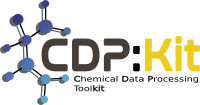 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_MAP_HPP
30 #define CDPL_UTIL_MAP_HPP
48 template <
typename ValueType,
bool Allow = true>
52 static const ValueType&
get()
60 template <
typename ValueType,
bool Allow>
63 template <
typename ValueType>
67 static const ValueType&
get()
93 template <
typename Key,
typename Value,
bool AllowDefValues =
false,
94 typename KeyCompFunc = std::less<Key> >
98 typedef std::map<Key, Value, KeyCompFunc> StorageType;
122 typedef typename StorageType::value_type
Entry;
154 Map(
const KeyCompFunc& func):
162 template <
typename InputIter>
163 Map(
const InputIter& first,
const InputIter& last):
174 template <
typename InputIter>
175 Map(
const InputIter& first,
const InputIter& last,
const KeyCompFunc& func):
176 data(first, last, func)
310 const Value&
getValue(
const Key& key,
const Value& def_value)
const;
390 std::pair<EntryIterator, bool>
insertEntry(
const Key& key,
const Value& value);
462 template <
typename InputIter>
475 template <
typename InputIter>
476 void setEntries(
const InputIter& first,
const InputIter& last);
606 const Value& getDefaultValue()
const;
619 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
631 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
643 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
655 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
667 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
679 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
689 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
690 typename CDPL::Util::Map<Key, Value, AllowDefValues, KeyCompFunc>::StorageType&
696 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
697 const typename CDPL::Util::Map<Key, Value, AllowDefValues, KeyCompFunc>::StorageType&
703 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
709 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
715 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
721 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
727 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
730 return data.key_comp();
733 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
737 return data.find(key);
740 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
744 return data.find(key);
747 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
750 return (data.find(key) != data.end());
753 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
758 if (lb == data.end() || data.key_comp()(key, (*lb).first)) {
762 return (*data.insert(lb,
Entry(key, getDefaultValue()))).second;
768 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
773 if (it == data.end())
779 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
784 if (it == data.end()) {
788 return getDefaultValue();
794 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
796 const Value& def_value)
const
800 if (it == data.end())
806 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
809 return getValue(key);
812 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
815 return getValue(key);
818 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
824 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
827 return (data.erase(key) > 0);
830 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
834 data.erase(first, last);
837 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
838 std::pair<typename CDPL::Util::Map<Key, Value, AllowDefValues, KeyCompFunc>::EntryIterator,
bool>
841 return data.insert(item);
844 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
845 std::pair<typename CDPL::Util::Map<Key, Value, AllowDefValues, KeyCompFunc>::EntryIterator,
bool>
848 return data.insert(
Entry(key, value));
851 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
855 return data.insert(it, item);
858 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
863 return data.insert(it,
Entry(key, value));
866 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
872 if (lb == data.end() || data.key_comp()(item.first, (*lb).first))
873 return data.insert(lb, item);
875 (*lb).second = item.second;
879 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
883 return setEntry(
Entry(key, value));
886 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
887 template <
typename InputIter>
890 data.insert(first, last);
893 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
894 template <
typename InputIter>
897 for (InputIter it = first; it != last; ++it)
901 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
905 return data.lower_bound(key);
908 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
912 return data.lower_bound(key);
915 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
919 return data.upper_bound(key);
922 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
926 return data.upper_bound(key);
929 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
936 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
943 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
950 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
957 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
964 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
971 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
978 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
985 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
989 return data.rbegin();
992 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
996 return data.rbegin();
999 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1006 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1013 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1019 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1027 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1029 const Map<Key, Value, AllowDefValues, KeyCompFunc>& map2)
1031 return (map1.getData() == map2.getData());
1034 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1036 const Map<Key, Value, AllowDefValues, KeyCompFunc>& map2)
1038 return (map1.getData() != map2.getData());
1041 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1043 const Map<Key, Value, AllowDefValues, KeyCompFunc>& map2)
1045 return (map1.getData() <= map2.getData());
1048 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1050 const Map<Key, Value, AllowDefValues, KeyCompFunc>& map2)
1052 return (map1.getData() >= map2.getData());
1055 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1057 const Map<Key, Value, AllowDefValues, KeyCompFunc>& map2)
1059 return (map1.getData() < map2.getData());
1062 template <
typename Key,
typename Value,
bool AllowDefValues,
typename KeyCompFunc>
1064 const Map<Key, Value, AllowDefValues, KeyCompFunc>& map2)
1066 return (map1.getData() > map2.getData());
1071 #endif // CDPL_UTIL_MAP_HPP
const Value & operator[](const Key &key) const
Returns a const reference to the value associated with the specified key.
