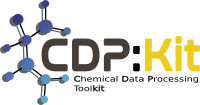 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_FUNCTIONAL_HPP
28 #define CDPL_MATH_FUNCTIONAL_HPP
30 #include <boost/algorithm/clamp.hpp>
45 class VectorExpression;
47 class MatrixExpression;
49 class QuaternionExpression;
53 template <
typename T1,
typename T2>
61 template <
typename T1,
typename T2>
74 template <
typename T1,
typename T2>
87 template <
typename T1,
typename T2>
100 template <
typename T1,
typename T2>
113 template <
typename T1,
typename T2>
126 template <
typename T>
135 template <
typename T>
149 template <
typename T>
163 template <
typename T>
172 template <
typename T>
186 template <
typename T>
200 template <
typename T1,
typename T2>
209 template <
typename T1,
typename T2>
223 template <
typename T1,
typename T2>
237 template <
typename T1,
typename T2>
251 template <
typename T1,
typename T2>
265 template <
typename V1,
typename V2>
272 template <
typename V1,
typename V2>
285 for (SizeType i = 0; i < size; i++)
286 res += e1()(i) * e2()(i);
292 template <
typename V1,
typename V2,
typename T>
309 template <
typename V1,
typename V2>
318 template <
typename V1,
typename V2>
331 for (
SizeType i = 0, size = e1().getSize(); i < size; i++)
339 template <
typename V1,
typename V2,
typename T>
349 template <
typename V1,
typename V2,
typename T>
365 ComparisonType norm_inf_max(epsilon);
367 for (
SizeType i = 0, size = e1().getSize(); i < size; i++)
375 template <
typename V1,
typename V2>
382 template <
typename V1,
typename V2>
388 template <
typename E1,
typename E2,
typename SizeType>
397 return (e1()(1) * e2()(2) - e1()(2) * e2()(1));
400 return (e1()(2) * e2()(0) - e1()(0) * e2()(2));
403 return (e1()(0) * e2()(1) - e1()(1) * e2()(0));
411 template <
typename V>
419 template <
typename V>
427 typedef typename V::SizeType
SizeType;
431 for (
SizeType i = 0, size = e().getSize(); i < size; i++)
438 template <
typename V>
447 template <
typename V>
457 typedef typename V::SizeType SizeType;
461 for (SizeType i = 0, size = e().getSize(); i < size; i++)
468 template <
typename V>
478 typedef typename V::SizeType SizeType;
482 for (SizeType i = 0, size = e().getSize(); i < size; i++) {
492 template <
typename V>
502 typedef typename V::SizeType SizeType;
506 for (SizeType i = 0, size = e().getSize(); i < size; i++) {
517 template <
typename V>
526 template <
typename V>
536 typedef typename V::SizeType SizeType;
541 for (SizeType i = 0, size = e().getSize(); i < size; i++) {
554 template <
typename M1,
typename M2>
563 template <
typename M1,
typename M2>
579 for (
SizeType i = 0, size1 = e1().getSize1(); i < size1; i++)
580 for (
SizeType j = 0, size2 = e1().getSize2(); j < size2; j++)
588 template <
typename M1,
typename M2,
typename T>
598 template <
typename M1,
typename M2,
typename T>
617 ComparisonType norm_inf_max(epsilon);
619 for (
SizeType i = 0, size1 = e1().getSize1(); i < size1; i++)
620 for (
SizeType j = 0, size2 = e1().getSize2(); j < size2; j++)
628 template <
typename M>
635 template <
typename M>
643 typedef typename M::SizeType SizeType;
646 SizeType size1 = e().getSize1();
647 SizeType size2 = e().getSize2();
649 for (SizeType i = 0; i < size1; i++)
650 for (SizeType j = 0; j < size2; j++)
657 template <
typename M>
665 typedef typename M::SizeType SizeType;
670 for (SizeType i = 0; i < size; i++)
677 template <
typename M>
686 template <
typename M>
696 typedef typename M::SizeType SizeType;
699 SizeType size1 = e().getSize1();
700 SizeType size2 = e().getSize2();
702 for (SizeType j = 0; j < size2; j++) {
705 for (SizeType i = 0; i < size1; i++)
716 template <
typename M>
726 typedef typename M::SizeType SizeType;
729 SizeType size1 = e().getSize1();
730 SizeType size2 = e().getSize2();
732 for (SizeType i = 0; i < size1; i++) {
733 for (SizeType j = 0; j < size2; j++) {
744 template <
typename M>
754 typedef typename M::SizeType SizeType;
757 SizeType size1 = e().getSize1();
758 SizeType size2 = e().getSize2();
760 for (SizeType i = 0; i < size1; i++) {
763 for (SizeType j = 0; j < size2; j++)
774 template <
typename V>
782 template <
typename V>
789 template <
typename E>
799 template <
typename V>
806 template <
typename E>
861 template <
typename M,
typename V>
870 template <
typename M,
typename V>
878 template <
typename E1,
typename E2>
885 res += e1()(i, j) * e2()(j);
891 template <
typename V,
typename M>
899 template <
typename E1,
typename E2>
906 res += e1()(j) * e2()(j, i);
912 template <
typename M1,
typename M2>
921 template <
typename M1,
typename M2>
929 template <
typename E1,
typename E2>
936 res += e1()(i, k) * e2()(k, j);
942 template <
typename Q1,
typename Q2>
950 template <
typename Q1,
typename Q2>
963 template <
typename Q1,
typename Q2,
typename T>
972 template <
typename Q1,
typename Q2,
typename T>
984 ComparisonType norm_inf_max(epsilon);
990 template <
typename Q>
997 template <
typename Q>
1005 return (e().getC1() + e().getC2() + e().getC3() + e().getC4());
1009 template <
typename Q>
1018 template <
typename Q>
