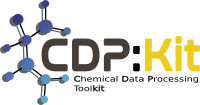 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_MATCHCONSTRAINTLIST_HPP
30 #define CDPL_CHEM_MATCHCONSTRAINTLIST_HPP
109 id(id), relation(relation) {}
118 template <
typename T>
120 id(id), relation(rel), value(std::forward<
T>(val))
159 template <
typename T>
162 return value.getData<
T>();
169 template <
typename T>
172 value = std::forward<T>(val);
262 template <
typename T>
269 const char* getClassName()
const;
276 #endif // CDPL_CHEM_MATCHCONSTRAINTLIST_HPP
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Relation
Defines constants for the specification of relational constraints on the values of query/target attri...
Definition: MatchConstraintList.hpp:58
bool hasValue() const
Tells wether a query attribute value has been set.
void setValue(T &&val)
Sets the value of the query attribute.
Definition: MatchConstraintList.hpp:170
@ LESS_OR_EQUAL
Specifies that the value of the query attribute must be less than or equal to the corresponding value...
Definition: MatchConstraintList.hpp:87
std::shared_ptr< MatchConstraintList > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchConstraintList instances.
Definition: MatchConstraintList.hpp:197
A dynamic array class providing amortized constant time access to arbitrary elements.
Definition: Array.hpp:92
@ EQUAL
Specifies that the value of the query attribute must be equal to the corresponding value of the targe...
Definition: MatchConstraintList.hpp:75
Type getType() const
Returns the logical type of the match constraint list.
@ OR_LIST
Specifies that at least one of the match constraints must be fulfilled.
Definition: MatchConstraintList.hpp:218
@ LESS
Specifies that the value of the query attribute must be less than the corresponding value of the targ...
Definition: MatchConstraintList.hpp:69
Definition of the class CDPL::Util::Array.
MatchConstraint(unsigned int id, Relation rel, T &&val)
Constructs a MatchConstraint object with the given identifier, relational constraint on the values of...
Definition: MatchConstraintList.hpp:119
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
Type
Defines constants that describe the logical type of the match constraint list.
Definition: MatchConstraintList.hpp:203
const Base::Any & getValue() const
Returns the value of the query attribute.
MatchConstraintList.
Definition: MatchConstraintList.hpp:191
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
@ GREATER
Specifies that the value of the query attribute must be greater than the corresponding value of the t...
Definition: MatchConstraintList.hpp:81
The namespace of the Chemical Data Processing Library.
MatchConstraint.
Definition: MatchConstraintList.hpp:50
void addElement(unsigned int id, MatchConstraint::Relation rel, T &&val)
Appends a new Chem::MatchConstraint element with the given identifier, relational constraint on the v...
Definition: MatchConstraintList.hpp:263
@ AND_LIST
Specifies that all of the match constraints must be fulfilled.
Definition: MatchConstraintList.hpp:208
MatchConstraint(unsigned int id, Relation relation)
Constructs a MatchConstraint object with the given identifier and relational constraint on the values...
Definition: MatchConstraintList.hpp:108
unsigned int getID() const
Returns the identifier of the match constraint.
@ GREATER_OR_EQUAL
Specifies that the value of the query attribute must be greater than or equal to the corresponding va...
Definition: MatchConstraintList.hpp:93
void setRelation(Relation rel)
Sets the relational constraint that must be fulfilled by the values of matching query/target attribut...
@ ANY
Specifies that the relation between the query and target attribute value is not constrained.
Definition: MatchConstraintList.hpp:63
MatchConstraintList(Type type=AND_LIST)
Constructs a MatchConstraintList object with the specified logical type.
Definition: MatchConstraintList.hpp:230
@ NOT_AND_LIST
Specifies that at least one of the match constraints must not be fulfilled.
Definition: MatchConstraintList.hpp:213
Definition of the class CDPL::Base::Any.
Relation getRelation() const
Returns the relational constraint that must be fulfilled by the values of matching query/target attri...
void setID(unsigned int id)
Sets the match constraint identifier to id.
const T & getValue() const
Returns a const reference to the value of the query attribute of type T.
Definition: MatchConstraintList.hpp:160
void setType(Type type)
Sets the logical type of the match constraint list.
void addElement(unsigned int id, MatchConstraint::Relation rel)
Appends a new Chem::MatchConstraint element with the given identifier and relational constraint on th...