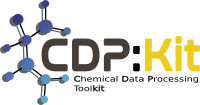 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94BONDCHARGEINCREMENTTABLE_HPP
30 #define CDPL_FORCEFIELD_MMFF94BONDCHARGEINCREMENTTABLE_HPP
35 #include <unordered_map>
39 #include <boost/iterator/transform_iterator.hpp>
57 typedef std::unordered_map<std::uint32_t, Entry> DataStorage;
68 Entry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type,
double bond_chg_inc);
81 unsigned int bondTypeIdx;
82 unsigned int atom1Type;
83 unsigned int atom2Type;
88 typedef boost::transform_iterator<std::function<
const Entry&(
const DataStorage::value_type&)>,
89 DataStorage::const_iterator>
92 typedef boost::transform_iterator<std::function<
Entry&(DataStorage::value_type&)>,
93 DataStorage::iterator>
98 void addEntry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type,
double bond_chg_inc);
100 const Entry&
getEntry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type)
const;
106 bool removeEntry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type);
141 #endif // CDPL_FORCEFIELD_MMFF94BONDCHARGEINCREMENTTABLE_HPP
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
void addEntry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type, double bond_chg_inc)
void load(std::istream &is)
Definition: MMFF94BondChargeIncrementTable.hpp:51
Definition: MMFF94BondChargeIncrementTable.hpp:63
EntryIterator removeEntry(const EntryIterator &it)
static const SharedPointer & get()
MMFF94BondChargeIncrementTable()
double getChargeIncrement() const
static void set(const SharedPointer &table)
std::shared_ptr< MMFF94BondChargeIncrementTable > SharedPointer
Definition: MMFF94BondChargeIncrementTable.hpp:60
ConstEntryIterator getEntriesBegin() const
ConstEntryIterator begin() const
unsigned int getBondTypeIndex() const
ConstEntryIterator getEntriesEnd() const
unsigned int getAtom1Type() const
bool removeEntry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type)
ConstEntryIterator end() const
The namespace of the Chemical Data Processing Library.
boost::transform_iterator< std::function< Entry &(DataStorage::value_type &)>, DataStorage::iterator > EntryIterator
Definition: MMFF94BondChargeIncrementTable.hpp:94
Entry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type, double bond_chg_inc)
boost::transform_iterator< std::function< const Entry &(const DataStorage::value_type &)>, DataStorage::const_iterator > ConstEntryIterator
Definition: MMFF94BondChargeIncrementTable.hpp:90
std::size_t getNumEntries() const
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
const Entry & getEntry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type) const
EntryIterator getEntriesBegin()
unsigned int getAtom2Type() const
EntryIterator getEntriesEnd()