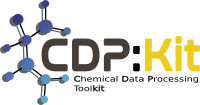 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_GAUSSIANSHAPEALIGNMENTSTARTGENERATOR_HPP
30 #define CDPL_SHAPE_GAUSSIANSHAPEALIGNMENTSTARTGENERATOR_HPP
46 class GaussianShapeFunction;
71 #endif // CDPL_SHAPE_GAUSSIANSHAPEALIGNMENTSTARTGENERATOR_HPP
Definition: GaussianShapeAlignmentStartGenerator.hpp:49
virtual ~GaussianShapeAlignmentStartGenerator()
Definition: GaussianShapeAlignmentStartGenerator.hpp:52
virtual bool generate(const GaussianShapeFunction &func, unsigned int sym_class)=0
virtual std::size_t getNumStartSubTransforms() const =0
virtual const QuaternionTransformation & getStartTransform(std::size_t idx) const =0
Definition: Vector.hpp:1053
virtual std::size_t getNumStartTransforms() const =0
Definition: GaussianShapeFunction.hpp:53
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
virtual void setReference(const GaussianShapeFunction &ref_shape_func, unsigned int sym_class)=0
virtual unsigned int setupAligned(GaussianShapeFunction &func, Math::Matrix4D &xform) const
Definition of matrix data types.
virtual unsigned int setupReference(GaussianShapeFunction &func, Math::Matrix4D &xform) const