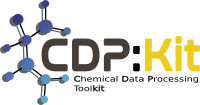 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_HYDROGEN3DCOORDINATESCALCULATOR_HPP
30 #define CDPL_CHEM_HYDROGEN3DCOORDINATESCALCULATOR_HPP
133 typedef std::vector<std::size_t> AtomIndexList;
146 void assignTemplateCoords(
const Atom&, std::size_t, std::size_t,
151 bool getConnectedAtomWithCoords(std::size_t,
const Atom&, std::size_t&)
const;
153 double getHydrogenBondLength(
const Atom&)
const;
159 void getRotationReferenceVector(
const Atom&, std::size_t, std::size_t, std::size_t,
163 typedef std::vector<Math::Vector3D> DynamicPointArray;
171 AtomIndexList centerAtoms;
172 AtomIndexList conctdAtoms;
175 DynamicPointArray genPoints;
182 #endif // CDPL_CHEM_HYDROGEN3DCOORDINATESCALCULATOR_HPP
Hydrogen3DCoordinatesCalculator()
Constructs the Hydrogen3DCoordinatesCalculator instance.
Definition of the preprocessor macro CDPL_CHEM_API.
Definition of the class CDPL::Math::VectorArray.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
void setup(const MolecularGraph &molgraph)
const Atom3DCoordinatesFunction & getAtom3DCoordinatesFunction() const
Returns the function that was registered for the retrieval of atom 3D-coordinates.
void calculate(Math::Vector3DArray &coords, bool init_coords=true)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Type definition of a generic wrapper class for storing user-defined Chem::Atom 3D-coordinates functio...
std::function< bool(const Chem::Atom &)> AtomPredicate
A generic wrapper class used to store a user-defined atom predicate.
Definition: AtomPredicate.hpp:41
void setAtom3DCoordinatesCheckFunction(const AtomPredicate &func)
Specifies a function that tells whether 3D coordinates are available for it's argument atom.
CDPL_CHEM_API unsigned int getHybridizationState(const Atom &atom)
std::size_t getConnectedAtoms(AtomType &atom, const MolecularGraph &molgraph, OutputIterator it, AtomType *excl_atom=0)
Definition: Chem/AtomFunctions.hpp:425
Type definition of a generic wrapper class for storing user-defined Chem::Atom predicates.
std::function< const Math::Vector3D &(const Chem::Atom &)> Atom3DCoordinatesFunction
A generic wrapper class used to store a user-defined Chem::Atom 3D-coordinates function.
Definition: Atom3DCoordinatesFunction.hpp:43
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
Implementation of the Kabsch algorithm.
Hydrogen3DCoordinatesCalculator(const MolecularGraph &molgraph, Math::Vector3DArray &coords, bool undef_only=true)
Constructs the Hydrogen3DCoordinatesCalculator instance and calculates 3D-coordinates for the hydroge...
bool undefinedOnly() const
Tells whether already defined hydrogen atom coordinates are recalculated or left unchanged.
The namespace of the Chemical Data Processing Library.
void undefinedOnly(bool undef_only)
Allows to specify whether already defined hydrogen atom coordinates have to be recalculated or are le...
Definition of matrix data types.
Hydrogen3DCoordinatesCalculator.
Definition: Hydrogen3DCoordinatesCalculator.hpp:57
void calculate(const MolecularGraph &molgraph, Math::Vector3DArray &coords, bool init_coords=true)
Calculates 3D-coordinates for the hydrogen atoms of the molecular graph molgraph.
const AtomPredicate & getAtom3DCoordinatesCheckFunction() const
Returns the function that was registered to determine whether for a given atom 3D coordinates are ava...
void setAtom3DCoordinatesFunction(const Atom3DCoordinatesFunction &func)
Specifies a function for the retrieval of atom 3D-coordinates.