1028 RealType t = e().getC1() * e().getC1() +
1029 e().getC2() * e().getC2() +
1030 e().getC3() * e().getC3() +
1031 e().getC4() * e().getC4();
1037 template <
typename Q>
1047 RealType t = e().getC1() * e().getC1() +
1048 e().getC2() * e().getC2() +
1049 e().getC3() * e().getC3() +
1050 e().getC4() * e().getC4();
1056 template <
typename Q>
1063 template <
typename Q>
1069 template <
typename E>
1075 template <
typename E>
1081 template <
typename E>
1087 template <
typename E>
1094 template <
typename Q>
1100 template <
typename E>
1106 template <
typename E>
1109 return -e().getC2();
1112 template <
typename E>
1115 return -e().getC3();
1118 template <
typename E>
1121 return -e().getC4();
1125 template <
typename T,
typename Q>
1133 template <
typename T,
typename Q>
1140 template <
typename E>
1143 return (t + e().getC1());
1146 template <
typename E>
1152 template <
typename E>
1158 template <
typename E>
1165 template <
typename T,
typename Q>
1172 template <
typename E>
1175 return (t - e().getC1());
1178 template <
typename E>
1181 return -e().getC2();
1184 template <
typename E>
1187 return -e().getC3();
1190 template <
typename E>
1193 return -e().getC4();
1197 template <
typename Q,
typename T>
1205 template <
typename Q,
typename T>
1212 template <
typename E>
1215 return (e().getC1() + t);
1218 template <
typename E>
1224 template <
typename E>
1230 template <
typename E>
1237 template <
typename Q,
typename T>
1244 template <
typename E>
1247 return (e().getC1() - t);
1250 template <
typename E>
1256 template <
typename E>
1262 template <
typename E>
1269 template <
typename Q,
typename T>
1276 template <
typename E>
1279 return (e().getC1() / n2);
1282 template <
typename E>
1285 return (-e().getC2() / n2);
1288 template <
typename E>
1291 return (-e().getC3() / n2);
1294 template <
typename E>
1297 return (-e().getC4() / n2);
1301 template <
typename Q1,
typename Q2>
1308 template <
typename Q1,
typename Q2>
1314 template <
typename E1,
typename E2>
1318 return (e1().getC1() * e2().getC1() - e1().getC2() * e2().getC2() - e1().getC3() * e2().getC3() - e1().getC4() * e2().getC4());
1321 template <
typename E1,
typename E2>
1325 return (e1().getC1() * e2().getC2() + e1().getC2() * e2().getC1() + e1().getC3() * e2().getC4() - e1().getC4() * e2().getC3());
1328 template <
typename E1,
typename E2>
1332 return (e1().getC1() * e2().getC3() - e1().getC2() * e2().getC4() + e1().getC3() * e2().getC1() + e1().getC4() * e2().getC2());
1335 template <
typename E1,
typename E2>
1339 return (e1().getC1() * e2().getC4() + e1().getC2() * e2().getC3() - e1().getC3() * e2().getC2() + e1().getC4() * e2().getC1());
1343 template <
typename Q1,
typename Q2,
typename T>
1351 template <
typename Q1,
typename Q2,
typename T>
1358 template <
typename E1,
typename E2>
1362 return ((e1().getC1() * e2().getC1() + e1().getC2() * e2().getC2() + e1().getC3() * e2().getC3() + e1().getC4() * e2().getC4()) / n2);
1365 template <
typename E1,
typename E2>
1369 return ((-e1().getC1() * e2().getC2() + e1().getC2() * e2().getC1() - e1().getC3() * e2().getC4() + e1().getC4() * e2().getC3()) / n2);
1372 template <
typename E1,
typename E2>
1376 return ((-e1().getC1() * e2().getC3() + e1().getC2() * e2().getC4() + e1().getC3() * e2().getC1() - e1().getC4() * e2().getC2()) / n2);
1379 template <
typename E1,
typename E2>
1383 return ((-e1().getC1() * e2().getC4() - e1().getC2() * e2().getC3() + e1().getC3() * e2().getC2() + e1().getC4() * e2().getC1()) / n2);
1387 template <
typename T1,
typename Q,
typename T2>
1396 template <
typename T1,
typename Q,
typename T2>
1404 template <
typename E>
1407 return (t * e().getC1() / n2);
1410 template <
typename E>
1413 return (t * -e().getC2() / n2);
1416 template <
typename E>
1419 return (t * -e().getC3() / n2);
1422 template <
typename E>
1425 return (t * -e().getC4() / n2);
1429 template <
typename Q,
typename V>
1438 template <
typename Q,
typename V>
1446 template <
typename E1,
typename E2>
1455 ValueType t1 = e1().getC1() * e1().getC1() + e1().getC2() * e1().getC2() - e1().getC3() * e1().getC3() - e1().getC4() * e1().getC4();
1456 ValueType t2 =
ValueType(2) * (e1().getC2() * e1().getC3() - e1().getC1() * e1().getC4());
1457 ValueType t3 =
ValueType(2) * (e1().getC2() * e1().getC4() + e1().getC1() * e1().getC3());
1459 return (t1 * e2()(0) + t2 * e2()(1) + t3 * e2()(2));
1464 ValueType t1 =
ValueType(2) * (e1().getC2() * e1().getC3() + e1().getC1() * e1().getC4());
1465 ValueType t2 = e1().getC1() * e1().getC1() - e1().getC2() * e1().getC2() + e1().getC3() * e1().getC3() - e1().getC4() * e1().getC4();
1466 ValueType t3 =
ValueType(2) * (e1().getC3() * e1().getC4() - e1().getC1() * e1().getC2());
1468 return (t1 * e2()(0) + t2 * e2()(1) + t3 * e2()(2));
1473 ValueType t1 =
ValueType(2) * (e1().getC2() * e1().getC4() - e1().getC1() * e1().getC3());
1474 ValueType t2 =
ValueType(2) * (e1().getC3() * e1().getC4() + e1().getC1() * e1().getC2());
1475 ValueType t3 = e1().getC1() * e1().getC1() - e1().getC2() * e1().getC2() - e1().getC3() * e1().getC3() + e1().getC4() * e1().getC4();
