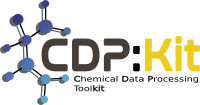 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_INDIRECTARRAY_HPP
30 #define CDPL_UTIL_INDIRECTARRAY_HPP
32 #include <type_traits>
34 #include <boost/iterator/transform_iterator.hpp>
66 template <
typename ValueType,
typename Po
interType =
typename ValueType::SharedPo
inter,
bool NullPo
interCheck = true>
70 typedef typename std::conditional<NullPointerCheck,
134 IndirectArray(std::size_t num_elem,
const PointerType& ptr = PointerType()):
143 template <
typename InputIter>
182 template <
typename InputIter>
376 virtual const char* getClassName()
const;
378 DerefFunc dereferencer;
386 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
393 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
395 const PointerType& ptr)
400 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
401 template <
typename InputIter>
403 const InputIter& last)
408 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
415 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
422 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
428 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
434 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
440 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
446 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
453 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
460 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
467 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
474 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
481 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
488 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
495 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
502 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
509 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
516 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
523 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
530 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
536 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
542 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
548 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
554 template <
typename ValueType,
typename Po
interType,
bool NullPo
interCheck>
557 return "IndirectArray";
560 #endif // CDPL_UTIL_INDIRECTARRAY_HPP
ConstReverseElementIterator getElementsReverseBegin() const
Returns a constant iterator over the pointed-to objects that points to the beginning of the reversed ...
Definition: IndirectArray.hpp:504
ReverseElementIterator getElementsReverseBegin()
Returns a mutable iterator over the pointed-to objects that points to the beginning of the reversed a...
Definition: IndirectArray.hpp:511
void insertElements(const ElementIterator &it, std::size_t num_elem, const PointerType &ptr)
Inserts num_elem copies of ptr before the location specified by the iterator it.
Definition: IndirectArray.hpp:394
ElementIterator removeElements(const ElementIterator &first, const ElementIterator &last)
Removes the elements pointed to by the iterators in the range [first, last).
Definition: Array.hpp:839
StorageType::reverse_iterator ReverseElementIterator
A mutable random access iterator used to iterate over the elements of the array in reverse order.
Definition: Array.hpp:143
void insertElements(const ElementIterator &it, const InputIter &first, const InputIter &last)
Inserts the range of pointers [first, last) before the location specified by the iterator it.
Definition: IndirectArray.hpp:402
ValueType & getElement(std::size_t idx)
Returns a non-const reference to the object pointed-to by the pointer element at index idx.
Definition: IndirectArray.hpp:537
ReverseElementIterator getElementsReverseEnd()
Returns a mutable iterator over the pointed-to objects that points to the end of the reversed array.
Definition: IndirectArray.hpp:525
const ValueType & getLastElement() const
Returns a const reference to the object pointed to by the last element of the array.
Definition: IndirectArray.hpp:429
ElementIterator end()
Returns a mutable iterator over the pointed-to objects that points to the end of the array.
Definition: IndirectArray.hpp:497
IndirectArray(std::size_t num_elem, const PointerType &ptr=PointerType())
Creates and initializes the array with num_elem copies of ptr.
Definition: IndirectArray.hpp:134
void insertElement(std::size_t idx, const ValueType &value=ValueType())
Inserts a new element before the location specified by the index idx.
Definition: Array.hpp:763
ElementIterator insertElement(const ElementIterator &it, const PointerType &ptr)
Inserts a new element before the location specified by the iterator it.
Definition: IndirectArray.hpp:388
virtual ~IndirectArray()
Virtual destructor.
Definition: IndirectArray.hpp:151
ElementIterator begin()
Returns a mutable iterator over the pointed-to objects that points to the beginning of the array.
Definition: IndirectArray.hpp:483
ConstElementIterator getElementsBegin() const
Returns a constant iterator over the pointed-to objects that points to the beginning of the array.
Definition: IndirectArray.hpp:448
void insertElements(std::size_t idx, std::size_t num_elem, const ValueType &value=ValueType())
Inserts num_elem copies of value before the location specified by the index idx.
Definition: Array.hpp:780
A dynamic array class for the storage of object pointers with an indirected query interface.
Definition: IndirectArray.hpp:68
A dynamic array class providing amortized constant time access to arbitrary elements.
Definition: Array.hpp:92
std::shared_ptr< IndirectArray > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated IndirectArray instances.
Definition: IndirectArray.hpp:90
An unary functor for the dereferenciation of pointers with null pointer checking.
Definition: Dereferencer.hpp:76
An unary functor for the dereferenciation of pointers without null pointer checking.
Definition: Dereferencer.hpp:52
ElementIterator getElementsEnd()
Returns a mutable iterator over the pointed-to objects that points to the end of the array.
