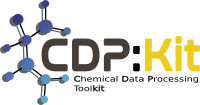 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BASE_VALUEKEY_HPP
30 #define CDPL_BASE_VALUEKEY_HPP
68 std::size_t operator()(
const LookupKey& key)
const;
92 void setName(
const std::string& name)
const;
105 std::size_t getID()
const;
133 std::size_t numericID;
148 return (numericID < key.numericID);
153 return (numericID == key.numericID);
158 return (numericID != key.numericID);
164 return key.numericID;
167 #endif // CDPL_BASE_VALUEKEY_HPP
static LookupKey create(const std::string &name)
Creates a new unique LookupKey instance and registers it under the specified name.
GridEquality< E1, E2 >::ResultType operator!=(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:346
GridEquality< E1, E2 >::ResultType operator==(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:339
void setName(const std::string &name) const
Sets the name of the LookupKey instance.
std::size_t operator()(const LookupKey &key) const
Returns the hash code of the LookupKey instance key.
Definition: LookupKey.hpp:162
A functor class implementing the generation of hash codes for LookupKey instances.
Definition: LookupKey.hpp:61
bool operator!=(const LookupKey &key) const
Inequality comparison operator.
Definition: LookupKey.hpp:156
#define CDPL_BASE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
const std::string & getName() const
Returns the name of the LookupKey instance.
An unique lookup key for control-parameter and property values.
Definition: LookupKey.hpp:54
Definition of the preprocessor macro CDPL_BASE_API.
bool operator==(const LookupKey &key) const
Equality comparison operator.
Definition: LookupKey.hpp:151
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
static const LookupKey NONE
Used to denote an invalid, unused or unspecified key.
Definition: LookupKey.hpp:74
The namespace of the Chemical Data Processing Library.
bool operator<(const LookupKey &key) const
Less than comparison operator.
Definition: LookupKey.hpp:146
std::size_t getID() const
Returns the unique numeric identifier associated with the LookupKey instance.
Definition: LookupKey.hpp:141