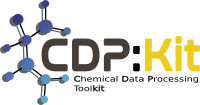 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_FONT_HPP
30 #define CDPL_VIS_FONT_HPP
73 Font(
const std::string& family,
double size = 12.0,
bool bold =
false,
bool italic =
false);
204 #endif // CDPL_VIS_FONT_HPP
void setFamily(const std::string &family)
Sets the font family name.
bool isUnderlined() const
Returns the flag specifying whether text is rendered underlined.
void setItalic(bool flag)
Sets or clears the flag specifying whether text should be rendered italicized.
Font()
Constructs a font object with an unspecified family name and a font size of 12.0.
bool isStrikedOut() const
Returns the flag specifying whether text is rendered striked-out.
Specifies a font for drawing text.
Definition: Font.hpp:54
bool isBold() const
Returns the flag specifying whether text is rendered bold.
bool isOverlined() const
Returns the flag specifying whether text is rendered overlined.
bool hasFixedPitch() const
Returns the fixed pitch flag.
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Font(const std::string &family, double size=12.0, bool bold=false, bool italic=false)
Constructs a font object with the specified font family name and font size.
void setBold(bool flag)
Sets or clears the flag specifying whether text should be rendered bold.
void setSize(double size)
Sets the font size.
The namespace of the Chemical Data Processing Library.
bool isItalic() const
Returns the flag specifying whether text is rendered italicized.
const std::string & getFamily() const
Returns the font family name.
void setUnderlined(bool flag)
Sets or clears the flag specifying whether text should be rendered underlined.
void setOverlined(bool flag)
Sets or clears the flag specifying whether text should be rendered overlined.
bool operator==(const Font &font) const
Equality comparison operator.
Definition of the preprocessor macro CDPL_VIS_API.
void setStrikedOut(bool flag)
Sets or clears the flag specifying whether text should be rendered striked-out.
double getSize() const
Returns the font size.
void setFixedPitch(bool flag)
Sets or clears the fixed pitch flag.
bool operator!=(const Font &font) const
Inequality comparison operator.