Definition: IndirectArray.hpp:469
ElementIterator removeElement(const ElementIterator &it)
Removes the element at the position specified by the iterator it.
Definition: IndirectArray.hpp:410
StorageType::const_reverse_iterator ConstReverseElementIterator
A constant random access iterator used to iterate over the elements of the array in reverse order.
Definition: Array.hpp:131
Array< PointerType > BaseType
The type of the public Array<PointerType> base class storing the pointer elements.
Definition: IndirectArray.hpp:79
void insertElements(std::size_t idx, std::size_t num_elem, const ValueType &value=ValueType())
Inserts num_elem copies of value before the location specified by the index idx.
Definition: Array.hpp:780
Definition of the class CDPL::Util::Array.
boost::transform_iterator< DerefFunc, typename BaseType::ConstElementIterator, const ValueType & > ConstElementIterator
A constant random access iterator used to iterate over the pointed-to objects.
Definition: IndirectArray.hpp:98
void removeElement(std::size_t idx)
Removes the element at the position specified by the index idx.
Definition: Array.hpp:823
IndirectArray(const InputIter &first, const InputIter &last)
Creates and initializes the array with copies of the pointers in the range [first,...
Definition: IndirectArray.hpp:144
ElementIterator getElementsBegin()
Returns a mutable iterator over the pointed-to objects that points to the beginning of the array.
Definition: IndirectArray.hpp:455
ConstElementIterator getElementsEnd() const
Returns a constant iterator over the pointed-to objects that points to the end of the array.
Definition: IndirectArray.hpp:462
StorageType::const_iterator ConstElementIterator
A constant random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:125
ValueType & getLastElement()
Returns a non-const reference to the object pointed to by the last element of the array.
Definition: IndirectArray.hpp:441
IndirectArray()
Creates an empty array.
Definition: IndirectArray.hpp:126
ElementIterator removeElements(const ElementIterator &first, const ElementIterator &last)
Removes the elements pointed to by the iterators in the range [first, last).
Definition: IndirectArray.hpp:417
ValueType ElementType
The type of the object that is obtained after dereferenciation of a stored pointer element.
Definition: IndirectArray.hpp:85
boost::transform_iterator< DerefFunc, typename BaseType::ReverseElementIterator, ValueType & > ReverseElementIterator
A mutable random access iterator used to iterate over the pointed-to objects in reverse order.
Definition: IndirectArray.hpp:122
The namespace of the Chemical Data Processing Library.
boost::transform_iterator< DerefFunc, typename BaseType::ConstReverseElementIterator, const ValueType & > ConstReverseElementIterator
A constant random access iterator used to iterate over the pointed-to objects in reverse order.
Definition: IndirectArray.hpp:106
const ValueType & getElement(std::size_t idx) const
Returns a const reference to the object pointed-to by the pointer element at index idx.
Definition: IndirectArray.hpp:531
const ValueType & operator[](std::size_t idx) const
Returns a const reference to the object pointed-to by the pointer element at index idx.
Definition: IndirectArray.hpp:543
ConstElementIterator end() const
Returns a constant iterator over the pointed-to objects that points to the end of the array.
Definition: IndirectArray.hpp:490
ConstReverseElementIterator getElementsReverseEnd() const
Returns a constant iterator over the pointed-to objects that points to the end of the reversed array.
Definition: IndirectArray.hpp:518
ValueType & getFirstElement()
Returns a non-const reference to the object pointed to by the first element of the array.
Definition: IndirectArray.hpp:435
boost::transform_iterator< DerefFunc, typename BaseType::ElementIterator, ValueType & > ElementIterator
A mutable random access iterator used to iterate over the pointed-to objects.
Definition: IndirectArray.hpp:114
ConstElementIterator begin() const
Returns a constant iterator over the pointed-to objects that points to the beginning of the array.
Definition: IndirectArray.hpp:476
Definition of the classes CDPL::Util::Dereferencer and CDPL::Util::NullCheckDereferencer.
void insertElement(std::size_t idx, const ValueType &value=ValueType())
Inserts a new element before the location specified by the index idx.
Definition: Array.hpp:763
ValueType & operator[](std::size_t idx)
Returns a non-const reference to the object pointed-to by the pointer element at index idx.
Definition: IndirectArray.hpp:549
StorageType::iterator ElementIterator
A mutable random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:137
void removeElement(std::size_t idx)
Removes the element at the position specified by the index idx.
Definition: Array.hpp:823
ElementIterator removeElements(const ElementIterator &first, const ElementIterator &last)
Removes the elements pointed to by the iterators in the range [first, last).
Definition: Array.hpp:839
const ValueType & getFirstElement() const
Returns a const reference to the object pointed to by the first element of the array.
Definition: IndirectArray.hpp:423