1477 return (t1 * e2()(0) + t2 * e2()(1) + t3 * e2()(2));
1486 template <
typename G1,
typename G2>
1495 template <
typename G1,
typename G2>
1514 for (
SizeType i = 0, size1 = e1().getSize1(); i < size1; i++)
1515 for (
SizeType j = 0, size2 = e1().getSize2(); j < size2; j++)
1516 for (
SizeType k = 0, size3 = e1().getSize3(); k < size3; k++)
1524 template <
typename G1,
typename G2,
typename T>
1534 template <
typename G1,
typename G2,
typename T>
1556 ComparisonType norm_inf_max(epsilon);
1558 for (
SizeType i = 0, size1 = e1().getSize1(); i < size1; i++)
1559 for (
SizeType j = 0, size2 = e1().getSize2(); j < size2; j++)
1560 for (
SizeType k = 0, size3 = e1().getSize3(); k < size3; k++)
1568 template <
typename M>
1575 template <
typename G>
1583 typedef typename G::SizeType SizeType;
1586 SizeType size1 = e().getSize1();
1587 SizeType size2 = e().getSize2();
1588 SizeType size3 = e().getSize3();
1590 for (SizeType i = 0; i < size1; i++)
1591 for (SizeType j = 0; j < size2; j++)
1592 for (SizeType k = 0; k < size3; k++)
1593 res += e()(i, j, k);
1601 #endif // CDPL_MATH_FUNCTIONAL_HPP
QuaternionVectorBinaryFunctor< Q, V >::SizeType SizeType
Definition: Functional.hpp:1443
RealType ResultType
Definition: Functional.hpp:683
QuaternionScalarRealUnaryFunctor< Q >::ValueType ValueType
Definition: Functional.hpp:1022
ScalarBinaryFunctor< T1, T2 >::ResultType ResultType
Definition: Functional.hpp:215
ScalarBinaryFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:241
CommonType< typename VectorInnerProduct< V1, V2 >::ResultType, T >::Type ResultType
Definition: Functional.hpp:296
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:752
static ResultType apply(const GridExpression< G1 > &e1, const GridExpression< G2 > &e2, Argument3Type epsilon)
Definition: Functional.hpp:1543
ValueType ResultType
Definition: Functional.hpp:918
Definition: Functional.hpp:565
Definition: TypeTraits.hpp:171
Definition: Functional.hpp:519
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:92
VectorScalarRealUnaryFunctor< V >::ResultType ResultType
Definition: Functional.hpp:474
GridBooleanBinaryFunctor< G1, G2 >::SizeType SizeType
Definition: Functional.hpp:1499
static ResultType applyC2(const QuaternionExpression< E > &e, Argument2Type)
Definition: Functional.hpp:1219
Definition: Functional.hpp:1135
#define CDPL_MATH_CHECK_SIZE_EQUALITY(size1, size2, e)
Definition: Check.hpp:62
CommonType< typename V1::SizeType, typename V2::SizeType >::Type SizeType
Definition: Functional.hpp:314
bool ResultType
Definition: Functional.hpp:1490
MatrixScalarRealUnaryFunctor< M >::RealType RealType
Definition: Functional.hpp:691
Scalar2QuaternionBinaryFunctor< Q, T >::Argument2Type Argument2Type
Definition: Functional.hpp:1209
std::common_type< T1, T2 >::type Type
Definition: CommonType.hpp:43
Definition: Functional.hpp:1303
M::ValueType ValueType
Definition: Functional.hpp:681
Scalar1QuaternionBinaryFunctor< T, Q >::Argument1Type Argument1Type
Definition: Functional.hpp:1169
Scalar2QuaternionBinaryFunctor< Q, T >::ResultType ResultType
Definition: Functional.hpp:1210
VectorScalarRealUnaryFunctor< V >::RealType RealType
Definition: Functional.hpp:473
CommonType< typename V1::ValueType, typename V2::ValueType >::Type ResultType
Definition: Functional.hpp:269
static ResultType apply(const VectorExpression< V1 > &e1, const VectorExpression< V2 > &e2)
Definition: Functional.hpp:278
Definition: Functional.hpp:1167
Scalar2QuaternionBinaryFunctor< Q, T >::ResultType ResultType
Definition: Functional.hpp:1274
VectorScalarRealUnaryFunctor< V >::ResultType ResultType
Definition: Functional.hpp:453
Definition: Functional.hpp:590
Definition: Functional.hpp:688
ScalarBinaryFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:214
static ResultType apply(const VectorExpression< V > &e)
Definition: Functional.hpp:476
Definition: Functional.hpp:1526
static ResultType apply(const MatrixExpression< M1 > &e1, const MatrixExpression< M2 > &e2)
Definition: Functional.hpp:571
QuaternionNorm2< E >::ResultType norm2(const QuaternionExpression< E > &e)
Definition: QuaternionExpression.hpp:804
Scalar3GridBooleanTernaryFunctor< G1, G2, T >::Argument3Type Argument3Type
Definition: Functional.hpp:1541
static ResultType applyC3(Argument1Type, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1185
CommonType< typename M1::SizeType, typename M2::SizeType >::Type SizeType
Definition: Functional.hpp:917
ScalarBinaryFunctor< T1, T2 >::ResultType ResultType
Definition: Functional.hpp:243
VectorBooleanBinaryFunctor< V1, V2 >::SizeType SizeType
Definition: Functional.hpp:322
ScalarBinaryFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:213
MatrixScalarUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:661
static ResultType applyC2(const QuaternionExpression< E > &e, Argument2Type)
Definition: Functional.hpp:1251
T1 Argument1Type
Definition: Functional.hpp:57
VectorScalarUnaryFunctor< V >::ResultType ResultType
Definition: Functional.hpp:423
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:663