Definition: Map.hpp:813
EntryIterator setEntry(const Entry &item)
Inserts a new entry or updates the value of an existing entry with the key and value given by item.
Definition: Map.hpp:868
StorageType & getData()
Definition: Map.hpp:691
EntryIterator begin()
Returns a mutable iterator pointing to the beginning of the map.
Definition: Map.hpp:966
EntryIterator setEntry(const Key &key, const Value &value)
Inserts a new entry or updates the value of an existing entry with the specified key and value.
Definition: Map.hpp:881
ConstEntryIterator getEntriesEnd() const
Returns a constant iterator pointing to the end of the map.
Definition: Map.hpp:945
KeyCompFunc getKeyCompareFunction() const
Returns the key compare function used by the map.
Definition: Map.hpp:728
std::shared_ptr< Map > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Map instances.
Definition: Map.hpp:104
Value & getValue(const Key &key)
Returns a non-const reference to the value associated with the specified key.
Definition: Map.hpp:754
bool operator!=(const Array< ValueType > &array1, const Array< ValueType > &array2)
Inequality comparison operator.
void clear()
Erases all entries.
Definition: Map.hpp:716
StorageType::const_iterator ConstEntryIterator
A constant iterator used to iterate over the entries of the map.
Definition: Map.hpp:127
bool isEmpty() const
Tells whether the map is empty (getSize() == 0).
Definition: Map.hpp:710
A unique sorted associative container that maps keys to values.
Definition: Map.hpp:96
bool containsEntry(const Key &key) const
Tells whether the map contains an entry with the specified key.
Definition: Map.hpp:748
ConstEntryIterator getLowerBound(const Key &key) const
Returns a constant iterator pointing to the first entry whose key is not less than the specified key.
Definition: Map.hpp:910
EntryIterator getEntry(const Key &key)
Returns a mutable iterator pointing to the entry specified by key.
Definition: Map.hpp:735
EntryIterator getLowerBound(const Key &key)
Returns a mutable iterator pointing to the first entry whose key is not less than the specified key.
Definition: Map.hpp:903
ConstEntryIterator getEntry(const Key &key) const
Returns a constant iterator pointing to the entry specified by key.
Definition: Map.hpp:742
ConstEntryIterator begin() const
Returns a constant iterator pointing to the beginning of the map.
Definition: Map.hpp:959
ReverseEntryIterator getEntriesReverseBegin()
Returns a mutable iterator pointing to the beginning of the reversed map.
Definition: Map.hpp:994
std::pair< EntryIterator, bool > insertEntry(const Entry &item)
Tries to insert the key/value pair item into the map.
Definition: Map.hpp:839
EntryIterator getUpperBound(const Key &key)
Returns a mutable iterator pointing to the first entry whose key is greater than the specified key.
Definition: Map.hpp:917
Thrown to indicate that some requested operation has failed (e.g. due to unfulfilled preconditions or...
Definition: Base/Exceptions.hpp:211
bool removeEntry(const Key &key)
Removes the entry specified by key from the map.
Definition: Map.hpp:825
Thrown to indicate that some requested data item could not be found.
Definition: Base/Exceptions.hpp:171
const Value & getValue(const Key &key, const Value &def_value) const
Returns a const reference to the value associated with the specified key, or the value given by the s...
Definition: Map.hpp:795
StorageType::value_type Entry
The type of the key/value pairs stored in the map.
Definition: Map.hpp:122
const Value & getValue(const Key &key) const
Returns a const reference to the value associated with the specified key.
Definition: Map.hpp:780
std::size_t getSize() const
Returns the size (number of entries) of the map.
Definition: Map.hpp:704
const StorageType & getData() const
Definition: Map.hpp:698
Value ValueType
The type of the mapped values.
Definition: Map.hpp:114
Map()
Creates an empty map.
Definition: Map.hpp:147
ReverseEntryIterator getEntriesReverseEnd()
Returns a mutable iterator pointing to the end of the reversed map.
