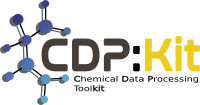 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
25 #ifndef CDPL_VIS_IMAGEWRITER_HPP
26 #define CDPL_VIS_IMAGEWRITER_HPP
57 class StructureView2D;
83 typedef std::unique_ptr<StructureView2D> StructureViewPtr;
84 typedef std::unique_ptr<ReactionView2D> ReactionViewPtr;
87 StructureViewPtr structureView;
88 ReactionViewPtr reactionView;
93 #endif // CDPL_VIS_IMAGEWRITER_HPP
virtual cairo_surface_t * createCairoSurface() const =0
The abstract base of classes implementing the 2D visualization of data objects.
Definition: View2D.hpp:59
Definition: ImageWriter.hpp:61
cairo_surface_t * renderReactionImage(const Chem::Reaction &)
struct _cairo_surface cairo_surface_t
Definition: CairoPointer.hpp:36
Provides infrastructure for the registration of I/O callback functions.
Definition: DataIOBase.hpp:59
MolecularGraph.
Definition: MolecularGraph.hpp:52
cairo_surface_t * renderMolGraphImage(const Chem::MolecularGraph &)
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
The namespace of the Chemical Data Processing Library.
Reaction.
Definition: Reaction.hpp:52
Specifies an axis aligned rectangular area in 2D space.
Definition: Rectangle2D.hpp:51
Definition of the preprocessor macro CDPL_VIS_API.
ImageWriter(const Base::DataIOBase &io_base)
virtual cairo_surface_t * createCairoSurface(double, double) const =0