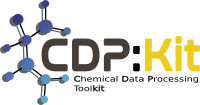 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_GRID_HPP
28 #define CDPL_MATH_GRID_HPP
34 #include <type_traits>
61 typedef typename std::conditional<std::is_const<G>::value,
62 typename G::ConstReference,
95 return data.getSize();
100 return data.getSize1();
105 return data.getSize2();
110 return data.getSize3();
115 return data.getMaxSize();
120 return data.getMaxSize1();
125 return data.getMaxSize2();
130 return data.getMaxSize3();
135 return data.isEmpty();
150 data.operator=(
r.data);
154 template <
typename E>
161 template <
typename E>
168 template <
typename E>
175 template <
typename T>
182 template <
typename T>
189 template <
typename E>
196 template <
typename E>
203 template <
typename E>
224 template <
typename T,
typename A = std::vector<T> >
245 data(), size1(0), size2(0), size3(0) {}
248 data(storageSize(
m, n, o)), size1(
m), size2(n), size3(o) {}
251 data(storageSize(
m, n, o), v), size1(
m), size2(n), size3(o) {}
254 data(g.data), size1(g.size1), size2(g.size2), size3(g.size3) {}
257 data(), size1(0), size2(0), size3(0)
262 template <
typename E>
266 gridAssignGrid<ScalarAssignment>(*
this, e);
300 return (size1 * size2 * size3);
320 return data.max_size();
348 template <
typename C>
354 template <
typename E>
362 template <
typename C>
368 template <
typename E>
376 template <
typename C>
382 template <
typename E>
390 template <
typename T1>
391 typename std::enable_if<IsScalar<T1>::value,
Grid>::type&
operator*=(
const T1& t)
393 gridAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
397 template <
typename T1>
398 typename std::enable_if<IsScalar<T1>::value,
Grid>::type&
operator/=(
const T1& t)
400 gridAssignScalar<ScalarDivisionAssignment>(*
this, t);
404 template <
typename E>
408 gridAssignGrid<ScalarAssignment>(*
this, e);
412 template <
typename E>
415 gridAssignGrid<ScalarAdditionAssignment>(*
this, e);
419 template <
typename E>
422 gridAssignGrid<ScalarSubtractionAssignment>(*
this, e);
429 std::swap(data, g.data);
430 std::swap(size1, g.size1);
431 std::swap(size2, g.size2);
432 std::swap(size3, g.size3);
443 std::fill(data.begin(), data.end(), v);
448 if (size1 ==
m && size2 == n && size3 == o)
452 Grid tmp(
m, n, o, v);
454 for (
SizeType i = 0, min_size1 = std::min(size1,
m); i < min_size1; i++)
455 for (
SizeType j = 0, min_size2 = std::min(size2, n); j < min_size2; j++)
456 for (
SizeType k = 0, min_size3 = std::min(size3, o); k < min_size3; k++)
457 tmp(i, j, k) = (*this)(i, j, k);
462 data.resize(storageSize(
m, n, o), v);
473 (n <= (std::numeric_limits<SizeType>::max() / o) &&
m <= (std::numeric_limits<SizeType>::max() / (n * o))),
484 template <
typename T>
501 size1(0), size2(0), size3(0) {}
504 size1(
m), size2(n), size3(o) {}
507 size1(
m.size1), size2(
m.size2), size3(
m.size3) {}
523 return (size1 == 0 || size2 == 0 || size3 == 0);
528 return (size1 * size2 * size3);
548 return std::numeric_limits<SizeType>::max();
553 return std::numeric_limits<SizeType>::max();
558 return std::numeric_limits<SizeType>::max();
575 std::swap(size1, g.size1);
576 std::swap(size2, g.size2);
577 std::swap(size3, g.size3);
600 template <
typename T>
603 template <
typename T>
620 size1(0), size2(0), size3(0), value() {}
623 size1(
m), size2(n), size3(o), value(v) {}
626 size1(
m.size1), size2(
m.size2), size3(
m.size3), value(
m.value) {}
642 return (size1 == 0 || size2 == 0 || size3 == 0);
647 return (size1 * size2 * size3);
667 return std::numeric_limits<SizeType>::max();
672 return std::numeric_limits<SizeType>::max();
677 return std::numeric_limits<SizeType>::max();
695 std::swap(size1, g.size1);
696 std::swap(size2, g.size2);
697 std::swap(size3, g.size3);
698 std::swap(value, g.value);
721 template <
typename G>
725 template <
typename G>
747 #endif // CDPL_MATH_GRID_HPP
Grid & operator-=(const GridContainer< C > &c)
Definition: Math/Grid.hpp:377
GridReference & assign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:190
void resize(SizeType m, SizeType n, SizeType o)
Definition: Math/Grid.hpp:707
SizeType getSize2() const
Definition: Math/Grid.hpp:536
GridReference & operator+=(const GridExpression< E > &e)
Definition: Math/Grid.hpp:162
void resize(SizeType m, SizeType n, SizeType o)
Definition: Math/Grid.hpp:586
GridReference & operator-=(const GridExpression< E > &e)
Definition: Math/Grid.hpp:169
SizeType getMaxSize2() const
Definition: Math/Grid.hpp:123
SelfType GridTemporaryType
Definition: Math/Grid.hpp:241
Definition of various grid expression types and operations.