CommonType< typename Q::ValueType, typename V::ValueType >::Type ValueType
Definition: Functional.hpp:1433
static ResultType apply(const VectorExpression< V > &e)
Definition: Functional.hpp:455
Definition: Functional.hpp:63
ScalarBinaryFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:227
Definition: Functional.hpp:1345
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:66
Definition: Functional.hpp:320
const T & Argument3Type
Definition: Functional.hpp:1529
Scalar13QuaternionTernaryFunctor< T1, Q, T2 >::Argument3Type Argument3Type
Definition: Functional.hpp:1401
static ResultType applyC2(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2)
Definition: Functional.hpp:1322
VectorScalarRealUnaryFunctor< V >::RealType RealType
Definition: Functional.hpp:452
Scalar13QuaternionTernaryFunctor< T1, Q, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:1400
Definition: Expression.hpp:54
Definition: Functional.hpp:174
ScalarUnaryFunctor< T >::ResultType ResultType
Definition: Functional.hpp:141
Scalar3VectorBooleanTernaryFunctor< V1, V2, T >::ResultType ResultType
Definition: Functional.hpp:355
ScalarUnaryFunctor< T >::ArgumentType ArgumentType
Definition: Functional.hpp:154
V::SizeType ResultType
Definition: Functional.hpp:523
Definition: Functional.hpp:1440
static ResultType applyC3(Argument1Type, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1153
Definition: Functional.hpp:556
Definition: Functional.hpp:1488
bool ResultType
Definition: Functional.hpp:967
Definition: Functional.hpp:311
const T2 & Argument2Type
Definition: Functional.hpp:58
const T1 & Argument1Type
Definition: Functional.hpp:1392
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:65
V::SizeType SizeType
Definition: Functional.hpp:779
static ResultType applyC4(Argument1Type, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1191
Definition: Functional.hpp:528
Scalar2QuaternionBinaryFunctor< Q, T >::ResultType ResultType
Definition: Functional.hpp:1242
VectorScalarBinaryFunctor< V1, V2 >::ResultType ResultType
Definition: Functional.hpp:386
QuaternionScalarRealUnaryFunctor< Q >::RealType RealType
Definition: Functional.hpp:1023
Scalar1QuaternionBinaryFunctor< T, Q >::ResultType ResultType
Definition: Functional.hpp:1138
ScalarUnaryFunctor< T >::ArgumentType ArgumentType
Definition: Functional.hpp:191
Definition: Functional.hpp:992
Definition: Functional.hpp:341
Definition: Functional.hpp:1431
Scalar3MatrixBooleanTernaryFunctor< M1, M2, T >::ValueType ValueType
Definition: Functional.hpp:603
Definition: Functional.hpp:630
Scalar3GridBooleanTernaryFunctor< G1, G2, T >::SizeType SizeType
Definition: Functional.hpp:1538
Scalar3QuaternionBooleanTernaryFunctor< Q1, Q2, T >::Argument3Type Argument3Type
Definition: Functional.hpp:978
GridUnaryTraits< E, ScalarReal< typename E::ValueType > >::ResultType real(const GridExpression< E > &e)
Definition: GridExpression.hpp:378
const T & ArgumentType
Definition: Functional.hpp:131
Scalar2QuaternionBinaryFunctor< Q, T >::Argument2Type Argument2Type
Definition: Functional.hpp:1241
Scalar3QuaternionBooleanTernaryFunctor< Q1, Q2, T >::ResultType ResultType
Definition: Functional.hpp:977
MatrixBooleanBinaryFunctor< M1, M2 >::SizeType SizeType
Definition: Functional.hpp:567
bool ResultType
Definition: Functional.hpp:946
static ResultType applyC3(const QuaternionExpression< E > &e, Argument2Type)
Definition: Functional.hpp:1257
M::ValueType ResultType
Definition: Functional.hpp:1572
static ResultType applyC4(const QuaternionExpression< E > &e, Argument2Type n2)
Definition: Functional.hpp:1295
static ResultType apply(const QuaternionExpression< Q > &e)
Definition: Functional.hpp:1026
const T1 & Argument1Type
Definition: Functional.hpp:204
MatrixVectorBinaryFunctor< M, V >::ResultType ResultType
Definition: Functional.hpp:876
Definition: Functional.hpp:872
Definition: Functional.hpp:239
static ResultType applyC3(Argument1Type t, const QuaternionExpression< E > &e, Argument3Type n2)
Definition: Functional.hpp:1417
VectorScalarIndexUnaryFunctor< V >::RealType RealType
Definition: Functional.hpp:531
Definition: Functional.hpp:151
static ResultType apply(const GridExpression< G > &e)
Definition: Functional.hpp:1581
VectorBooleanBinaryFunctor< V1, V2 >::ValueType ValueType
Definition: Functional.hpp:323
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:91
Definition: Functional.hpp:1199
CommonType< typename G1::ValueType, typename G2::ValueType >::Type ValueType
Definition: Functional.hpp:1531
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:78
Definition: Functional.hpp:974
static ResultType apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:217
static ResultType apply(const QuaternionExpression< Q > &e)
Definition: Functional.hpp:1003
Definition: Functional.hpp:211
CommonType< typename M1::SizeType, typename M2::SizeType >::Type SizeType
Definition: Functional.hpp:594
VectorScalarRealUnaryFunctor< V >::ValueType ValueType
Definition: Functional.hpp:451
Definition: Functional.hpp:188
Definition: Functional.hpp:421
