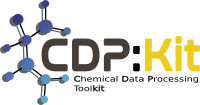 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_MATRIXADAPTER_HPP
28 #define CDPL_MATH_MATRIXADAPTER_HPP
30 #include <type_traits>
49 static typename M::ConstReference
get(
const M&
m,
typename M::SizeType i,
typename M::SizeType j)
52 return m.getData()(i, j);
62 static typename M::ConstReference
get(
const M&
m,
typename M::SizeType i,
typename M::SizeType j)
68 return m.getData()(i, j);
78 static typename M::ConstReference
get(
const M&
m,
typename M::SizeType i,
typename M::SizeType j)
81 return m.getData()(i, j);
91 static typename M::ConstReference
get(
const M&
m,
typename M::SizeType i,
typename M::SizeType j)
97 return m.getData()(i, j);
103 template <
typename M,
typename Tri>
121 typedef typename std::conditional<std::is_const<M>::value,
122 typename M::ConstReference,
124 typedef typename std::conditional<std::is_const<M>::value,
125 typename M::ConstClosureType,
136 return TriangularType::template get<SelfType>(*
this, i, j);
141 return data.getSize1();
146 return data.getSize2();
161 return (data.getSize1() == 0 || data.getSize2() == 0);
170 template <
typename M,
typename Tri>
173 template <
typename M,
typename Tri>
176 template <
typename M,
typename Tri>
180 template <
typename M,
typename Tri>
184 template <
typename M,
typename Tri>
188 template <
typename M,
typename Tri>
192 template <
typename Tri,
typename E>
199 template <
typename Tri,
typename E>
200 TriangularAdapter<const E, Tri>
208 #endif // CDPL_MATH_MATRIXADAPTER_HPP
Definition: TypeTraits.hpp:179
Definition: MatrixAdapter.hpp:59
M::ValueType ValueType
Definition: MatrixAdapter.hpp:119
Definition: MatrixAdapter.hpp:88
const MatrixClosureType & getData() const
Definition: MatrixAdapter.hpp:154
Range< SizeType > RangeType
Definition: MatrixAdapter.hpp:129
bool isEmpty() const
Definition: MatrixAdapter.hpp:159
SizeType getSize1() const
Definition: MatrixAdapter.hpp:139
static M::ConstReference get(const M &m, typename M::SizeType i, typename M::SizeType j)
Definition: MatrixAdapter.hpp:49
Definition: MatrixAdapter.hpp:46
Definition: Expression.hpp:76
SizeType getSize2() const
Definition: MatrixAdapter.hpp:144
Definition: MatrixAdapter.hpp:105
static M::ConstReference get(const M &m, typename M::SizeType i, typename M::SizeType j)
Definition: MatrixAdapter.hpp:62
MatrixClosureType & getData()
Definition: MatrixAdapter.hpp:149
const unsigned int M
A generic type that covers any element that is a metal.
Definition: AtomType.hpp:637
Definition of type traits.
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
Definition: TypeTraits.hpp:186
M::SizeType SizeType
Definition: MatrixAdapter.hpp:117
TriangularAdapter(MatrixType &m)
Definition: MatrixAdapter.hpp:131
static M::ConstReference get(const M &m, typename M::SizeType i, typename M::SizeType j)
Definition: MatrixAdapter.hpp:78
M::DifferenceType DifferenceType
Definition: MatrixAdapter.hpp:118
The namespace of the Chemical Data Processing Library.
static M::ConstReference get(const M &m, typename M::SizeType i, typename M::SizeType j)
Definition: MatrixAdapter.hpp:91
Tri TriangularType
Definition: MatrixAdapter.hpp:116
M MatrixType
Definition: MatrixAdapter.hpp:115
Definition: MatrixAdapter.hpp:75
SelfType ClosureType
Definition: MatrixAdapter.hpp:128
std::conditional< std::is_const< M >::value, typename M::ConstClosureType, typename M::ClosureType >::type MatrixClosureType
Definition: MatrixAdapter.hpp:126
TriangularAdapter< E, Tri > triang(MatrixExpression< E > &e)
Definition: MatrixAdapter.hpp:194
const SelfType ConstClosureType
Definition: MatrixAdapter.hpp:127
std::conditional< std::is_const< M >::value, typename M::ConstReference, typename M::Reference >::type Reference
Definition: MatrixAdapter.hpp:123
Definition of basic expression types.
M::ConstReference ConstReference
Definition: MatrixAdapter.hpp:120
ConstReference operator()(SizeType i, SizeType j) const
Definition: MatrixAdapter.hpp:134