SizeType getSize1() const
Definition: Math/Grid.hpp:98
T * Pointer
Definition: Math/Grid.hpp:237
std::size_t SizeType
Definition: Math/Grid.hpp:494
SizeType getMaxSize2() const
Definition: Math/Grid.hpp:670
SizeType getSize3() const
Definition: Math/Grid.hpp:313
void swap(ZeroGrid &g)
Definition: Math/Grid.hpp:572
T & Reference
Definition: Math/Grid.hpp:232
void swap(GridReference &r)
Definition: Math/Grid.hpp:210
void resize(SizeType m, SizeType n, SizeType o, bool preserve=true, const ValueType &v=ValueType())
Definition: Math/Grid.hpp:446
ConstReference operator()(SizeType i) const
Definition: Math/Grid.hpp:78
GridReference< SelfType > ClosureType
Definition: Math/Grid.hpp:496
SizeType getSize2() const
Definition: Math/Grid.hpp:103
SizeType getMaxSize1() const
Definition: Math/Grid.hpp:665
GridReference & operator=(const GridReference &r)
Definition: Math/Grid.hpp:148
bool isEmpty() const
Definition: Math/Grid.hpp:293
ZeroGrid(const ZeroGrid &m)
Definition: Math/Grid.hpp:506
Grid & minusAssign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:420
GridReference & minusAssign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:204
ScalarGrid(SizeType m, SizeType n, SizeType o, const ValueType &v=ValueType())
Definition: Math/Grid.hpp:622
GridReference & plusAssign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:197
Grid & operator=(const GridContainer< C > &c)
Definition: Math/Grid.hpp:349
Grid & operator=(const Grid &g)
Definition: Math/Grid.hpp:333
SizeType getMaxSize() const
Definition: Math/Grid.hpp:318
friend void swap(Grid &g1, Grid &g2)
Definition: Math/Grid.hpp:436
Grid(SizeType m, SizeType n, SizeType o, const ValueType &v)
Definition: Math/Grid.hpp:250
A ArrayType
Definition: Math/Grid.hpp:236
void clear(const ValueType &v=ValueType())
Definition: Math/Grid.hpp:441
Definition: TypeTraits.hpp:200
A::size_type SizeType
Definition: Math/Grid.hpp:234
const T & ConstReference
Definition: Math/Grid.hpp:612
G GridType
Definition: Math/Grid.hpp:59
#define CDPL_MATH_CHECK(expr, msg, e)
Definition: Check.hpp:36
ConstReference operator()(SizeType i) const
Definition: Math/Grid.hpp:628
void swap(Grid &g)
Definition: Math/Grid.hpp:426
bool isEmpty() const
Definition: Math/Grid.hpp:640
ConstReference operator()(SizeType i) const
Definition: Math/Grid.hpp:509
Reference operator()(SizeType i, SizeType j, SizeType k)
Definition: Math/Grid.hpp:281
SelfType ClosureType
Definition: Math/Grid.hpp:67
Grid & operator=(Grid &&g)
Definition: Math/Grid.hpp:342
G::SizeType SizeType
Definition: Math/Grid.hpp:65
const GridReference< const SelfType > ConstClosureType
Definition: Math/Grid.hpp:616
SizeType getSize1() const
Definition: Math/Grid.hpp:303
const T & Reference
Definition: Math/Grid.hpp:611
Grid & operator+=(const GridContainer< C > &c)
Definition: Math/Grid.hpp:363
Grid & plusAssign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:413
ZeroGrid & operator=(const ZeroGrid &g)
Definition: Math/Grid.hpp:561
SizeType getMaxSize3() const
Definition: Math/Grid.hpp:128
