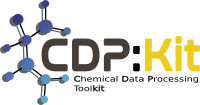 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94PRIMARYTOPARAMETERATOMTYPEMAP_HPP
30 #define CDPL_FORCEFIELD_MMFF94PRIMARYTOPARAMETERATOMTYPEMAP_HPP
34 #include <unordered_map>
38 #include <boost/iterator/transform_iterator.hpp>
56 typedef std::unordered_map<unsigned int, Entry> DataStorage;
59 typedef std::shared_ptr<MMFF94PrimaryToParameterAtomTypeMap>
SharedPointer;
65 static constexpr std::size_t NUM_TYPES = 4;
69 Entry(
unsigned int atom_type,
unsigned int param_types[]);
78 unsigned int atomType;
79 unsigned int paramTypes[NUM_TYPES];
83 typedef boost::transform_iterator<std::function<
const Entry&(
const DataStorage::value_type&)>,
84 DataStorage::const_iterator>
87 typedef boost::transform_iterator<std::function<
Entry&(DataStorage::value_type&)>,
88 DataStorage::iterator>
93 void addEntry(
unsigned int atom_type,
unsigned int param_types[]);
136 #endif // CDPL_FORCEFIELD_MMFF94PRIMARYTOPARAMETERATOMTYPEMAP_HPP
std::shared_ptr< MMFF94PrimaryToParameterAtomTypeMap > SharedPointer
Definition: MMFF94PrimaryToParameterAtomTypeMap.hpp:59
EntryIterator removeEntry(const EntryIterator &it)
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
Entry(unsigned int atom_type, unsigned int param_types[])
MMFF94PrimaryToParameterAtomTypeMap()
Definition: MMFF94PrimaryToParameterAtomTypeMap.hpp:62
static void set(const SharedPointer &table)
Definition: MMFF94PrimaryToParameterAtomTypeMap.hpp:50
const unsigned int * getParameterTypes() const
EntryIterator getEntriesBegin()
unsigned int getAtomType() const
EntryIterator getEntriesEnd()
ConstEntryIterator getEntriesBegin() const
ConstEntryIterator begin() const
ConstEntryIterator end() const
ConstEntryIterator getEntriesEnd() const
std::size_t getNumEntries() const
boost::transform_iterator< std::function< const Entry &(const DataStorage::value_type &)>, DataStorage::const_iterator > ConstEntryIterator
Definition: MMFF94PrimaryToParameterAtomTypeMap.hpp:85
static const SharedPointer & get()
The namespace of the Chemical Data Processing Library.
bool removeEntry(unsigned int atom_type)
void load(std::istream &is)
boost::transform_iterator< std::function< Entry &(DataStorage::value_type &)>, DataStorage::iterator > EntryIterator
Definition: MMFF94PrimaryToParameterAtomTypeMap.hpp:89
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
const Entry & getEntry(unsigned int atom_type) const
void addEntry(unsigned int atom_type, unsigned int param_types[])