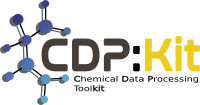 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_GAUSSIANSHAPE_HPP
30 #define CDPL_SHAPE_GAUSSIANSHAPE_HPP
59 position(pos), radius(radius), hardness(hardness), color(color) {}
78 this->radius = radius;
98 this->hardness = hardness;
109 typedef std::vector<Element> ElementList;
148 ElementList elements;
153 #endif // CDPL_SHAPE_GAUSSIANSHAPE_HPP
Definition: GaussianShape.hpp:55
void addElement(const Math::Vector3D &pos, double radius, std::size_t color=0, double hardness=2.7)
std::size_t getNumElements() const
ElementIterator getElementsEnd()
ConstElementIterator begin() const
Element & getElement(std::size_t idx)
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
void setRadius(double radius)
Definition: GaussianShape.hpp:76
void setColor(std::size_t color)
Definition: GaussianShape.hpp:86
void setPosition(const Math::Vector3D &pos)
Definition: GaussianShape.hpp:66
const Element & getElement(std::size_t idx) const
double getRadius() const
Definition: GaussianShape.hpp:71
std::shared_ptr< GaussianShape > SharedPointer
Definition: GaussianShape.hpp:112
A data type for the descripton of arbitrary shapes composed of spheres approximated by gaussian funct...
Definition: GaussianShape.hpp:51
ConstElementIterator end() const
ConstElementIterator getElementsEnd() const
void removeElement(std::size_t idx)
double getHardness() const
Definition: GaussianShape.hpp:91
ElementList::iterator ElementIterator
Definition: GaussianShape.hpp:115
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void addElement(const Element &elem)
A class providing methods for the storage and lookup of object properties.
Definition: PropertyContainer.hpp:75
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
const Math::Vector3D & getPosition() const
Definition: GaussianShape.hpp:61
ElementList::const_iterator ConstElementIterator
Definition: GaussianShape.hpp:114
std::size_t getColor() const
Definition: GaussianShape.hpp:81
Element(const Math::Vector3D &pos, double radius, std::size_t color=0, double hardness=2.7)
Definition: GaussianShape.hpp:58
void setHardness(double hardness)
Definition: GaussianShape.hpp:96
ConstElementIterator getElementsBegin() const
Definition of the class CDPL::Base::PropertyContainer.
ElementIterator getElementsBegin()
Definition of vector data types.