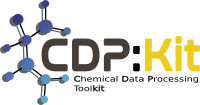 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_GAUSSIANSHAPEALIGNMENT_HPP
30 #define CDPL_SHAPE_GAUSSIANSHAPEALIGNMENT_HPP
35 #include <unordered_map>
39 #include <boost/functional/hash.hpp>
40 #include <boost/iterator/transform_iterator.hpp>
61 typedef std::vector<AlignmentResult> ResultList;
62 typedef std::vector<GaussianShapeFunction*> ShapeFunctionList;
67 static constexpr
double DEF_OPTIMIZATION_STOP_GRADIENT = 1.0;
68 static constexpr std::size_t DEF_MAX_OPTIMIZATION_ITERATIONS = 20;
69 static constexpr std::size_t DEF_MAX_PRODUCT_ORDER = 1;
71 static constexpr
double DEF_DISTANCE_CUTOFF = 0.0;
77 typedef boost::transform_iterator<GetShapeFunction, ShapeFunctionList::const_iterator>
ConstShapeIterator;
206 std::size_t setIndex;
208 unsigned int symClass;
211 double colSelfOverlap;
212 GaussianShapePtr shape;
215 typedef std::pair<std::size_t, std::size_t> ResultID;
223 void processResults(std::size_t ref_idx, std::size_t al_idx);
225 bool getResultIndex(
const ResultID& res_id, std::size_t& res_idx);
230 typedef std::vector<ShapeMetaData> ShapeMetaDataArray;
231 typedef std::unordered_map<ResultID, std::size_t, boost::hash<ResultID> > ResultIndexMap;
233 ShapeFunctionCache shapeFuncCache;
234 bool calcSlfOverlaps;
235 bool calcColSlfOverlaps;
236 unsigned int resultSelMode;
237 ResultCompareFunction resultCmpFunc;
238 ScoringFunction scoringFunc;
240 ShapeFunctionList refShapeFuncs;
241 ShapeMetaDataArray refShapeMetaData;
243 ShapeMetaData algdShapeMetaData;
244 ResultIndexMap resIndexMap;
246 std::size_t currSetIndex;
247 std::size_t currShapeIndex;
252 #endif // CDPL_SHAPE_GAUSSIANSHAPEALIGNMENT_HPP
void setMaxOrder(std::size_t max_order)
ResultList::const_iterator ConstResultIterator
Definition: GaussianShapeAlignment.hpp:75
const ScoringFunction & getScoringFunction() const
Definition: GaussianShapeAlignmentStartGenerator.hpp:49
Definition of the class CDPL::Util::ObjectStack.
GaussianShapeFunctionAlignment::ColorFilterFunction ColorFilterFunction
Definition: GaussianShapeAlignment.hpp:79
Definition: GaussianShapeSet.hpp:46
const PrincipalAxesAlignmentStartGenerator & getDefaultStartGenerator() const
ConstResultIterator getResultsEnd() const
void setResultCompareFunction(const ResultCompareFunction &func)
AlignmentResult & getResult(std::size_t idx)
void addReferenceShape(const GaussianShape &shape, bool new_set=true)
Definition: GaussianShapeFunctionAlignment.hpp:52
void clearReferenceShapes()
const GaussianShape & getReferenceShape(std::size_t idx) const
Definition: PrincipalAxesAlignmentStartGenerator.hpp:49
std::size_t getNumReferenceShapes() const
Definition of the class CDPL::Shape::AlignmentResult.
void calcSelfOverlaps(bool calc)
CDPL_SHAPE_API void transform(GaussianShape &shape, const Math::Matrix4D &xform)
Definition of the class CDPL::Shape::GaussianShapeFunctionAlignment.
const ResultCompareFunction & getResultCompareFunction() const
~GaussianShapeAlignment()
const unsigned int BEST_PER_REFERENCE_SET
Definition: AlignmentResultSelectionMode.hpp:45
void setColorFilterFunction(const ColorFilterFunction &func)
const ColorFilterFunction & getColorFilterFunction() const
bool optimizeOverlap() const
void calcColorSelfOverlaps(bool calc)
void performAlignment(bool perf_align)
void setStartGenerator(GaussianShapeAlignmentStartGenerator &gen)
GaussianShapeFunctionAlignment::ColorMatchFunction ColorMatchFunction
Definition: GaussianShapeAlignment.hpp:80
void calcColorOverlaps(bool calc)
double getDistanceCutoff() const
const ColorMatchFunction & getColorMatchFunction() const
void setOverlapFunction(GaussianShapeOverlapFunction &func)
std::size_t getMaxOrder() const
Definition of the class CDPL::Shape::GaussianShapeSet.
