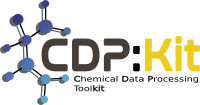 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94OUTOFPLANEBENDINGINTERACTION_HPP
30 #define CDPL_FORCEFIELD_MMFF94OUTOFPLANEBENDINGINTERACTION_HPP
46 std::size_t oop_atom_idx,
double force_const):
47 termAtom1Idx(term_atom1_idx),
48 ctrAtomIdx(ctr_atom_idx), termAtom2Idx(term_atom2_idx),
49 oopAtomIdx(oop_atom_idx), forceConst(force_const) {}
97 std::size_t termAtom1Idx;
98 std::size_t ctrAtomIdx;
99 std::size_t termAtom2Idx;
100 std::size_t oopAtomIdx;
106 #endif // CDPL_FORCEFIELD_MMFF94OUTOFPLANEBENDINGINTERACTION_HPP
std::size_t getAtom3Index() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:81
std::size_t getAtom1Index() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:71
std::size_t getCenterAtomIndex() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:56
MMFF94OutOfPlaneBendingInteraction(std::size_t term_atom1_idx, std::size_t ctr_atom_idx, std::size_t term_atom2_idx, std::size_t oop_atom_idx, double force_const)
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:45
double getForceConstant() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:91
std::size_t getTerminalAtom1Index() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:51
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:42
std::size_t getOutOfPlaneAtomIndex() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:66
The namespace of the Chemical Data Processing Library.
std::size_t getAtom2Index() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:76
std::size_t getTerminalAtom2Index() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:61
std::size_t getAtom4Index() const
Definition: MMFF94OutOfPlaneBendingInteraction.hpp:86