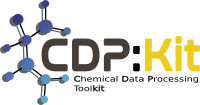 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94STRETCHBENDINTERACTIONPARAMETERIZER_HPP
30 #define CDPL_FORCEFIELD_MMFF94STRETCHBENDINTERACTIONPARAMETERIZER_HPP
34 #include <unordered_map>
37 #include <boost/functional/hash.hpp>
67 typedef std::shared_ptr<MMFF94StretchBendInteractionParameterizer>
SharedPointer;
91 void getBondStretchingParameters(std::size_t atom1_idx, std::size_t atom2_idx,
unsigned int& bond_type_idx,
double& ref_length)
const;
94 const Chem::Atom& term_atom2,
unsigned int term_atom2_type,
unsigned int bond_type_idx1,
unsigned int bond_type_idx2,
95 unsigned int angle_type_idx,
unsigned int& sb_type_idx,
double& ijk_force_const,
double& kji_force_const)
const;
98 const Chem::Atom& term_atom2,
unsigned int bond_type_idx1,
unsigned int bond_type_idx2,
99 unsigned int angle_type_idx,
unsigned int& sb_type_idx,
double& ijk_force_const,
double& kji_force_const,
bool strict)
const;
129 unsigned int getStretchBendTypeIndex(
bool symmetric,
unsigned int bond_type_idx1,
unsigned int bond_type_idx2,
unsigned int angle_type_idx)
const;
133 typedef std::unordered_map<std::pair<std::size_t, std::size_t>,
const MMFF94BondStretchingInteraction*, boost::hash<std::pair<std::size_t, std::size_t> > > BondStretchingParamLookupTable;
140 BondStretchingParamLookupTable bsParamTable;
145 #endif // CDPL_FORCEFIELD_MMFF94STRETCHBENDINTERACTIONPARAMETERIZER_HPP
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
Definition of the class CDPL::ForceField::MMFF94BondStretchingInteractionList.
Definition of the class CDPL::ForceField::MMFF94DefaultStretchBendParameterTable.
void setStretchBendParameterTable(const MMFF94StretchBendParameterTable::SharedPointer &table)
Definition: MMFF94BondStretchingInteraction.hpp:42
Type definition of generic wrapper classes for storing user-defined interaction filtering functions.
Atom.
Definition: Atom.hpp:52
std::shared_ptr< MMFF94AtomTypePropertyTable > SharedPointer
Definition: MMFF94AtomTypePropertyTable.hpp:59
std::shared_ptr< MMFF94DefaultStretchBendParameterTable > SharedPointer
Definition: MMFF94DefaultStretchBendParameterTable.hpp:60
Definition: MMFF94StretchBendInteractionParameterizer.hpp:64
MolecularGraph.
Definition: MolecularGraph.hpp:52
void setFilterFunction(const InteractionFilterFunction3 &func)
void setAtomTypeFunction(const MMFF94NumericAtomTypeFunction &func)
void parameterize(const Chem::MolecularGraph &molgraph, const MMFF94BondStretchingInteractionList &bs_ia_list, const MMFF94AngleBendingInteractionList &ab_ia_list, MMFF94StretchBendInteractionList &ia_list, bool strict)
std::function< unsigned int(const Chem::Atom &)> MMFF94NumericAtomTypeFunction
A generic wrapper class used to store a user-defined numeric MMFF94 atom type function.
Definition: MMFF94PropertyFunctions.hpp:56
MMFF94StretchBendInteractionParameterizer(const Chem::MolecularGraph &molgraph, const MMFF94BondStretchingInteractionList &bs_ia_list, const MMFF94AngleBendingInteractionList &ab_ia_list, MMFF94StretchBendInteractionList &ia_list, bool strict)
Definition of the class CDPL::ForceField::MMFF94StretchBendInteractionList.
std::shared_ptr< MMFF94StretchBendInteractionParameterizer > SharedPointer
Definition: MMFF94StretchBendInteractionParameterizer.hpp:67
Definition of the class CDPL::ForceField::MMFF94StretchBendParameterTable.
The namespace of the Chemical Data Processing Library.
void setDefaultStretchBendParameterTable(const MMFF94DefaultStretchBendParameterTable::SharedPointer &table)
void setAtomTypePropertyTable(const MMFF94AtomTypePropertyTable::SharedPointer &table)
Definition of the class CDPL::ForceField::MMFF94AtomTypePropertyTable.
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::shared_ptr< MMFF94StretchBendParameterTable > SharedPointer
Definition: MMFF94StretchBendParameterTable.hpp:60
std::function< bool(const Chem::Atom &, const Chem::Atom &, const Chem::Atom &)> InteractionFilterFunction3
Definition: InteractionFilterFunctions.hpp:50
Definition of the class CDPL::ForceField::MMFF94AngleBendingInteractionList.
Type definition of generic wrapper classes for storing user-defined functions for the retrieval of MM...
MMFF94StretchBendInteractionParameterizer()