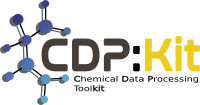 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_HASHCODECALCULATOR_HPP
30 #define CDPL_CHEM_HASHCODECALCULATOR_HPP
64 static constexpr
unsigned int DEF_ATOM_PROPERTY_FLAGS =
73 static constexpr
unsigned int DEF_BOND_PROPERTY_FLAGS =
100 calculator(calculator), flags(flags) {}
114 std::uint64_t getAtomTypeHashSeed(
const Atom&)
const;
115 std::uint64_t getAtomIsotopeHashSeed(
const Atom&)
const;
116 std::uint64_t getAtomChargeHashSeed(
const Atom&)
const;
117 std::uint64_t getAtomHCountHashSeed(
const Atom&)
const;
118 std::uint64_t getAtomConfigHashSeed(
const Atom&)
const;
119 std::uint64_t getAtomAromaticityHashSeed(
const Atom&)
const;
159 std::uint64_t getBondTypeHashSeed(
const Bond&)
const;
160 std::uint64_t getBondConfigHashSeed(
const Bond&)
const;
161 std::uint64_t getBondTopologyHashSeed(
const Bond&)
const;
235 void calcAtomHashCodes();
236 void calcBondHashCodes();
237 void calcSHAHashCode();
239 typedef std::vector<std::uint64_t> UInt64Array;
240 typedef std::vector<std::size_t> IndexList;
245 UInt64Array atomHashCodes;
246 UInt64Array bondHashCodes;
247 UInt64Array tmpHashCodes1;
248 UInt64Array tmpHashCodes2;
249 UInt64Array shaInput;
250 std::uint8_t shaHashCode[20];
255 #endif // CDPL_CHEM_HASHCODECALCULATOR_HPP
const unsigned int FORMAL_CHARGE
Specifies the formal charge of an atom.
Definition: Chem/AtomPropertyFlag.hpp:73
const unsigned int AROMATICITY
Specifies the membership of a bond in aromatic rings.
Definition: BondPropertyFlag.hpp:73
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
std::uint64_t operator()(const Bond &bond) const
Generates an initial hash code value (seed) for the specified bond.
Atom.
Definition: Atom.hpp:52
const unsigned int CIP_CONFIGURATION
Specifies the CIP-configuration of a double bond.
Definition: BondPropertyFlag.hpp:58
DefBondHashSeedFunctor(unsigned int flags=DEF_BOND_PROPERTY_FLAGS)
Constructs the bond hash seed functor object for the specified set of bond properties.
Definition: HashCodeCalculator.hpp:144
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
std::uint64_t getResult() const
Returns the result of the last hash code calculation.
std::uint64_t calculate(const MolecularGraph &molgraph)
Calculates the hash code of the molecular graph molgraph.
void setAtomHashSeedFunction(const AtomHashSeedFunction &func)
Allows to specify a custom function for the generation of initial atom hash codes.
HashCodeCalculator(const MolecularGraph &molgraph)
Constructs the HashCodeCalculator instance and calculates the hash code of the molecular graph molgra...
std::function< std::uint64_t(const Bond &)> BondHashSeedFunction
Type of the generic functor class used to store user-defined functions or function objects for the ge...
Definition: HashCodeCalculator.hpp:184
const unsigned int ORDER
Specifies the order of a bond.
Definition: BondPropertyFlag.hpp:63
DefAtomHashSeedFunctor(const HashCodeCalculator &calculator, unsigned int flags=DEF_ATOM_PROPERTY_FLAGS)
Constructs the atom hash seed functor object for the specified set of atomic properties.
Definition: HashCodeCalculator.hpp:99
The default functor for the generation of bond hash seeds.
Definition: HashCodeCalculator.hpp:129
const unsigned int TOPOLOGY
Specifies the ring/chain topology of a bond.
Definition: BondPropertyFlag.hpp:68
const unsigned int TYPE
Specifies the generic type or element of an atom.
Definition: Chem/AtomPropertyFlag.hpp:63
const unsigned int ISOTOPE
Specifies the isotopic mass of an atom.
Definition: Chem/AtomPropertyFlag.hpp:68
The default functor for the generation of atom hash seeds.
Definition: HashCodeCalculator.hpp:81
The namespace of the Chemical Data Processing Library.
const unsigned int CIP_CONFIGURATION
Specifies the CIP-configuration of a chiral atom.
Definition: Chem/AtomPropertyFlag.hpp:58
HashCodeCalculator()
Constructs the HashCodeCalculator instance.
const unsigned int AROMATICITY
Specifies the membership of an atom in aromatic rings.
Definition: Chem/AtomPropertyFlag.hpp:93
std::function< std::uint64_t(const Atom &)> AtomHashSeedFunction
Type of the generic functor class used to store user-defined functions or function objects for the ge...
Definition: HashCodeCalculator.hpp:174
HashCodeCalculator.
Definition: HashCodeCalculator.hpp:57
const unsigned int H_COUNT
Specifies the hydrogen count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:78
Definition of constants in namespace CDPL::Chem::BondPropertyFlag.
std::uint64_t operator()(const Atom &atom) const
Generates an initial hash code value (seed) for the specified atom.
void setBondHashSeedFunction(const BondHashSeedFunction &func)
Allows to specify a custom function for the generation of initial bond hash codes.