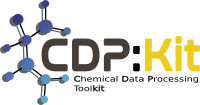 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_GAUSSIANSHAPEFUNCTION_HPP
30 #define CDPL_SHAPE_GAUSSIANSHAPEFUNCTION_HPP
48 class GaussianProductList;
49 class ExactGaussianShapeOverlapFunction;
50 class FastGaussianShapeOverlapFunction;
56 static constexpr std::size_t DEF_MAX_PRODUCT_ORDER = 6;
57 static constexpr
double DEF_DISTANCE_CUTOFF = 0.0;
107 const GaussianProductList* getProductList()
const;
109 typedef std::unique_ptr<GaussianProductList> ProductListPtr;
113 ProductListPtr prodList;
118 #endif // CDPL_SHAPE_GAUSSIANSHAPEFUNCTION_HPP
void calcCentroid(Math::Vector3D &ctr) const
Definition of the class CDPL::Math::VectorArray.
double calcDensity(const Math::Vector3D &pos) const
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
double getDistanceCutoff() const
GaussianShapeFunction(const GaussianShapeFunction &func)
GaussianShapeFunction(const GaussianShape &shape)
GaussianShapeFunction & operator=(const GaussianShapeFunction &func)
std::shared_ptr< GaussianShapeFunction > SharedPointer
Definition: GaussianShapeFunction.hpp:59
std::size_t getMaxOrder() const
double calcVolume() const
A data type for the descripton of arbitrary shapes composed of spheres approximated by gaussian funct...
Definition: GaussianShape.hpp:51
void getElementPositions(Math::Vector3DArray &coords) const
void transform(const Math::Matrix4D &xform)
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
void calcQuadrupoleTensor(const Math::Vector3D &ctr, Math::Matrix3D &quad_tensor) const
Definition: GaussianShapeFunction.hpp:53
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
double calcSurfaceArea() const
Definition: FastGaussianShapeOverlapFunction.hpp:47
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
void setDistanceCutoff(double cutoff)
double calcSurfaceArea(std::size_t elem_idx) const
Definition of matrix data types.
void setMaxOrder(std::size_t max_order)
Definition: ExactGaussianShapeOverlapFunction.hpp:47
const GaussianShape * getShape() const
void setShape(const GaussianShape &shape)
Definition of vector data types.
const Math::Vector3D & getElementPosition(std::size_t idx) const