable_if< IsScalar< T >::value, MatrixTranspose >::type & operator/=(const T &t)
Definition: MatrixExpression.hpp:612
Definition of various functors.
friend void swap(MatrixTranspose &mt1, MatrixTranspose &mt2)
Definition: MatrixExpression.hpp:644
Definition: MatrixExpression.hpp:251
GridUnaryTraits< E, ScalarConjugation< typename E::ValueType > >::ResultType conj(const GridExpression< E > &e)
Definition: GridExpression.hpp:360
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
Definition: TypeTraits.hpp:186
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:264
Definition: MatrixExpression.hpp:353
MatrixUnary< E, F > ExpressionType
Definition: MatrixExpression.hpp:95
Definition: MatrixExpression.hpp:447
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Scalar2MatrixBinary< E1, E2, F > ExpressionType
Definition: MatrixExpression.hpp:500
GridBinary1Traits< E1, E2, ScalarMultiplication< typename E1::ValueType, typename E2::ValueType > >::ResultType elemProd(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:406
ConstReference operator()(SizeType i) const
Definition: MatrixExpression.hpp:379
Definition of exception classes.
MatrixBooleanBinaryFunctor< M1, M2 >::ResultType ResultType
Definition: Functional.hpp:569
const ValueType Reference
Definition: MatrixExpression.hpp:365
VectorMatrixUnary< E, F > ExpressionType
Definition: MatrixExpression.hpp:143
CommonType< typename E1::DifferenceType, typename E2::DifferenceType >::Type DifferenceType
Definition: MatrixExpression.hpp:165
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:486
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:533
MatrixTranspose & operator=(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:582
E::SizeType SizeType
Definition: MatrixExpression.hpp:114
SizeType getSize2() const
Definition: MatrixExpression.hpp:430
const ValueType Reference
Definition: MatrixExpression.hpp:63
const ValueType ConstReference
Definition: MatrixExpression.hpp:160
F::ResultType ValueType
Definition: MatrixExpression.hpp:210
Definition: MatrixExpression.hpp:404
bool isEmpty() const
Definition: MatrixExpression.hpp:553
SizeType getSize2() const
Definition: MatrixExpression.hpp:226
VectorMatrixUnaryTraits< E, DiagonalMatrixFromVector< E > >::ResultType diag(const VectorExpression< E > &e)
Definition: MatrixExpression.hpp:924
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:435
const ValueType ConstReference
Definition: MatrixExpression.hpp:364
The namespace of the Chemical Data Processing Library.
MatrixTranspose & operator=(const MatrixTranspose &mt)
Definition: MatrixExpression.hpp:568
MatrixNormInfinity< E >::ResultType normInf(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:917
ExpressionType ResultType
Definition: MatrixExpression.hpp:348
SizeType getMaxSize() const
Definition: MatrixExpression.hpp:548
ExpressionType ResultType
Definition: MatrixExpression.hpp:96
F::ResultType ValueType
Definition: MatrixExpression.hpp:363
ExpressionType ResultType
Definition: MatrixExpression.hpp:450
const ValueType Reference
Definition: MatrixExpression.hpp:314
std::enable_if< IsScalar< T >::value, typename Scalar2GridBinaryTraits< E, T, ScalarDivision< typename E::ValueType, T > >::ResultType >::type operator/(const GridExpression< E > &e, const T &t)
Definition: GridExpression.hpp:329
SizeType getSize1() const
Definition: MatrixExpression.hpp:170
E::SizeType SizeType
Definition: MatrixExpression.hpp:66
CommonType< typename E1::SizeType, typename E2::SizeType >::Type SizeType
Definition: MatrixExpression.hpp:266
std::enable_if< IsScalar< T >::value, MatrixTranspose >::type & operator*=(const T &t)
Definition: MatrixExpression.hpp:604
const ValueType ConstReference
Definition: MatrixExpression.hpp:313
MatrixBinary1(const Expression1Type &e1, const Expression2Type &e2)
Definition: MatrixExpression.hpp:167
ConstReference operator()(SizeType i) const
Definition: MatrixExpression.hpp:328
CommonType< typename E1::DifferenceType, typename E2::DifferenceType >::Type DifferenceType
Definition: MatrixExpression.hpp:267
MatrixBinary1< E1, E2, F > ExpressionType
Definition: MatrixExpression.hpp:194
SelfType ClosureType
Definition: MatrixExpression.hpp:523
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:724
ExpressionType ResultType
Definition: MatrixExpression.hpp:399