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
SizeType getSize1() const
Definition: Math/Grid.hpp:650
ZeroGrid< float > FZeroGrid
Definition: Math/Grid.hpp:729
Grid()
Definition: Math/Grid.hpp:244
std::conditional< std::is_const< G >::value, typename G::ConstReference, typename G::Reference >::type Reference
Definition: Math/Grid.hpp:63
Grid & operator=(const GridExpression< E > &e)
Definition: Math/Grid.hpp:355
GridReference(GridType &g)
Definition: Math/Grid.hpp:70
Grid< T > GridTemporaryType
Definition: Math/Grid.hpp:617
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
const T & ConstReference
Definition: Math/Grid.hpp:493
friend void swap(ZeroGrid &g1, ZeroGrid &g2)
Definition: Math/Grid.hpp:581
Definition: Math/Grid.hpp:605
bool isEmpty() const
Definition: Math/Grid.hpp:133
SizeType getMaxSize1() const
Definition: Math/Grid.hpp:546
Definition of type traits.
ArrayType & getData()
Definition: Math/Grid.hpp:323
ConstReference operator()(SizeType i, SizeType j, SizeType k) const
Definition: Math/Grid.hpp:88
Grid(SizeType m, SizeType n, SizeType o)
Definition: Math/Grid.hpp:247
Reference operator()(SizeType i)
Definition: Math/Grid.hpp:269
ZeroGrid(SizeType m, SizeType n, SizeType o)
Definition: Math/Grid.hpp:503
SizeType getSize3() const
Definition: Math/Grid.hpp:108
ConstReference operator()(SizeType i, SizeType j, SizeType k) const
Definition: Math/Grid.hpp:634
SizeType getSize2() const
Definition: Math/Grid.hpp:308
std::shared_ptr< SelfType > SharedPointer
Definition: Math/Grid.hpp:242
ZeroGrid< double > DZeroGrid
Definition: Math/Grid.hpp:730
ConstReference operator()(SizeType i, SizeType j, SizeType k) const
Definition: Math/Grid.hpp:287
const GridReference< const SelfType > ConstClosureType
Definition: Math/Grid.hpp:497
Definition of various functors.
ConstReference operator()(SizeType i) const
Definition: Math/Grid.hpp:275
friend void swap(ScalarGrid &g1, ScalarGrid &g2)
Definition: Math/Grid.hpp:702
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
GridReference< SelfType > ClosureType
Definition: Math/Grid.hpp:239
Grid< T > GridTemporaryType
Definition: Math/Grid.hpp:498
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
ScalarGrid< float > FScalarGrid
Definition: Math/Grid.hpp:732
Grid< float > FGrid
An unbounded dense grid holding floating point values of type float.
Definition: Math/Grid.hpp:738
Definition of exception classes.
std::ptrdiff_t DifferenceType
Definition: Math/Grid.hpp:614
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
Definition: Expression.hpp:120
const ArrayType & getData() const
Definition: Math/Grid.hpp:328
ZeroGrid()
Definition: Math/Grid.hpp:500
SizeType getMaxSize1() const
Definition: Math/Grid.hpp:118
const SelfType ConstClosureType
Definition: Math/Grid.hpp:68
ScalarGrid()
Definition: Math/Grid.hpp:619
G::ValueType ValueType
Definition: Math/Grid.hpp:60
T ValueType
Definition: Math/Grid.hpp:491
Definition: Math/Grid.hpp:486
Grid & assign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:405
The namespace of the Chemical Data Processing Library.