static ResultType applyC1(const QuaternionExpression< E > &e, Argument2Type t)
Definition: Functional.hpp:1213
Definition: Expression.hpp:76
bool ResultType
Definition: Functional.hpp:313
#define CDPL_MATH_CHECK(expr, msg, e)
Definition: Check.hpp:36
static ResultType applyC4(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1119
const T & Argument3Type
Definition: Functional.hpp:593
Definition: Functional.hpp:1127
ScalarUnaryFunctor< T >::ArgumentType ArgumentType
Definition: Functional.hpp:177
static ResultType applyC4(Argument1Type, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1159
CommonType< typename M1::ValueType, typename M2::ValueType >::Type ValueType
Definition: Functional.hpp:560
bool ResultType
Definition: Functional.hpp:1528
CommonType< typename M1::ValueType, typename M2::ValueType >::Type ValueType
Definition: Functional.hpp:595
Definition: CommonType.hpp:41
Definition: Expression.hpp:98
MatrixScalarUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:639
static ResultType applyC1(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1101
Definition: Functional.hpp:253
Definition: Functional.hpp:952
static ResultType applyC1(const QuaternionExpression< E > &e, Argument2Type t)
Definition: Functional.hpp:1245
static ResultType applyC1(Argument1Type t, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1141
Scalar3QuaternionTernaryFunctor< Q1, Q2, T >::ResultType ResultType
Definition: Functional.hpp:1356
static ResultType apply(const MatrixExpression< M1 > &e1, const MatrixExpression< M2 > &e2, Argument3Type epsilon)
Definition: Functional.hpp:607
static ResultType applyC3(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1082
static ResultType apply(const VectorExpression< V > &e)
Definition: Functional.hpp:534
static ResultType apply(ArgumentType v)
Definition: Functional.hpp:180
static ResultType apply(const VectorExpression< V > &e)
Definition: Functional.hpp:425
TypeTraits< ValueType >::RealType RealType
Definition: Functional.hpp:682
VectorScalarRealUnaryFunctor< V >::RealType RealType
Definition: Functional.hpp:497
MatrixScalarRealUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:692
static void apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:107
static ResultType apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:245
Definition: Functional.hpp:202
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:117
QuaternionBooleanBinaryFunctor< Q1, Q2 >::ResultType ResultType
Definition: Functional.hpp:955
QuaternionBinaryFunctor< Q1, Q2 >::ResultType ResultType
Definition: Functional.hpp:1312
MatrixVectorBinaryFunctor< M, V >::ValueType ValueType
Definition: Functional.hpp:895
VectorScalarUnaryFunctor< V >::SizeType SizeType
Definition: Functional.hpp:804
Definition: Functional.hpp:893
Definition: Functional.hpp:718
ScalarBinaryFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:242
Definition: Functional.hpp:965
Definition: Functional.hpp:377
Definition: Functional.hpp:494
ValueType ResultType
Definition: Functional.hpp:867
static ResultType apply(ArgumentType v)
Definition: Functional.hpp:157
QuaternionVectorBinaryFunctor< Q, V >::ResultType ResultType
Definition: Functional.hpp:1444
Definition: Functional.hpp:115
static void apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:94
bool ResultType
Definition: Functional.hpp:592
static ResultType apply(const VectorExpression< V > &e)
Definition: Functional.hpp:500
static ResultType applyC4(Argument1Type t, const QuaternionExpression< E > &e, Argument3Type n2)
Definition: Functional.hpp:1423
Definition: Functional.hpp:1058
const T & Argument3Type
Definition: Functional.hpp:1348
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:118
static ResultType applyC3(const QuaternionExpression< E > &e, Argument2Type)
Definition: Functional.hpp:1225
ScalarUnaryFunctor< T >::ResultType ResultType
Definition: Functional.hpp:155
RealType ResultType
Definition: Functional.hpp:1015
Scalar3GridBooleanTernaryFunctor< G1, G2, T >::ValueType ValueType
Definition: Functional.hpp:1539
TypeTraits< T >::RealType ResultType
Definition: Functional.hpp:169
const T & Argument3Type
Definition: Functional.hpp:968
ScalarBinaryFunctor< T1, T2 >::ResultType ResultType
Definition: Functional.hpp:257
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:694
Scalar3VectorBooleanTernaryFunctor< V1, V2, T >::Argument3Type Argument3Type
Definition: Functional.hpp:356
ScalarUnaryFunctor< T >::ResultType ResultType
Definition: Functional.hpp:178
ScalarUnaryFunctor< T >::ValueType ValueType
Definition: Functional.hpp:153
static ResultType apply(const GridExpression< G1 > &e1, const GridExpression< G2 > &e2)
Definition: Functional.hpp:1503
static void apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:68
Definition: Functional.hpp:440
QuaternionScalarRealUnaryFunctor< Q >::RealType RealType
Definition: Functional.hpp:1042
Definition: Functional.hpp:128
static ResultType applyC2(Argument1Type t, const QuaternionExpression< E > &e, Argument3Type n2)
Definition: Functional.hpp:1411
Definition of type traits.