Definition: MatrixExpression.hpp:345
SizeType getSize1() const
Definition: MatrixExpression.hpp:272
const ValueType ConstReference
Definition: MatrixExpression.hpp:110
SelfType ClosureType
Definition: MatrixExpression.hpp:214
CommonType< typename E1::SizeType, typename E2::SizeType >::Type SizeType
Definition: MatrixExpression.hpp:368
MatrixNorm1< E >::ResultType norm1(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:903
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:282
SelfType ClosureType
Definition: MatrixExpression.hpp:163
GridUnaryTraits< E, ScalarImaginary< typename E::ValueType > >::ResultType imag(const GridExpression< E > &e)
Definition: GridExpression.hpp:387
std::enable_if< std::is_arithmetic< T >::value, typename GridToleranceEquality< E1, E2, T >::ResultType >::type equals(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2, const T &eps)
Definition: GridExpression.hpp:353
CommonType< typename E1::DifferenceType, typename E2::DifferenceType >::Type DifferenceType
Definition: MatrixExpression.hpp:216
SizeType getSize1() const
Definition: MatrixExpression.hpp:538
Definition: MatrixExpression.hpp:53
MatrixNormFrobenius< E >::ResultType normFrob(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:910
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixExpression.hpp:82
MatrixTrace< E >::ResultType trace(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:896
const ValueType Reference
Definition: MatrixExpression.hpp:212
MatrixTranspose(MatrixType &m)
Definition: MatrixExpression.hpp:525
E2::SizeType SizeType
Definition: MatrixExpression.hpp:419
SelfType ClosureType
Definition: MatrixExpression.hpp:316
Definition of various preprocessor macros for error checking.
CommonType< typename E1::DifferenceType, typename E2::DifferenceType >::Type DifferenceType
Definition: MatrixExpression.hpp:369
ConstReference operator[](SizeType i) const
Definition: MatrixExpression.hpp:333
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:64
F::ResultType ValueType
Definition: MatrixExpression.hpp:414
void swap(MatrixTranspose &mt)
Definition: MatrixExpression.hpp:639
const MatrixClosureType & getData() const
Definition: MatrixExpression.hpp:563
const SelfType ConstClosureType
Definition: MatrixExpression.hpp:417
CommonType< typename E1::DifferenceType, typename E2::DifferenceType >::Type DifferenceType
Definition: MatrixExpression.hpp:318
Definition: MatrixExpression.hpp:455
VectorMatrixBinary(const Expression1Type &e1, const Expression2Type &e2)
Definition: MatrixExpression.hpp:269
ExpressionType ResultType
Definition: MatrixExpression.hpp:297
Scalar3MatrixBooleanTernaryFunctor< M1, M2, T >::ResultType ResultType
Definition: Functional.hpp:604
Definition of basic expression types.
Definition: MatrixExpression.hpp:192
CommonType< typename E1::SizeType, typename E2::SizeType >::Type SizeType
Definition: MatrixExpression.hpp:164
SizeType getSize2() const
Definition: MatrixExpression.hpp:125
E1::SizeType SizeType
Definition: MatrixExpression.hpp:470
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:641
MatrixClosureType & getData()
Definition: MatrixExpression.hpp:558
Definition: MatrixExpression.hpp:93
Scalar2MatrixBinary(const Expression1Type &e1, const Expression2Type &e2)
Definition: MatrixExpression.hpp:473
CommonType< typename E1::SizeType, typename E2::SizeType >::Type SizeType
Definition: MatrixExpression.hpp:215
const ValueType ConstReference
Definition: MatrixExpression.hpp:262
const ValueType Reference
Definition: MatrixExpression.hpp:416
Definition: MatrixExpression.hpp:294
Definition: MatrixExpression.hpp:498
const unsigned int F
Specifies Fluorine.
Definition: AtomType.hpp:107
SizeType getSize2() const
Definition: MatrixExpression.hpp:543
ExpressionType ResultType
Definition: MatrixExpression.hpp:195
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92
VectorMatrixUnary(const ExpressionType &e)
Definition: MatrixExpression.hpp:117
Scalar1MatrixBinary(const Expression1Type &e1, const Expression2Type &e2)
Definition: MatrixExpression.hpp:422
Definition: Expression.hpp:164
MatrixScalarRealUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:750
M::ConstReference ConstReference
Definition: MatrixExpression.hpp:515
F::ResultType ValueType
Definition: MatrixExpression.hpp:61
Definition: MatrixExpression.hpp:396