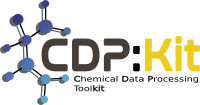 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
32 #ifndef CDPL_MOLPROP_MHMOPICHARGECALCULATOR_HPP
33 #define CDPL_MOLPROP_MHMOPICHARGECALCULATOR_HPP
60 class PEOESigmaChargeCalculator;
122 bool diagHueckelMatrix();
128 double calcElecDensity(std::size_t i, std::size_t j)
const;
134 double coeffVecIndex;
140 typedef std::vector<const Chem::Bond*> BondList;
141 typedef std::vector<std::size_t> CountsArray;
142 typedef std::vector<double> DoubleArray;
143 typedef std::vector<MODescr> MODescrArray;
144 typedef std::vector<MODescr*> MODescrPtrArray;
145 typedef std::unique_ptr<PEOESigmaChargeCalculator> PEOECalculatorPtr;
147 Matrix hueckelMatrix;
148 Matrix hmEigenVectors;
149 Vector hmEigenValues;
151 CountsArray atomPiSysCounts;
152 CountsArray atomFreeElecCounts;
153 CountsArray atomPiElecCounts;
155 MODescrArray moDescriptors;
156 MODescrPtrArray moDescriptorPtrs;
158 DoubleArray atomElecDensities;
159 DoubleArray bondElecDensities;
160 DoubleArray atomPiCharges;
162 PEOECalculatorPtr peoeCalculatorPtr;
167 #endif // CDPL_MOLPROP_MHMOPICHARGECALCULATOR_HPP
void localizedPiBonds(bool localized)
Bond.
Definition: Bond.hpp:50
MHMOPiChargeCalculator(const Chem::MolecularGraph &molgraph)
double getCharge(std::size_t atom_idx) const
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
MolecularGraph.
Definition: MolecularGraph.hpp:52
MHMOPiChargeCalculator.
Definition: MHMOPiChargeCalculator.hpp:67
Definition of the type CDPL::Util::BitSet.
Describes an electron system of a molecule in terms of involved atoms and their electron contribution...
Definition: ElectronSystem.hpp:55
double getElectronDensity(std::size_t atom_idx) const
A data type for the storage of Chem::ElectronSystem objects.
Definition: ElectronSystemList.hpp:49
Definition of the class CDPL::Chem::ElectronSystemList.
void calculate(const Chem::ElectronSystemList &pi_sys_list, const Chem::MolecularGraph &molgraph)
The namespace of the Chemical Data Processing Library.
std::shared_ptr< MHMOPiChargeCalculator > SharedPointer
Definition: MHMOPiChargeCalculator.hpp:70
Definition of the preprocessor macro CDPL_MOLPROP_API.
#define CDPL_MOLPROP_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void calculate(const Chem::MolecularGraph &molgraph)
Definition of matrix data types.
bool localizedPiBonds() const
~MHMOPiChargeCalculator()
double getBondOrder(std::size_t bond_idx) const
Definition of vector data types.
MHMOPiChargeCalculator(const Chem::ElectronSystemList &pi_sys_list, const Chem::MolecularGraph &molgraph)