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:79
CommonType< typename Q1::ValueType, typename Q2::ValueType >::Type ResultType
Definition: Functional.hpp:1305
static ResultType apply(ArgumentType v)
Definition: Functional.hpp:194
Definition: Functional.hpp:1096
static ResultType apply(const VectorExpression< E > &e, SizeType i, SizeType j)
Definition: Functional.hpp:807
Definition: Functional.hpp:225
VectorScalarIndexUnaryFunctor< V >::ResultType ResultType
Definition: Functional.hpp:532
static ResultType apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:231
static ResultType apply(const QuaternionExpression< Q > &e)
Definition: Functional.hpp:1045
Definition: Functional.hpp:944
V::ValueType ResultType
Definition: Functional.hpp:778
CommonType< typename G1::ValueType, typename G2::ValueType >::Type ValueType
Definition: Functional.hpp:1492
V::SizeType SizeType
Definition: Functional.hpp:1434
static ResultType applyC1(const QuaternionExpression< E > &e, Argument2Type n2)
Definition: Functional.hpp:1277
static ResultType apply(const VectorExpression< E1 > &e1, const VectorExpression< E2 > &e2, SizeType i)
Definition: Functional.hpp:389
static ResultType applyC4(const QuaternionExpression< E > &e, Argument2Type)
Definition: Functional.hpp:1231
V::SizeType SizeType
Definition: Functional.hpp:416
GridBooleanBinaryFunctor< G1, G2 >::ResultType ResultType
Definition: Functional.hpp:1501
Definition: Functional.hpp:914
bool ResultType
Definition: Functional.hpp:558
MatrixScalarRealUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:722
static ResultType applyC1(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1070
V::ValueType ValueType
Definition: Functional.hpp:442
Scalar3MatrixBooleanTernaryFunctor< M1, M2, T >::SizeType SizeType
Definition: Functional.hpp:602
VectorScalarRealUnaryFunctor< V >::ValueType ValueType
Definition: Functional.hpp:496
static ResultType apply(const VectorExpression< V1 > &e1, const VectorExpression< V2 > &e2, const T &sd, bool clamp)
Definition: Functional.hpp:298
VectorScalarUnaryFunctor< V >::ResultType ResultType
Definition: Functional.hpp:803
Definition: Functional.hpp:294
GridUnaryTraits< E, ScalarConjugation< typename E::ValueType > >::ResultType conj(const GridExpression< E > &e)
Definition: GridExpression.hpp:360
Definition: Functional.hpp:449
V::ValueType ValueType
Definition: Functional.hpp:521
Definition: Functional.hpp:165
Definition: Functional.hpp:1011
Definition: Functional.hpp:1039
CommonType< typename CommonType< typename Q1::ValueType, typename Q2::ValueType >::Type, T >::Type ResultType
Definition: Functional.hpp:1347
Definition: Functional.hpp:801
static ResultType applyC1(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2)
Definition: Functional.hpp:1315
Definition: Functional.hpp:274
Definition: Functional.hpp:1577
Scalar3MatrixBooleanTernaryFunctor< M1, M2, T >::Argument3Type Argument3Type
Definition: Functional.hpp:605
CommonType< typename CommonType< T1, typename Q::ValueType >::Type, T2 >::Type ResultType
Definition: Functional.hpp:1391
static ResultType applyC4(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2)
Definition: Functional.hpp:1336
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
ScalarBinaryFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:256
Definition: Functional.hpp:1020
Definition of exception classes.
ValueType RealType
Definition: Functional.hpp:1014
MatrixBooleanBinaryFunctor< M1, M2 >::ResultType ResultType
Definition: Functional.hpp:569
CommonType< typename G1::SizeType, typename G2::SizeType >::Type SizeType
Definition: Functional.hpp:1491
CommonType< typename V1::ValueType, typename V2::ValueType >::Type ValueType
Definition: Functional.hpp:315
const T2 & Argument3Type
Definition: Functional.hpp:1393
Q::ValueType ResultType
Definition: Functional.hpp:1060
ScalarBinaryFunctor< T1, T2 >::ResultType ResultType
Definition: Functional.hpp:229
ScalarUnaryFunctor< T >::ResultType ResultType
Definition: Functional.hpp:192
GridScalarUnaryFunctor< G >::ResultType ResultType
Definition: Functional.hpp:1579
MatrixScalarRealUnaryFunctor< M >::RealType RealType
Definition: Functional.hpp:721
V::ValueType ResultType
Definition: Functional.hpp:415
static ResultType applyC3(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2)
Definition: Functional.hpp:1329
Q::ValueType ResultType
Definition: Functional.hpp:994
M::ValueType ResultType
Definition: Functional.hpp:632
Definition: Expression.hpp:120
static ResultType apply(const MatrixExpression< E1 > &e1, const MatrixExpression< E2 > &e2, SizeType i, SizeType j)
Definition: Functional.hpp:930
TypeTraits< ValueType >::RealType RealType
Definition: Functional.hpp:443
ValueType ResultType
Definition: Functional.hpp:1435
Definition: Functional.hpp:1065
MatrixVectorBinaryFunctor< M, V >::SizeType SizeType
Definition: Functional.hpp:896
VectorScalarUnaryFunctor< V >::ResultType ResultType
Definition: Functional.hpp:786
VectorScalarRealUnaryFunctor< V >::ValueType ValueType
Definition: Functional.hpp:472
Definition: Functional.hpp:351
VectorScalarUnaryFunctor< V >::SizeType SizeType
Definition: Functional.hpp:787
MatrixScalarRealUnaryFunctor< M >::ValueType ValueType
Definition: Functional.hpp:690
static ResultType applyC2(const QuaternionExpression< E > &e, Argument2Type n2)
Definition: Functional.hpp:1283
ScalarUnaryFunctor< T >::ValueType ValueType
Definition: Functional.hpp:190
static ResultType apply(const VectorExpression< E1 > &e1, const MatrixExpression< E2 > &e2, SizeType i)
Definition: Functional.hpp:900
The namespace of the Chemical Data Processing Library.
