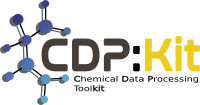 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_GAUSSIANSHAPEFUNCTIONALIGNMENT_HPP
30 #define CDPL_SHAPE_GAUSSIANSHAPEFUNCTIONALIGNMENT_HPP
58 typedef std::vector<Result> ResultList;
61 static constexpr
double DEF_OPTIMIZATION_STOP_GRADIENT = 1.0;
62 static constexpr std::size_t DEF_MAX_OPTIMIZATION_ITERATIONS = 20;
76 transform(xform), overlap(overlap), colOverlap(col_overlap) {}
97 transform(), overlap(0.0), colOverlap(0.0) {}
201 unsigned int refShapeSymClass;
203 bool calcColOverlaps;
206 std::size_t maxNumOptIters;
217 #endif // CDPL_SHAPE_GAUSSIANSHAPEFUNCTIONALIGNMENT_HPP
double calcSelfOverlap(const GaussianShapeFunction &func)
Definition: GaussianShapeAlignmentStartGenerator.hpp:49
bool align(const GaussianShapeFunction &func, unsigned int sym_class)
Definition: GaussianShapeFunctionAlignment.hpp:72
void performAlignment(bool perf_align)
Definition of the class CDPL::Math::VectorArray.
double getOverlap() const
Definition: GaussianShapeFunctionAlignment.hpp:83
void setMaxNumOptimizationIterations(std::size_t max_iter)
Definition: GaussianShapeFunctionAlignment.hpp:52
double getColorOverlap() const
Definition: GaussianShapeFunctionAlignment.hpp:88
Definition: PrincipalAxesAlignmentStartGenerator.hpp:49
GaussianShapeFunctionAlignment(const GaussianShapeFunction &ref_func, unsigned int sym_class)
FastGaussianShapeOverlapFunction & getDefaultOverlapFunction()
PrincipalAxesAlignmentStartGenerator & getDefaultStartGenerator()
CDPL_SHAPE_API void transform(GaussianShape &shape, const Math::Matrix4D &xform)
void setColorMatchFunction(const ColorMatchFunction &func)
void optimizeOverlap(bool optimize)
bool greedyOptimization() const
void setOptimizationStopGradient(double grad_norm)
std::function< bool(std::size_t, std::size_t)> ColorMatchFunction
Definition: GaussianShapeOverlapFunction.hpp:55
GaussianShapeAlignmentStartGenerator & getStartGenerator() const
~GaussianShapeFunctionAlignment()
Implementation of the BFGS optimization algorithm.
Definition of the class CDPL::Shape::PrincipalAxesAlignmentStartGenerator.
GaussianShapeOverlapFunction::ColorFilterFunction ColorFilterFunction
Definition: GaussianShapeFunctionAlignment.hpp:68
void calcColorOverlaps(bool calc)
const ColorMatchFunction & getColorMatchFunction() const
const GaussianShapeFunction * getReference() const
bool calcColorOverlaps() const
ResultList::const_iterator ConstResultIterator
Definition: GaussianShapeFunctionAlignment.hpp:66
std::size_t getMaxNumOptimizationIterations() const
Definition: Vector.hpp:1053
void greedyOptimization(bool greedy)
GaussianShapeOverlapFunction & getOverlapFunction() const
bool performAlignment() const
void setColorFilterFunction(const ColorFilterFunction &func)
double getOptimizationStopGradient() const
Definition: GaussianShapeOverlapFunction.hpp:49
unsigned int setupReference(GaussianShapeFunction &func, Math::Matrix4D &xform) const
Definition: GaussianShapeFunction.hpp:53
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
ConstResultIterator begin() const
void setStartGenerator(GaussianShapeAlignmentStartGenerator &gen)
Result(const Math::Matrix4D &xform, double overlap, double col_overlap)
Definition: GaussianShapeFunctionAlignment.hpp:75
Definition: FastGaussianShapeOverlapFunction.hpp:47
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
void setReference(const GaussianShapeFunction &func, unsigned int sym_class)
GaussianShapeFunctionAlignment()
Definition of the class CDPL::Shape::FastGaussianShapeOverlapFunction.
double calcColorSelfOverlap(const GaussianShapeFunction &func)
ConstResultIterator getResultsEnd() const
const PrincipalAxesAlignmentStartGenerator & getDefaultStartGenerator() const
unsigned int setupAligned(GaussianShapeFunction &func, Math::Matrix4D &xform) const
std::size_t getNumResults() const
GaussianShapeOverlapFunction::ColorMatchFunction ColorMatchFunction
Definition: GaussianShapeFunctionAlignment.hpp:69
Definition of matrix data types.
ConstResultIterator getResultsBegin() const
std::shared_ptr< GaussianShapeFunctionAlignment > SharedPointer
Definition: GaussianShapeFunctionAlignment.hpp:64
const Math::Matrix4D & getTransform() const
Definition: GaussianShapeFunctionAlignment.hpp:78
std::function< bool(std::size_t)> ColorFilterFunction
Definition: GaussianShapeOverlapFunction.hpp:54
const FastGaussianShapeOverlapFunction & getDefaultOverlapFunction() const
ConstResultIterator end() const
void setOverlapFunction(GaussianShapeOverlapFunction &func)
const ColorFilterFunction & getColorFilterFunction() const
const Result & getResult(std::size_t idx) const
bool optimizeOverlap() const