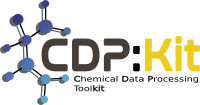 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_GRAIL_GRAILDATASETGENERATOR_HPP
30 #define CDPL_GRAIL_GRAILDATASETGENERATOR_HPP
71 static constexpr
double DEF_GRID_STEP_SIZE = 0.5;
89 void setGridName(
unsigned int ftr_type,
unsigned int tgt_ftr_type,
const std::string& name);
91 const std::string&
getGridName(
unsigned int ftr_type,
unsigned int tgt_ftr_type)
const;
117 template <
typename T>
120 gridTransform = xform;
154 const std::string& grid_name);
161 typedef std::pair<unsigned int, unsigned int> FeatureTypePair;
162 typedef std::map<FeatureTypePair, ScoringFunction> ScoringFuncMap;
163 typedef std::map<FeatureTypePair, std::string> GridNameMap;
164 typedef std::set<FeatureTypePair> EnabledInteractionsSet;
166 ScoringFuncMap ftrInteractionScoringFuncMap;
167 GridNameMap gridNameMap;
168 EnabledInteractionsSet enabledFtrInteractions;
175 std::size_t gridXSize;
176 std::size_t gridYSize;
177 std::size_t gridZSize;
179 bool diminishByAtomDensity;
180 bool storeEnvAtomDensityGrid;
181 std::string envAtomDensityGridName;
186 #endif // CDPL_GRAIL_GRAILDATASETGENERATOR_HPP
void setGridName(unsigned int ftr_type, unsigned int tgt_ftr_type, const std::string &name)
const ScoringFunction & getScoringFunction(unsigned int ftr_type, unsigned int tgt_ftr_type) const
void setPharmacophoreProcessingFunction(const PharmacophoreProcessingFunction &func)
const ScoreCombinationFunction & getScoreCombinationFunction() const
virtual ~GRAILDataSetGenerator()
Definition: GRAILDataSetGenerator.hpp:75
const Math::Matrix4D & getGridTransform() const
Grid::DRegularGrid::SharedPointer calcAtomDensityGrid(const Chem::AtomContainer &atoms, const Chem::Atom3DCoordinatesFunction &coords_func, const std::string &grid_name)
std::size_t getGridYSize() const
void enableInteraction(unsigned int ftr_type, unsigned int tgt_ftr_type, bool enable)
const std::string & getGridName(unsigned int ftr_type, unsigned int tgt_ftr_type) const
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
Definition of the class CDPL::GRAIL::FeatureInteractionScoreGridCalculator.
void calcInteractionGrids(const Chem::MolecularGraph &tgt_env, const Chem::Atom3DCoordinatesFunction &coords_func, Grid::DRegularGridSet &grid_set, bool append=false)
bool isInteractionEnabled(unsigned int ftr_type, unsigned int tgt_ftr_type) const
void setGridParamsForBoundingBox(const Math::Vector3D &bbox_min, const Math::Vector3D &bbox_max, double step_size=DEF_GRID_STEP_SIZE)
bool scoresNormalized() const
void clearEnabledInteractions()
double getGridStepSize() const
void setGridXSize(std::size_t size)
AtomDensityGridCalculator.
Definition: AtomDensityGridCalculator.hpp:69
Definition of the class CDPL::Pharm::BasicPharmacophore.
void removeScoringFunction(unsigned int ftr_type, unsigned int tgt_ftr_type)
std::size_t getGridZSize() const
std::function< double(const Math::Vector3D &, const Pharm::Feature &)> ScoringFunction
Definition: FeatureInteractionScoreGridCalculator.hpp:75
const std::string & getEnvironmentAtomDensityGridName() const
Definition of the class CDPL::Grid::RegularGridSet.
FeatureInteractionScoreGridCalculator::ScoreCombinationFunction ScoreCombinationFunction
Definition: GRAILDataSetGenerator.hpp:67
void setScoreCombinationFunction(const ScoreCombinationFunction &func)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Type definition of a generic wrapper class for storing user-defined Chem::Atom 3D-coordinates functio...
A common interface for data-structures that support a random access to stored Chem::Atom instances.
Definition: AtomContainer.hpp:55
bool scoresDiminishedByAtomDensity() const
void normalizeScores(bool normalize)
void setGridYSize(std::size_t size)
GRAILDataSetGenerator.
Definition: GRAILDataSetGenerator.hpp:61
void setScoringFunction(unsigned int ftr_type, unsigned int tgt_ftr_type, const ScoringFunction &func)
FeatureInteractionScoreGridCalculator::ScoringFunction ScoringFunction
Definition: GRAILDataSetGenerator.hpp:66
Pharmacophore.
Definition: Pharmacophore.hpp:48
void setGridZSize(std::size_t size)
Definition of the preprocessor macro CDPL_GRAIL_API.
Definition of the class CDPL::GRAIL::AtomDensityGridCalculator.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
const Pharm::DefaultPharmacophoreGenerator & getPharmacophoreGenerator() const
std::function< const Math::Vector3D &(const Chem::Atom &)> Atom3DCoordinatesFunction
A generic wrapper class used to store a user-defined Chem::Atom 3D-coordinates function.
Definition: Atom3DCoordinatesFunction.hpp:43
BasicPharmacophore.
Definition: BasicPharmacophore.hpp:52
std::function< void(CDPL::Pharm::Pharmacophore &)> PharmacophoreProcessingFunction
Definition: GRAILDataSetGenerator.hpp:69
void setGridStepSize(double size)
The namespace of the Chemical Data Processing Library.
std::size_t getGridXSize() const
Definition of the class CDPL::Pharm::DefaultPharmacophoreGenerator.
void setGridTransform(const T &xform)
Definition: GRAILDataSetGenerator.hpp:118
std::shared_ptr< RegularGrid > SharedPointer
Definition: RegularGrid.hpp:51
Pharm::DefaultPharmacophoreGenerator & getPharmacophoreGenerator()
std::shared_ptr< GRAILDataSetGenerator > SharedPointer
Definition: GRAILDataSetGenerator.hpp:64
bool environmentAtomDensityGridStored() const
DefaultPharmacophoreGenerator.
Definition: DefaultPharmacophoreGenerator.hpp:48
void setEnvironmentAtomDensityGridName(const std::string &name)
void removeGridName(unsigned int ftr_type, unsigned int tgt_ftr_type)
Definition of matrix data types.
void diminishScoresByAtomDensity(bool diminish)
FeatureInteractionScoreGridCalculator.
Definition: FeatureInteractionScoreGridCalculator.hpp:67
#define CDPL_GRAIL_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::function< double(const Math::DVector &)> ScoreCombinationFunction
Definition: FeatureInteractionScoreGridCalculator.hpp:76
const PharmacophoreProcessingFunction & getPharmacophoreProcessingFunction() const
RegularGridSet.
Definition: RegularGridSet.hpp:47
void storeEnvironmentAtomDensityGrid(bool store)
Definition of vector data types.