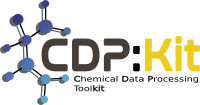 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_MATRIXASSIGNMENT_HPP
28 #define CDPL_MATH_MATRIXASSIGNMENT_HPP
44 class MatrixExpression;
46 template <
template <
typename T1,
typename T2>
class F,
typename M,
typename E>
54 typedef F<typename M::Reference, typename E::ValueType> FunctorType;
56 for (SizeType i = 0; i < size1; i++)
57 for (SizeType j = 0; j < size2; j++)
58 FunctorType::apply(
m(i, j), e()(i, j));
61 template <
template <
typename T1,
typename T2>
class F,
typename M,
typename T>
64 typedef F<typename M::Reference, T> FunctorType;
65 typedef typename M::SizeType SizeType;
67 SizeType size1 =
m.getSize1();
68 SizeType size2 =
m.getSize2();
70 for (SizeType i = 0; i < size1; i++)
71 for (SizeType j = 0; j < size2; j++)
72 FunctorType::apply(
m(i, j), t);
75 template <
typename M,
typename E>
83 for (SizeType i = 0; i < size1; i++)
84 for (SizeType j = 0; j < size2; j++)
85 std::swap(
m(i, j), e()(i, j));
90 #endif // CDPL_MATH_MATRIXASSIGNMENT_HPP
#define CDPL_MATH_CHECK_SIZE_EQUALITY(size1, size2, e)
Definition: Check.hpp:62
const unsigned int E
Specifies that the stereocenter has E configuration.
Definition: CIPDescriptor.hpp:96
std::common_type< T1, T2 >::type Type
Definition: CommonType.hpp:43
void matrixAssignScalar(M &m, const T &t)
Definition: MatrixAssignment.hpp:62
Definition: Expression.hpp:76
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
const unsigned int M
A generic type that covers any element that is a metal.
Definition: AtomType.hpp:637
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
void matrixAssignMatrix(M &m, const MatrixExpression< E > &e)
Definition: MatrixAssignment.hpp:47
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Definition of exception classes.
The namespace of the Chemical Data Processing Library.
Definition of various preprocessor macros for error checking.
void matrixSwap(M &m, MatrixExpression< E > &e)
Definition: MatrixAssignment.hpp:76
const unsigned int F
Specifies Fluorine.
Definition: AtomType.hpp:107