T ValueType
Definition: Functional.hpp:130
Definition: Functional.hpp:1207
static ResultType apply(const VectorExpression< V1 > &e1, const VectorExpression< V2 > &e2)
Definition: Functional.hpp:326
CommonType< T1, T2 >::Type ResultType
Definition: Functional.hpp:206
MatrixScalarRealUnaryFunctor< M >::ValueType ValueType
Definition: Functional.hpp:748
static ResultType apply(const QuaternionExpression< Q1 > &e1, const QuaternionExpression< Q2 > &e2)
Definition: Functional.hpp:957
static void apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:81
ScalarBinaryFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:255
Scalar1QuaternionBinaryFunctor< T, Q >::Argument1Type Argument1Type
Definition: Functional.hpp:1137
Definition: Functional.hpp:89
const T & ArgumentType
Definition: Functional.hpp:168
MatrixNormInfinity< E >::ResultType normInf(const MatrixExpression< E > &e)
Definition: MatrixExpression.hpp:917
static ResultType apply(const QuaternionExpression< E1 > &e1, const VectorExpression< E2 > &e2, SizeType i)
Definition: Functional.hpp:1447
CommonType< typename Q1::ValueType, typename Q2::ValueType >::Type ValueType
Definition: Functional.hpp:947
Definition: Functional.hpp:137
static ResultType apply(const VectorExpression< E > &e, SizeType i, SizeType j)
Definition: Functional.hpp:790
CommonType< T, typename Q::ValueType >::Type ResultType
Definition: Functional.hpp:1129
Definition: Functional.hpp:1536
QuaternionNorm< E >::ResultType norm(const QuaternionExpression< E > &e)
Definition: QuaternionExpression.hpp:797
const T & Argument2Type
Definition: Functional.hpp:1202
static ResultType applyC1(Argument1Type t, const QuaternionExpression< E > &e, Argument3Type n2)
Definition: Functional.hpp:1405
MatrixVectorBinaryFunctor< M1, M2 >::ValueType ValueType
Definition: Functional.hpp:925
const T & Argument1Type
Definition: Functional.hpp:1130
CommonType< typename M::SizeType, typename V::SizeType >::Type SizeType
Definition: Functional.hpp:866
RealType ResultType
Definition: Functional.hpp:444
QuaternionScalarRealUnaryFunctor< Q >::ResultType ResultType
Definition: Functional.hpp:1043
Definition: Functional.hpp:1389
QuaternionScalarRealUnaryFunctor< Q >::ValueType ValueType
Definition: Functional.hpp:1041
static ResultType applyC2(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1107
VectorBooleanBinaryFunctor< V1, V2 >::ResultType ResultType
Definition: Functional.hpp:324
ScalarUnaryFunctor< T >::ValueType ValueType
Definition: Functional.hpp:176
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:724
static ResultType applyC3(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2, Argument3Type n2)
Definition: Functional.hpp:1373
VectorScalarRealUnaryFunctor< V >::ResultType ResultType
Definition: Functional.hpp:498
Definition: Functional.hpp:76
Definition: Functional.hpp:1497
Definition: Functional.hpp:659
CommonType< typename V1::ValueType, typename V2::ValueType >::Type ValueType
Definition: Functional.hpp:346
MatrixScalarRealUnaryFunctor< M >::RealType RealType
Definition: Functional.hpp:749
Definition: Functional.hpp:863
ScalarUnaryFunctor< T >::ValueType ValueType
Definition: Functional.hpp:139
Definition: Functional.hpp:470
Definition: Functional.hpp:102
ScalarBinaryFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:228
Definition: Functional.hpp:1570
Scalar3VectorBooleanTernaryFunctor< V1, V2, T >::ValueType ValueType
Definition: Functional.hpp:354
MatrixScalarRealUnaryFunctor< M >::ValueType ValueType
Definition: Functional.hpp:720
VectorScalarBinaryFunctor< V1, V2 >::ResultType ResultType
Definition: Functional.hpp:276
TypeTraits< ValueType >::RealType RealType
Definition: Functional.hpp:522
Definition: Functional.hpp:999
CommonType< typename Q1::ValueType, typename Q2::ValueType >::Type ValueType
Definition: Functional.hpp:969
CommonType< typename V1::SizeType, typename V2::SizeType >::Type SizeType
Definition: Functional.hpp:345
GridUnaryTraits< E, ScalarImaginary< typename E::ValueType > >::ResultType imag(const GridExpression< E > &e)
Definition: GridExpression.hpp:387
static ResultType applyC2(Argument1Type, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1179
Definition: Functional.hpp:267
MatrixVectorBinaryFunctor< M1, M2 >::ResultType ResultType
Definition: Functional.hpp:927
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument1Type Argument1Type
Definition: Functional.hpp:104
Scalar3VectorBooleanTernaryFunctor< V1, V2, T >::SizeType SizeType
Definition: Functional.hpp:353
static ResultType applyC4(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1088
QuaternionScalarRealUnaryFunctor< Q >::ResultType ResultType
Definition: Functional.hpp:1024
Definition: Functional.hpp:55
T ValueType
Definition: Functional.hpp:167
Scalar3QuaternionTernaryFunctor< Q1, Q2, T >::Argument3Type Argument3Type
Definition: Functional.hpp:1355
QuaternionBooleanBinaryFunctor< Q1, Q2 >::ValueType ValueType
Definition: Functional.hpp:954
Scalar2QuaternionBinaryFunctor< Q, T >::Argument2Type Argument2Type
Definition: Functional.hpp:1273
QuaternionVectorBinaryFunctor< Q, V >::ValueType ValueType
Definition: Functional.hpp:1442
MatrixVectorBinaryFunctor< M, V >::ValueType ValueType
Definition: Functional.hpp:874
CommonType< typename V1::ValueType, typename V2::ValueType >::Type ResultType
Definition: Functional.hpp:379
static ResultType applyC1(Argument1Type t, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1173
Definition: Functional.hpp:600
static ResultType applyC3(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1113
const T & Argument3Type
Definition: Functional.hpp:344
static ResultType applyC4(const QuaternionExpression< E > &e, Argument2Type)
Definition: Functional.hpp:1263
Definition: Functional.hpp:1353
Definition of various preprocessor macros for error checking.
