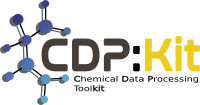 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94ANGLEBENDINGPARAMETERTABLE_HPP
30 #define CDPL_FORCEFIELD_MMFF94ANGLEBENDINGPARAMETERTABLE_HPP
35 #include <unordered_map>
39 #include <boost/iterator/transform_iterator.hpp>
57 typedef std::unordered_map<std::uint32_t, Entry> DataStorage;
60 typedef std::shared_ptr<MMFF94AngleBendingParameterTable>
SharedPointer;
68 Entry(
unsigned int angle_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom_type,
69 unsigned int term_atom2_type,
double force_const,
double ref_angle);
86 unsigned int angleTypeIdx;
87 unsigned int termAtom1Type;
88 unsigned int ctrAtomType;
89 unsigned int termAtom2Type;
95 typedef boost::transform_iterator<std::function<
const Entry&(
const DataStorage::value_type&)>,
96 DataStorage::const_iterator>
99 typedef boost::transform_iterator<std::function<
Entry&(DataStorage::value_type&)>,
100 DataStorage::iterator>
105 void addEntry(
unsigned int angle_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom_type,
106 unsigned int term_atom2_type,
double force_const,
double ref_angle);
108 const Entry&
getEntry(
unsigned int angle_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom_type,
109 unsigned int term_atom2_type)
const;
115 bool removeEntry(
unsigned int angle_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom_type,
116 unsigned int term_atom2_type);
151 #endif // CDPL_FORCEFIELD_MMFF94ANGLEBENDINGPARAMETERTABLE_HPP
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
EntryIterator removeEntry(const EntryIterator &it)
unsigned int getCenterAtomType() const
unsigned int getAngleTypeIndex() const
ConstEntryIterator getEntriesBegin() const
MMFF94AngleBendingParameterTable()
Entry(unsigned int angle_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom_type, unsigned int term_atom2_type, double force_const, double ref_angle)
boost::transform_iterator< std::function< Entry &(DataStorage::value_type &)>, DataStorage::iterator > EntryIterator
Definition: MMFF94AngleBendingParameterTable.hpp:101
Definition: MMFF94AngleBendingParameterTable.hpp:51
double getReferenceAngle() const
unsigned int getTerminalAtom2Type() const
std::shared_ptr< MMFF94AngleBendingParameterTable > SharedPointer
Definition: MMFF94AngleBendingParameterTable.hpp:60
std::size_t getNumEntries() const
double getForceConstant() const
const Entry & getEntry(unsigned int angle_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom_type, unsigned int term_atom2_type) const
EntryIterator getEntriesEnd()
ConstEntryIterator begin() const
bool removeEntry(unsigned int angle_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom_type, unsigned int term_atom2_type)
unsigned int getTerminalAtom1Type() const
EntryIterator getEntriesBegin()
The namespace of the Chemical Data Processing Library.
ConstEntryIterator end() const
static void set(const SharedPointer &table)
ConstEntryIterator getEntriesEnd() const
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition: MMFF94AngleBendingParameterTable.hpp:63
boost::transform_iterator< std::function< const Entry &(const DataStorage::value_type &)>, DataStorage::const_iterator > ConstEntryIterator
Definition: MMFF94AngleBendingParameterTable.hpp:97
void addEntry(unsigned int angle_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom_type, unsigned int term_atom2_type, double force_const, double ref_angle)
static const SharedPointer & get()
void load(std::istream &is)