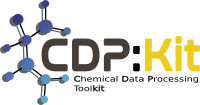 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_INCHIMOLECULEREADER_HPP
30 #define CDPL_CHEM_INCHIMOLECULEREADER_HPP
180 const std::string& getLogOutput()
const;
189 bool readData(std::istream&,
Molecule&,
bool overwrite);
190 bool skipData(std::istream&);
191 bool moreData(std::istream&);
193 bool readMolecule(std::istream&,
Molecule&);
194 bool skipMolecule(std::istream&);
213 typedef std::vector<char> StringData;
215 std::string inputToken;
216 StringData inputData;
217 StringData inchiOptions;
218 bool strictErrorChecking;
221 std::string logOutput;
226 #endif // CDPL_CHEM_INCHIMOLECULEREADER_HPP
CDPL_CHEM_API void setFormalCharge(Atom &atom, long charge)
Definition of the preprocessor macro CDPL_CHEM_API.
CDPL_CHEM_API void set3DCoordinates(Entity3DContainer &cntnr, const Math::Vector3DArray &coords)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
struct tagINCHIStereo0D inchi_Stereo0D
Definition: INCHIMolecularGraphWriter.hpp:42
CDPL_CHEM_API void setRadicalType(Atom &atom, unsigned int type)
int getReturnCode() const
Returns the error code of the last read operation.
Atom.
Definition: Atom.hpp:52
struct tagInchiAtom inchi_Atom
Definition: INCHIMolecularGraphWriter.hpp:41
A reader for molecule data in the IUPAC International Chemical Identifier (InChI) [INCHI] format.
Definition: INCHIMoleculeReader.hpp:151
Molecule.
Definition: Molecule.hpp:49
CDPL_CHEM_API void setIsotope(Atom &atom, std::size_t isotope)
const std::string & getMessage() const
Returns the error message associated with the last read operation.
INCHIMoleculeReader(std::istream &is)
Constructs a INCHIMoleculeReader instance that will read the molecule data from the input stream is.
The namespace of the Chemical Data Processing Library.
Definition of the class CDPL::Util::StreamDataReader.
A helper class that implements Base::DataReader for std::istream based data readers.
Definition: StreamDataReader.hpp:73
CDPL_CHEM_API void set2DCoordinates(AtomContainer &cntnr, const Math::Vector2DArray &coords)