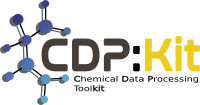 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_MATCHEXPRESSION_HPP
30 #define CDPL_CHEM_MATCHEXPRESSION_HPP
47 class AtomBondMapping;
73 template <
typename ObjType1,
typename ObjType2 =
void>
99 virtual bool operator()(
const ObjType1& query_obj1,
const ObjType2& query_obj2,
const ObjType1& target_obj1,
const ObjType2& target_obj2,
114 virtual bool operator()(
const ObjType1& query_obj1,
const ObjType2& query_obj2,
const ObjType1& target_obj1,
const ObjType2& target_obj2,
148 template <
typename ObjType>
172 virtual bool operator()(
const ObjType& query_obj,
const ObjType& target_obj,
const Base::Any& aux_data)
const;
200 template <
typename ObjType1,
typename ObjType2>
202 const ObjType1&,
const ObjType2&,
208 template <
typename ObjType1,
typename ObjType2>
210 const ObjType1& target_obj1,
const ObjType2& target_obj2,
213 return operator()(query_obj1, query_obj2, target_obj1, target_obj2, data);
216 template <
typename ObjType1,
typename ObjType2>
223 template <
typename ObjType>
230 template <
typename ObjType>
234 return operator()(query_obj, target_obj, data);
237 template <
typename ObjType1>
243 #endif // CDPL_CHEM_MATCHEXPRESSION_HPP
virtual bool operator()(const ObjType1 &query_obj1, const ObjType2 &query_obj2, const ObjType1 &target_obj1, const ObjType2 &target_obj2, const Base::Any &aux_data) const
Performs an evaluation of the expression for the given query and target objects.
Definition: MatchExpression.hpp:201
const unsigned int Any
Specifies any atom.
Definition: SybylAtomType.hpp:198
virtual bool requiresAtomBondMapping() const
Tells whether the expression must be reevaluated after a query to target atom/bond mapping candidate ...
Definition: MatchExpression.hpp:217
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
std::shared_ptr< MatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchExpression instances.
Definition: MatchExpression.hpp:156
virtual ~MatchExpression()
Virtual Destructor.
Definition: MatchExpression.hpp:161
std::shared_ptr< MatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchExpression instances.
Definition: MatchExpression.hpp:81
virtual bool requiresAtomBondMapping() const
Tells whether the expression must be reevaluated after a query to target atom/bond mapping candidate ...
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
The namespace of the Chemical Data Processing Library.
virtual bool operator()(const ObjType1 &query_obj1, const ObjType2 &query_obj2, const ObjType1 &target_obj1, const ObjType2 &target_obj2, const AtomBondMapping &mapping, const Base::Any &aux_data) const
Performs an evaluation of the expression for the given query and target objects under consideration o...
Definition: MatchExpression.hpp:209
virtual ~MatchExpression()
Virtual Destructor.
Definition: MatchExpression.hpp:86
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55