Definition of constants in namespace CDPL::Shape::AlignmentResultSelectionMode.
ConstShapeIterator getReferenceShapesBegin() const
GaussianShapeOverlapFunction::ColorFilterFunction ColorFilterFunction
Definition: GaussianShapeFunctionAlignment.hpp:68
std::shared_ptr< GaussianShape > SharedPointer
Definition: GaussianShape.hpp:112
bool calcColorSelfOverlaps() const
void setMaxNumOptimizationIterations(std::size_t max_iter)
void greedyOptimization(bool greedy)
GaussianShapeAlignment(const GaussianShapeSet &ref_shapes)
Definition: GaussianShapeAlignment.hpp:59
double getOptimizationStopGradient() const
std::function< bool(const AlignmentResult &, const AlignmentResult &)> ResultCompareFunction
Definition: GaussianShapeAlignment.hpp:82
Definition of the class CDPL::Shape::GaussianShapeFunction.
GaussianShapeAlignmentStartGenerator & getStartGenerator() const
A data type for the descripton of arbitrary shapes composed of spheres approximated by gaussian funct...
Definition: GaussianShape.hpp:51
void setOptimizationStopGradient(double grad_norm)
std::shared_ptr< GaussianShapeAlignment > SharedPointer
Definition: GaussianShapeAlignment.hpp:73
ResultList::iterator ResultIterator
Definition: GaussianShapeAlignment.hpp:76
bool align(const GaussianShape &shape)
PrincipalAxesAlignmentStartGenerator & getDefaultStartGenerator()
const AlignmentResult & getResult(std::size_t idx) const
void setResultSelectionMode(unsigned int mode)
bool align(const GaussianShapeSet &shapes)
void setScoringFunction(const ScoringFunction &func)
Definition: GaussianShapeOverlapFunction.hpp:49
Definition: GaussianShapeFunction.hpp:53
ResultIterator getResultsEnd()
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setColorMatchFunction(const ColorMatchFunction &func)
void optimizeOverlap(bool optimize)
FastGaussianShapeOverlapFunction & getDefaultOverlapFunction()
boost::transform_iterator< GetShapeFunction, ShapeFunctionList::const_iterator > ConstShapeIterator
Definition: GaussianShapeAlignment.hpp:77
std::function< double(const AlignmentResult &)> ScoringFunction
Definition: GaussianShapeAlignment.hpp:81
Definition: FastGaussianShapeOverlapFunction.hpp:47
The namespace of the Chemical Data Processing Library.
bool performAlignment() const
Definition of the preprocessor macro CDPL_SHAPE_API.
std::size_t getMaxNumOptimizationIterations() const
void addReferenceShapes(const GaussianShapeSet &shapes, bool new_set=true)
GaussianShapeOverlapFunction & getOverlapFunction() const
Definition: AlignmentResult.hpp:45
unsigned int getResultSelectionMode() const
bool greedyOptimization() const
bool calcSelfOverlaps() const
GaussianShapeOverlapFunction::ColorMatchFunction ColorMatchFunction
Definition: GaussianShapeFunctionAlignment.hpp:69
ConstResultIterator getResultsBegin() const
Definition of matrix data types.
void setDistanceCutoff(double cutoff)
bool calcColorOverlaps() const
ResultIterator getResultsBegin()
ConstShapeIterator getReferenceShapesEnd() const
GaussianShapeAlignment(const GaussianShape &ref_shape)
const FastGaussianShapeOverlapFunction & getDefaultOverlapFunction() const
std::size_t getNumResults() const