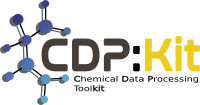 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_GAUSSIANSHAPEGENERATOR_HPP
30 #define CDPL_SHAPE_GAUSSIANSHAPEGENERATOR_HPP
106 typedef std::vector<const Chem::Atom*> AtomList;
108 template <
typename CoordsFunc>
112 template <
typename CoordsFunc>
113 void createShape(
const CoordsFunc& coords_func,
GaussianShape& shape)
const;
132 #endif // CDPL_SHAPE_GAUSSIANSHAPEGENERATOR_HPP
Definition: GaussianShapeSet.hpp:46
void setAtomRadius(double radius)
Definition of the class CDPL::Pharm::BasicPharmacophore.
Definition of the class CDPL::Shape::GaussianShapeSet.
bool includeHydrogens() const
MolecularGraph.
Definition: MolecularGraph.hpp:52
void setFeatureRadius(double radius)
std::shared_ptr< GaussianShapeGenerator > SharedPointer
Definition: GaussianShapeGenerator.hpp:57
Pharm::PharmacophoreGenerator & getPharmacophoreGenerator() const
void multiConformerMode(bool multi_conf)
Pharm::DefaultPharmacophoreGenerator & getDefaultPharmacophoreGenerator()
A data type for the descripton of arbitrary shapes composed of spheres approximated by gaussian funct...
Definition: GaussianShape.hpp:51
const GaussianShapeSet & generate(const Chem::MolecularGraph &molgraph)
bool generateMoleculeShape() const
const GaussianShapeSet & getShapes() const
void setAtomHardness(double hardness)
void includeHydrogens(bool include)
BasicPharmacophore.
Definition: BasicPharmacophore.hpp:52
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
PharmacophoreGenerator.
Definition: PharmacophoreGenerator.hpp:49
void setPharmacophoreGenerator(Pharm::PharmacophoreGenerator &gen)
Definition of the class CDPL::Pharm::DefaultPharmacophoreGenerator.
double getFeatureRadius() const
void generateMoleculeShape(bool generate)
void setFeatureHardness(double hardness)
DefaultPharmacophoreGenerator.
Definition: DefaultPharmacophoreGenerator.hpp:48
void generatePharmacophoreShape(bool generate)
bool multiConformerMode() const
bool generatePharmacophoreShape() const
const Pharm::DefaultPharmacophoreGenerator & getDefaultPharmacophoreGenerator() const
Definition: GaussianShapeGenerator.hpp:54
double getFeatureHardness()
double getAtomRadius() const