bool ResultType
Definition: Functional.hpp:343
static ResultType applyC2(const QuaternionExpression< E > &e)
Definition: Functional.hpp:1076
static ResultType applyC4(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2, Argument3Type n2)
Definition: Functional.hpp:1380
static void apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:120
Scalar3QuaternionBooleanTernaryFunctor< Q1, Q2, T >::ValueType ValueType
Definition: Functional.hpp:976
static ResultType apply(const VectorExpression< V1 > &e1, const VectorExpression< V2 > &e2, Argument3Type epsilon)
Definition: Functional.hpp:358
Definition: Functional.hpp:784
MatrixVectorBinaryFunctor< M, V >::SizeType SizeType
Definition: Functional.hpp:875
CommonType< typename M::ValueType, typename V::ValueType >::Type ValueType
Definition: Functional.hpp:865
Scalar13QuaternionTernaryFunctor< T1, Q, T2 >::ResultType ResultType
Definition: Functional.hpp:1402
MatrixVectorBinaryFunctor< M1, M2 >::SizeType SizeType
Definition: Functional.hpp:926
ScalarBinaryAssignmentFunctor< T1, T2 >::Argument2Type Argument2Type
Definition: Functional.hpp:105
Scalar1QuaternionBinaryFunctor< T, Q >::ResultType ResultType
Definition: Functional.hpp:1170
Scalar3MatrixBooleanTernaryFunctor< M1, M2, T >::ResultType ResultType
Definition: Functional.hpp:604
Definition: Functional.hpp:1398
CommonType< typename Q::ValueType, T >::Type ResultType
Definition: Functional.hpp:1201
static ResultType applyC2(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2, Argument3Type n2)
Definition: Functional.hpp:1366
QuaternionUnaryFunctor< Q >::ResultType ResultType
Definition: Functional.hpp:1067
Definition: Functional.hpp:1239
const T2 & Argument2Type
Definition: Functional.hpp:205
QuaternionUnaryFunctor< Q >::ResultType ResultType
Definition: Functional.hpp:1098
ScalarUnaryFunctor< T >::ArgumentType ArgumentType
Definition: Functional.hpp:140
static ResultType apply(const MatrixExpression< E1 > &e1, const VectorExpression< E2 > &e2, SizeType i)
Definition: Functional.hpp:879
QuaternionScalarUnaryFunctor< Q >::ResultType ResultType
Definition: Functional.hpp:1001
Definition: Functional.hpp:1271
static ResultType apply(const MatrixExpression< M > &e)
Definition: Functional.hpp:641
Definition: Functional.hpp:776
MatrixBooleanBinaryFunctor< M1, M2 >::ValueType ValueType
Definition: Functional.hpp:568
Definition: Functional.hpp:637
Scalar3GridBooleanTernaryFunctor< G1, G2, T >::ResultType ResultType
Definition: Functional.hpp:1540
Definition: Functional.hpp:746
CommonType< typename M1::ValueType, typename M2::ValueType >::Type ValueType
Definition: Functional.hpp:916
static ResultType applyC3(const QuaternionExpression< E > &e, Argument2Type n2)
Definition: Functional.hpp:1289
GridBooleanBinaryFunctor< G1, G2 >::ValueType ValueType
Definition: Functional.hpp:1500
Definition: Functional.hpp:1310
MatrixVectorBinaryFunctor< M, V >::ResultType ResultType
Definition: Functional.hpp:897
Definition: Functional.hpp:413
Definition: Functional.hpp:384
Definition: Functional.hpp:923
ValueType ResultType
Definition: Functional.hpp:132
CommonType< typename G1::SizeType, typename G2::SizeType >::Type SizeType
Definition: Functional.hpp:1530
CommonType< typename M1::SizeType, typename M2::SizeType >::Type SizeType
Definition: Functional.hpp:559
static ResultType apply(ArgumentType v)
Definition: Functional.hpp:143
static ResultType apply(Argument1Type t1, Argument2Type t2)
Definition: Functional.hpp:259
Q::ValueType ValueType
Definition: Functional.hpp:1013
VectorScalarIndexUnaryFunctor< V >::ValueType ValueType
Definition: Functional.hpp:530
MatrixScalarRealUnaryFunctor< M >::ResultType ResultType
Definition: Functional.hpp:750
static ResultType apply(const QuaternionExpression< Q1 > &e1, const QuaternionExpression< Q2 > &e2, Argument3Type epsilon)
Definition: Functional.hpp:980
Definition: Functional.hpp:679
static ResultType applyC1(const QuaternionExpression< E1 > &e1, const QuaternionExpression< E2 > &e2, Argument3Type n2)
Definition: Functional.hpp:1359
static ResultType applyC2(Argument1Type, const QuaternionExpression< E > &e)
Definition: Functional.hpp:1147