Definition: Map.hpp:1008
Value & getValue(const Key &key, Value &def_value)
Returns a non-const reference to the value associated with the specified key, or the value given by t...
Definition: Map.hpp:769
void removeEntry(const EntryIterator &it)
Removes the entry pointed to by the iterator it from the map.
Definition: Map.hpp:819
ConstReverseEntryIterator getEntriesReverseEnd() const
Returns a constant iterator pointing to the end of the reversed map.
Definition: Map.hpp:1001
StorageType::iterator EntryIterator
A mutable iterator used to iterate over the entries of the map.
Definition: Map.hpp:137
EntryIterator insertEntry(const EntryIterator &it, const Entry &item)
Tries to insert the key/value pair item into the map and uses the iterator it as a hint for the inser...
Definition: Map.hpp:853
static const ValueType & get()
Definition: Map.hpp:67
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
bool operator>=(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater or equal comparison operator.
static const ValueType defValue
Definition: Map.hpp:57
void swap(Map &map)
Swaps the contents with map.
Definition: Map.hpp:722
ConstEntryIterator getUpperBound(const Key &key) const
Returns a constant iterator pointing to the first entry whose key is greater than the specified key.
Definition: Map.hpp:924
EntryIterator getEntriesEnd()
Returns a mutable iterator pointing to the end of the map.
Definition: Map.hpp:952
Definition of exception classes.
bool operator<=(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less or equal comparison operator.
The namespace of the Chemical Data Processing Library.
Map(const InputIter &first, const InputIter &last, const KeyCompFunc &func)
Creates and initializes the map with copies of the key value pairs in the range [first,...
Definition: Map.hpp:175
EntryIterator end()
Returns a mutable iterator pointing to the end of the map.
Definition: Map.hpp:980
ConstEntryIterator end() const
Returns a constant iterator pointing to the end of the map.
Definition: Map.hpp:973
std::pair< EntryIterator, bool > insertEntry(const Key &key, const Value &value)
Tries to insert a new entry with specified key and value into the map.
Definition: Map.hpp:846
EntryIterator insertEntry(const EntryIterator &it, const Key &key, const Value &value)
Tries to insert a new entry with the specified key and value into the map and uses the iterator it as...
Definition: Map.hpp:860
ConstReverseEntryIterator getEntriesReverseBegin() const
Returns a constant iterator pointing to the beginning of the reversed map.
Definition: Map.hpp:987
Map(const KeyCompFunc &func)
Creates an empty map and uses func as key compare function.
Definition: Map.hpp:154
void insertEntries(const InputIter &first, const InputIter &last)
Tries to insert the key/value pairs in the range [first, last).
Definition: Map.hpp:888
virtual const char * getClassName() const
Returns the name of the (derived) Map class.
Definition: Map.hpp:1014
Map(const InputIter &first, const InputIter &last)
Creates and initializes the map with copies of the key value pairs in the range [first,...
Definition: Map.hpp:163
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
bool operator==(const Array< ValueType > &array1, const Array< ValueType > &array2)
Equality comparison operator.
void removeEntries(const EntryIterator &first, const EntryIterator &last)
Removes all entries pointed to by the iterators in the range [first, last) from the map.
Definition: Map.hpp:831
Value & operator[](const Key &key)
Returns a non-const reference to the value associated with the specified key.
Definition: Map.hpp:807
StorageType::reverse_iterator ReverseEntryIterator
A mutable iterator used to iterate backwards over the entries of the map.
Definition: Map.hpp:142
ConstEntryIterator getEntriesBegin() const
Returns a constant iterator pointing to the beginning of the map.
Definition: Map.hpp:931
void setEntries(const InputIter &first, const InputIter &last)
Inserts new entries or updates the value of existing entries using the key/value pairs in the range [...
Definition: Map.hpp:895
Key KeyType
The type of the map's keys.
Definition: Map.hpp:109
static const ValueType & get()
Definition: Map.hpp:52
EntryIterator getEntriesBegin()
Returns a mutable iterator pointing to the beginning of the map.
Definition: Map.hpp:938
virtual ~Map()
Virtual destructor.
Definition: Map.hpp:182
StorageType::const_reverse_iterator ConstReverseEntryIterator
A constant iterator used to iterate backwards over the entries of the map.
Definition: Map.hpp:132