G::DifferenceType DifferenceType
Definition: Math/Grid.hpp:66
std::ptrdiff_t DifferenceType
Definition: Math/Grid.hpp:495
GridType & getData()
Definition: Math/Grid.hpp:143
SizeType getSize1() const
Definition: Math/Grid.hpp:531
friend void swap(GridReference &r1, GridReference &r2)
Definition: Math/Grid.hpp:215
SizeType getSize2() const
Definition: Math/Grid.hpp:655
const T & Reference
Definition: Math/Grid.hpp:492
std::enable_if< IsScalar< T >::value, GridReference >::type & operator*=(const T &t)
Definition: Math/Grid.hpp:176
G::ConstReference ConstReference
Definition: Math/Grid.hpp:64
Reference operator()(SizeType i, SizeType j, SizeType k)
Definition: Math/Grid.hpp:83
Definition: Math/Grid.hpp:226
Grid(Grid &&g)
Definition: Math/Grid.hpp:256
Grid & operator-=(const GridExpression< E > &e)
Definition: Math/Grid.hpp:383
A::difference_type DifferenceType
Definition: Math/Grid.hpp:235
std::enable_if< IsScalar< T1 >::value, Grid >::type & operator/=(const T1 &t)
Definition: Math/Grid.hpp:398
void swap(ScalarGrid &g)
Definition: Math/Grid.hpp:692
ScalarGrid(const ScalarGrid &m)
Definition: Math/Grid.hpp:625
T ValueType
Definition: Math/Grid.hpp:231
T ValueType
Definition: Math/Grid.hpp:610
Grid(const GridExpression< E > &e)
Definition: Math/Grid.hpp:263
Definition: Math/Grid.hpp:54
const T * ConstPointer
Definition: Math/Grid.hpp:238
std::enable_if< IsScalar< T1 >::value, Grid >::type & operator*=(const T1 &t)
Definition: Math/Grid.hpp:391
Definition: Expression.hpp:208
const T & ConstReference
Definition: Math/Grid.hpp:233
SizeType getSize() const
Definition: Math/Grid.hpp:645
ScalarGrid & operator=(const ScalarGrid &g)
Definition: Math/Grid.hpp:680
SizeType getMaxSize2() const
Definition: Math/Grid.hpp:551
SizeType getSize() const
Definition: Math/Grid.hpp:93
Implementation of grid assignment routines.
Grid< double > DGrid
An unbounded dense grid holding floating point values of type double.
Definition: Math/Grid.hpp:743
SizeType getSize() const
Definition: Math/Grid.hpp:526
Definition of various preprocessor macros for error checking.
SizeType getSize3() const
Definition: Math/Grid.hpp:541
bool isEmpty() const
Definition: Math/Grid.hpp:521
std::size_t SizeType
Definition: Math/Grid.hpp:613
ScalarGrid< double > DScalarGrid
Definition: Math/Grid.hpp:733
Reference operator()(SizeType i)
Definition: Math/Grid.hpp:73
const GridType & getData() const
Definition: Math/Grid.hpp:138
GridReference & operator=(const GridExpression< E > &e)
Definition: Math/Grid.hpp:155
SizeType getSize() const
Definition: Math/Grid.hpp:298
SizeType getMaxSize3() const
Definition: Math/Grid.hpp:556
Grid & operator+=(const GridExpression< E > &e)
Definition: Math/Grid.hpp:369
ConstReference operator()(SizeType i, SizeType j, SizeType k) const
Definition: Math/Grid.hpp:515
GridReference< SelfType > ClosureType
Definition: Math/Grid.hpp:615
const unsigned int A
A generic type that covers any element except hydrogen.
Definition: AtomType.hpp:617
SizeType getSize3() const
Definition: Math/Grid.hpp:660
SizeType getMaxSize() const
Definition: Math/Grid.hpp:113
const GridReference< const SelfType > ConstClosureType
Definition: Math/Grid.hpp:240
Grid(const Grid &g)
Definition: Math/Grid.hpp:253
std::enable_if< IsScalar< T >::value, GridReference >::type & operator/=(const T &t)
Definition: Math/Grid.hpp:183
SizeType getMaxSize3() const
Definition: Math/Grid.hpp:675